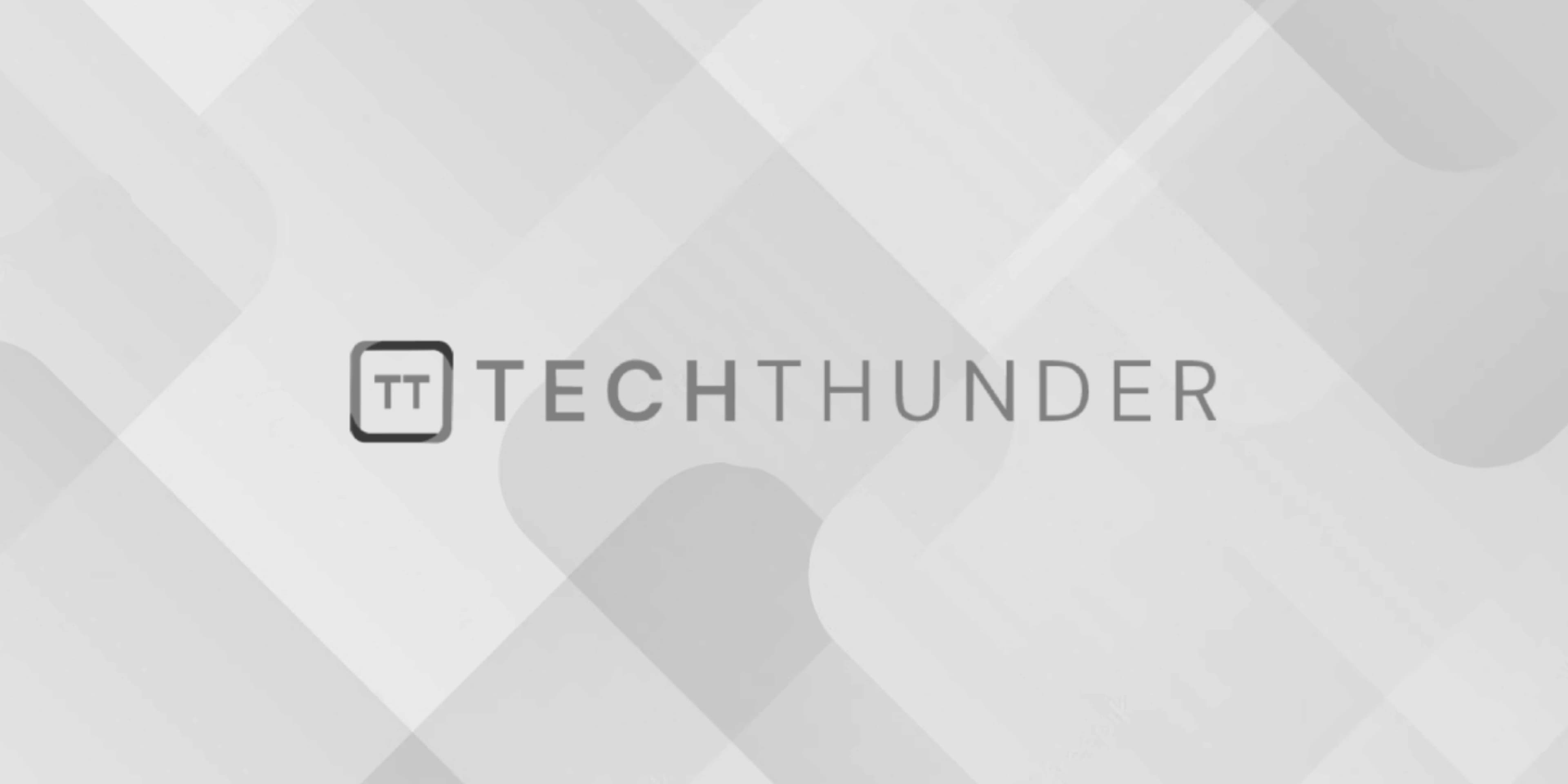
PHP Registration Form
Creating a PHP registration form involves creating an HTML form to capture user details and using PHP to process the form data and perform user registration. Below is a basic example of a PHP registration form:
index.html (HTML form):
<!DOCTYPE html>
<html>
<head>
<title>User Registration Form</title>
</head>
<body>
<h2>User Registration</h2>
<form action="register.php" method="post">
<label for="username">Username:</label>
<input type="text" name="username" required><br>
<label for="email">Email:</label>
<input type="email" name="email" required><br>
<label for="password">Password:</label>
<input type="password" name="password" required><br>
<input type="submit" name="submit" value="Register">
</form>
</body>
</html>
register.php (PHP form processing):
<?php
// Check if the form is submitted
if ($_SERVER["REQUEST_METHOD"] === "POST") {
// Get form data
$username = $_POST["username"];
$email = $_POST["email"];
$password = $_POST["password"];
// Perform basic validation (you can add more validation as needed)
if (empty($username) || empty($email) || empty($password)) {
echo "All fields are required.";
} else {
// Database connection (replace with your actual database credentials)
$dbHost = "localhost";
$dbUser = "your_db_username";
$dbPass = "your_db_password";
$dbName = "your_db_name";
$conn = mysqli_connect($dbHost, $dbUser, $dbPass, $dbName);
if (!$conn) {
die("Database connection failed: " . mysqli_connect_error());
}
// Escape form data to prevent SQL injection
$username = mysqli_real_escape_string($conn, $username);
$email = mysqli_real_escape_string($conn, $email);
$password = password_hash($password, PASSWORD_DEFAULT); // Hash the password
// Insert user data into the database (replace 'users' with your actual table name)
$sql = "INSERT INTO users (username, email, password) VALUES ('$username', '$email', '$password')";
if (mysqli_query($conn, $sql)) {
echo "Registration successful!";
} else {
echo "Error: " . mysqli_error($conn);
}
// Close database connection
mysqli_close($conn);
}
}
?>
Note: The above example assumes you have a MySQL database and a table named ‘users’ with columns for ‘username’, ’email’, and ‘password’. Additionally, it’s essential to use prepared statements or an ORM (Object-Relational Mapping) library to prevent SQL injection attacks. The example above provides only basic validation and should be expanded to include additional security measures based on your specific needs.
Before deploying a registration form on a live website, always ensure that you follow best practices for security, such as using HTTPS, validating user inputs thoroughly, and implementing additional authentication and authorization mechanisms as required.