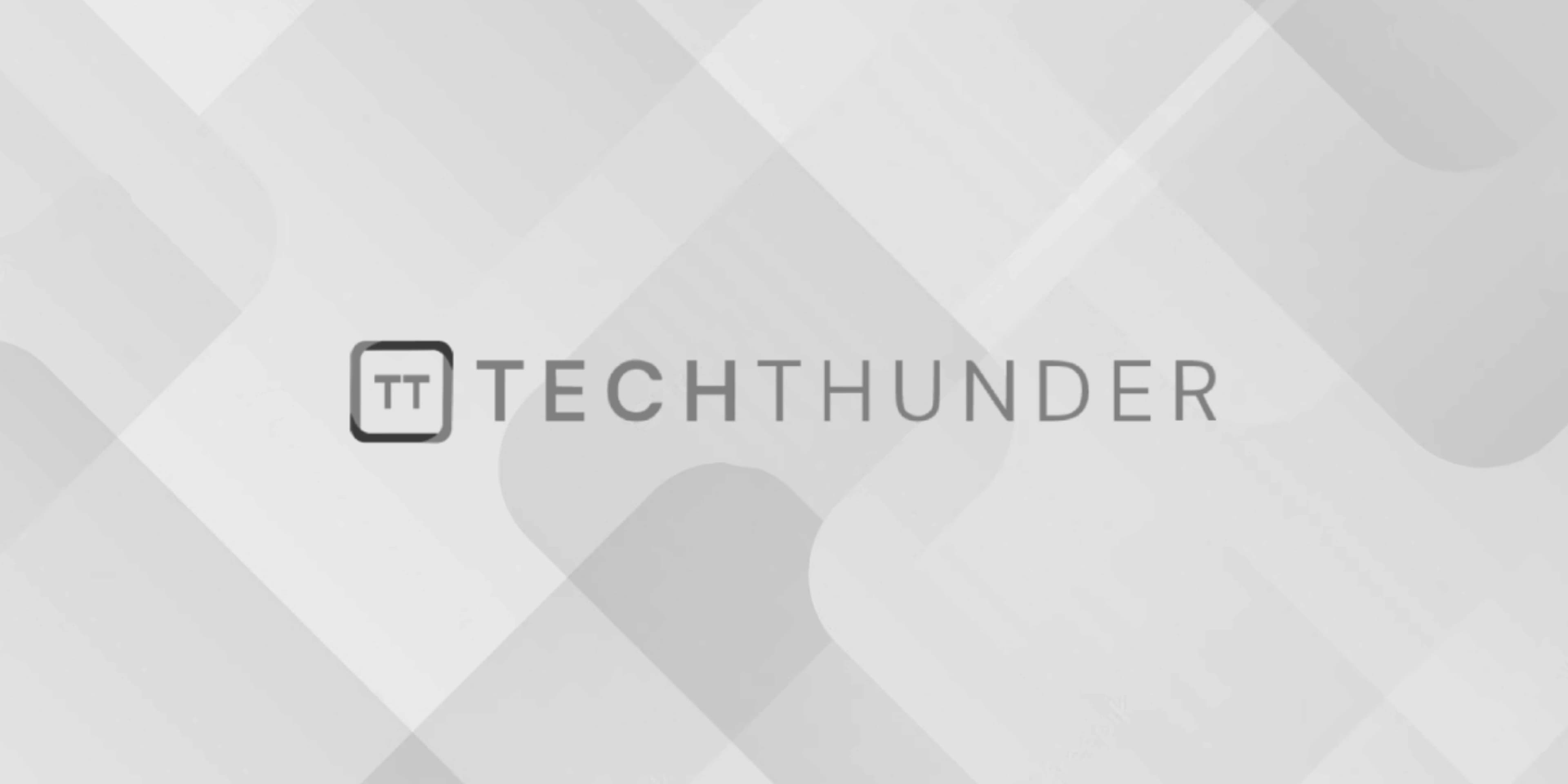
PHP Session
In PHP, sessions are a way to store and persist data across multiple HTTP requests for the same user. A session allows you to store user-specific information on the server and associate it with a unique session identifier (usually stored as a cookie on the client-side). This way, the server can identify the user on subsequent requests and retrieve the stored data.
Here’s how you can work with sessions in PHP:
- Starting a Session:
To start a session, you need to call thesession_start()
function at the beginning of your PHP script. This function initializes the session mechanism and either creates a new session or resumes an existing one based on the session identifier sent by the client.
<?php
session_start();
?>
- Storing Data in a Session:
Once the session is started, you can store data in the session using the$_SESSION
superglobal array. The data in this array will be available throughout the user’s session.
<?php
// Storing data in the session
$_SESSION['username'] = 'JohnDoe';
$_SESSION['email'] = '[email protected]';
?>
- Retrieving Data from a Session:
You can retrieve the data stored in the session using the$_SESSION
superglobal array.
<?php
// Retrieving data from the session
if (isset($_SESSION['username'])) {
echo "Welcome back, " . $_SESSION['username'];
} else {
echo "Welcome, guest!";
}
?>
- Removing Data from a Session:
To remove specific data from the session, you can use theunset()
function on the corresponding session variable.
<?php
// Removing data from the session
unset($_SESSION['email']);
?>
- Destroying a Session:
To end the session and remove all stored data associated with it, you can use thesession_destroy()
function.
<?php
// Destroy the session
session_destroy();
?>
Remember that to use sessions in PHP, you need to have the session support enabled in the PHP configuration file (php.ini
). Also, to work with sessions, the server must be able to store session data for each user. By default, PHP stores session data on the server’s file system, but you can also configure it to use other storage mechanisms like a database.
It’s essential to handle sessions securely and avoid storing sensitive data in the session. Additionally, ensure that your PHP application is properly configured to handle sessions, especially when dealing with user authentication and authorization.