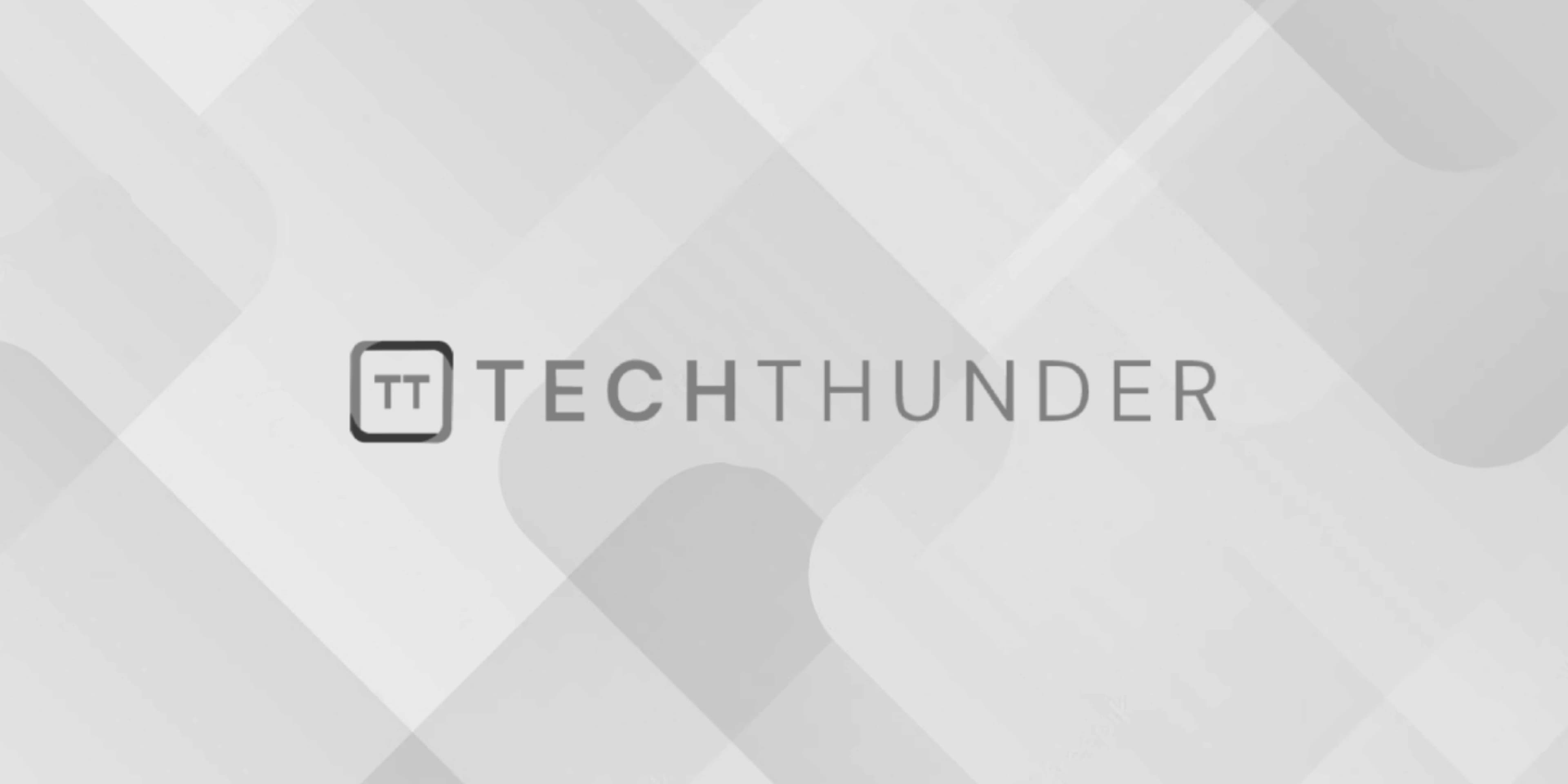
PHP Functions
In PHP, a function is a block of code that performs a specific task or set of tasks. Functions are used to organize code into reusable and modular units, making it easier to manage and maintain large PHP applications. Functions help improve code readability, promote code reusability, and allow developers to break down complex tasks into smaller, more manageable parts.
Creating a PHP Function:
To define a function in PHP, you use the function
keyword followed by the function name, a set of parentheses, and a pair of curly braces enclosing the function’s code block. Parameters (if any) can be defined within the parentheses, and the function can optionally return a value using the return
statement.
Here’s the syntax for creating a PHP function:
function functionName(parameter1, parameter2, ...) {
// Function code block
// ...
return result; // Optional return statement
}
Example of a Simple PHP Function:
function greet() {
echo "Hello, World!";
}
// Call the function
greet(); // Output: Hello, World!
Example of a PHP Function with Parameters and Return Value:
function add($num1, $num2) {
$sum = $num1 + $num2;
return $sum;
}
// Call the function and store the result in a variable
$result = add(5, 10);
echo $result; // Output: 15
Function Parameters:
You can pass parameters to a function to provide input values that the function will work with. Parameters act as placeholders for values that will be passed into the function when it is called. PHP functions can have zero or more parameters, and you can specify their data types (starting from PHP 7.0) for added type safety.
Function Return Values:
Functions in PHP can optionally return a value using the return
statement. If a function does not explicitly return a value, it returns null
by default. You can use the returned value for further processing or output.
Built-in Functions:
PHP comes with a large set of built-in functions that perform various tasks, such as string manipulation, array operations, mathematical calculations, date/time handling, file handling, and more. These functions are available for use in any PHP script without the need for additional setup or installation.
For a comprehensive list of built-in PHP functions and their descriptions, refer to the official PHP documentation: https://www.php.net/manual/en/function.array.php
Overall, functions are an essential aspect of PHP programming, allowing developers to create efficient, modular, and maintainable code. By using functions effectively, you can write more organized and structured PHP applications.