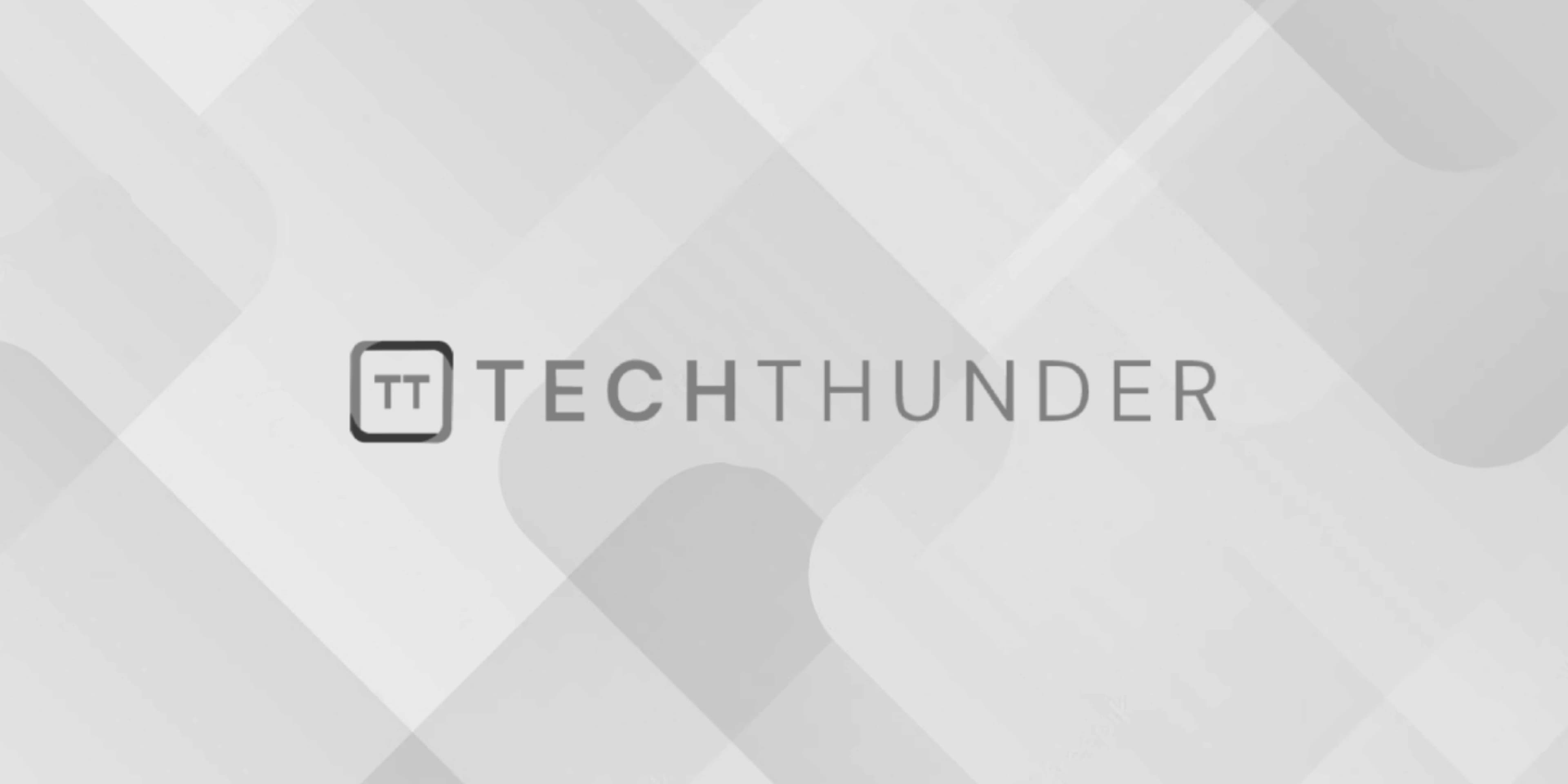
How to Encrypt or Decrypt a String in PHP
Encrypting and decrypting strings in PHP can be achieved using various cryptographic algorithms. One of the common methods for encryption is using the openssl_encrypt()
and openssl_decrypt()
functions, which use the OpenSSL library for encryption and decryption.
Here’s a step-by-step guide on how to encrypt and decrypt a string in PHP using OpenSSL:
- Generating a Cryptographically Secure Key:
Before encrypting and decrypting data, you need a strong encryption key. You can use a secure method to generate a key, such as theopenssl_random_pseudo_bytes()
function. The generated key should be kept secret and stored securely.
// Generate a cryptographically secure encryption key
$encryptionKey = openssl_random_pseudo_bytes(32); // 32 bytes key (256 bits)
- Encrypting a String:
Use theopenssl_encrypt()
function to encrypt a string. It takes the plaintext string, the encryption method (cipher), and the encryption key as arguments.
function encryptString($plaintext, $encryptionKey) {
$cipher = "AES-256-CBC"; // AES encryption with 256-bit key and CBC mode
$ivLength = openssl_cipher_iv_length($cipher);
$iv = openssl_random_pseudo_bytes($ivLength);
$ciphertext = openssl_encrypt($plaintext, $cipher, $encryptionKey, OPENSSL_RAW_DATA, $iv);
$encryptedData = $iv . $ciphertext;
return base64_encode($encryptedData); // Encoded for safe storage or transmission
}
- Decrypting a String:
Use theopenssl_decrypt()
function to decrypt an encrypted string. It takes the ciphertext, the encryption method (cipher), and the encryption key as arguments.
function decryptString($encryptedData, $encryptionKey) {
$cipher = "AES-256-CBC"; // AES encryption with 256-bit key and CBC mode
$ivLength = openssl_cipher_iv_length($cipher);
$encryptedData = base64_decode($encryptedData); // Decode from base64
$iv = substr($encryptedData, 0, $ivLength);
$ciphertext = substr($encryptedData, $ivLength);
$plaintext = openssl_decrypt($ciphertext, $cipher, $encryptionKey, OPENSSL_RAW_DATA, $iv);
return $plaintext;
}
Example Usage:
$plaintext = "Hello, this is a secret message!";
$encryptedData = encryptString($plaintext, $encryptionKey);
echo "Encrypted: " . $encryptedData . "\n";
$decryptedData = decryptString($encryptedData, $encryptionKey);
echo "Decrypted: " . $decryptedData . "\n";
In this example, the $encryptionKey
is generated using the openssl_random_pseudo_bytes()
function. The encryptString()
function encrypts the input plaintext string using AES-256 encryption with Cipher Block Chaining (CBC) mode. The result is base64-encoded for safe storage or transmission.
The decryptString()
function takes the base64-encoded ciphertext, extracts the IV (initialization vector) and ciphertext, and then decrypts the data using the same encryption key and IV.
Remember to handle encryption keys securely and store them in a safe place, as the security of your encrypted data relies on the strength and secrecy of the encryption key.