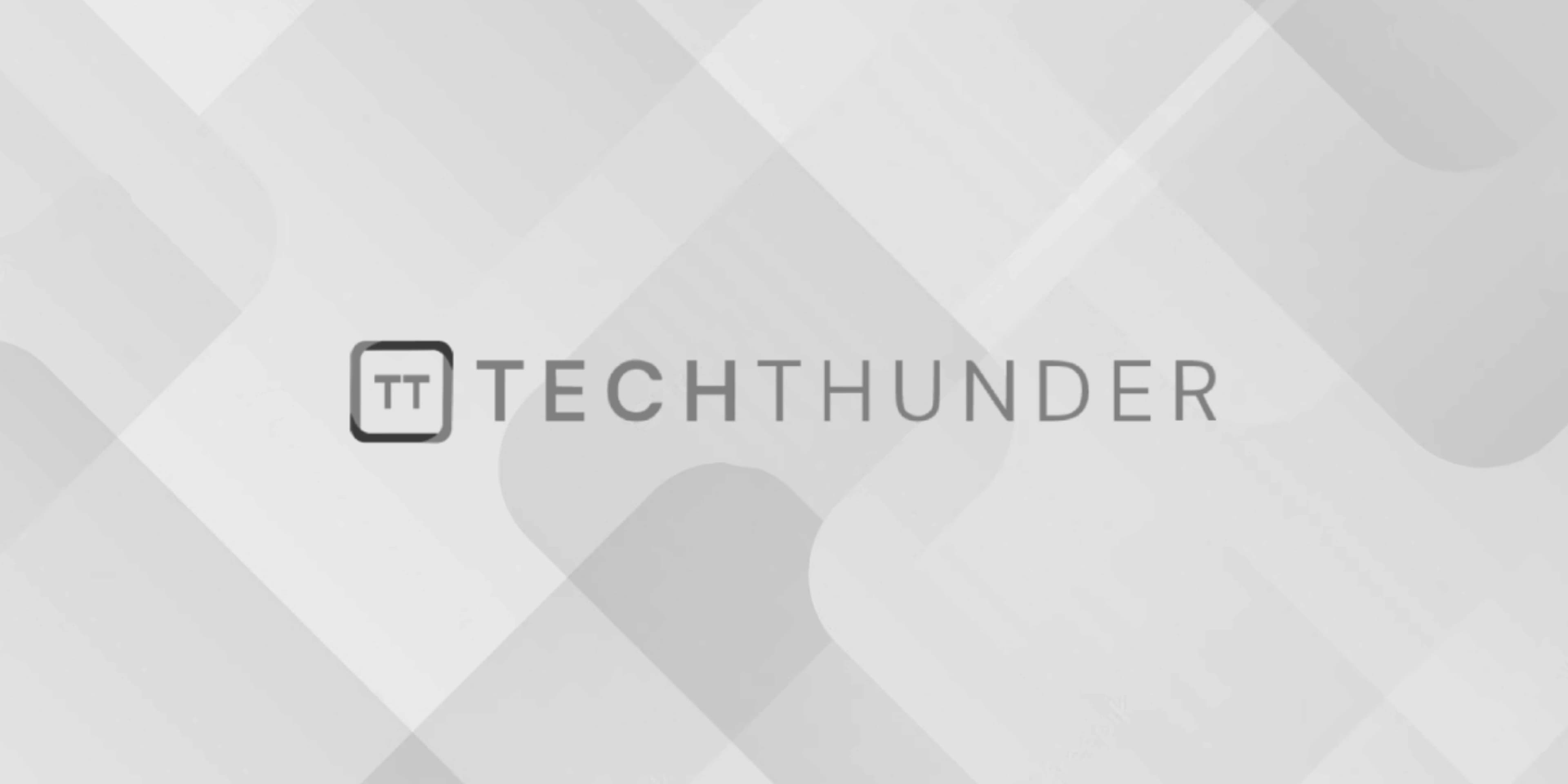
PHP Static Variables
In PHP, a static variable is a variable that belongs to a function or method and retains its value between invocations of that function or method. Unlike regular variables, static variables are not destroyed when a function or method finishes executing. Instead, they preserve their value, allowing you to maintain state information across multiple calls.
To define a static variable within a function or method, you use the static
keyword before the variable declaration. Here’s the syntax:
function functionName() {
static $variableName = initialValue;
// Function code...
}
Let’s look at an example to better understand the behavior of static variables:
function countCalls() {
static $count = 0;
$count++;
echo "This function has been called $count times.<br>";
}
countCalls(); // Output: This function has been called 1 times.
countCalls(); // Output: This function has been called 2 times.
countCalls(); // Output: This function has been called 3 times.
In this example, the countCalls()
function contains a static variable called $count
. Each time the function is called, the value of $count
is incremented by one and printed. However, since $count
is declared as static, it retains its value between calls. As a result, the count is correctly preserved across multiple invocations of the function.
Static variables are often used when you need to maintain some state information within a function or method. They can be useful for implementing counters, caching results, or tracking data that should persist across function calls but doesn’t need to be stored globally.
Keep in mind the following points about PHP static variables:
- Static variables are only accessible within the function or method where they are defined. They are not visible outside the function’s scope.
- If you want to initialize a static variable with the result of an expression or a function call, it’s better to use the
static
keyword outside the variable definition to ensure the initialization happens only once, like this:
function expensiveOperation() {
static $result;
if (!isset($result)) {
$result = // perform the expensive operation
}
return $result;
}
- The value of a static variable is not shared across different functions or methods. Each function/method has its own copy of the static variable.
Overall, static variables in PHP provide a convenient way to retain data across function calls without resorting to global variables, improving code encapsulation and maintainability.