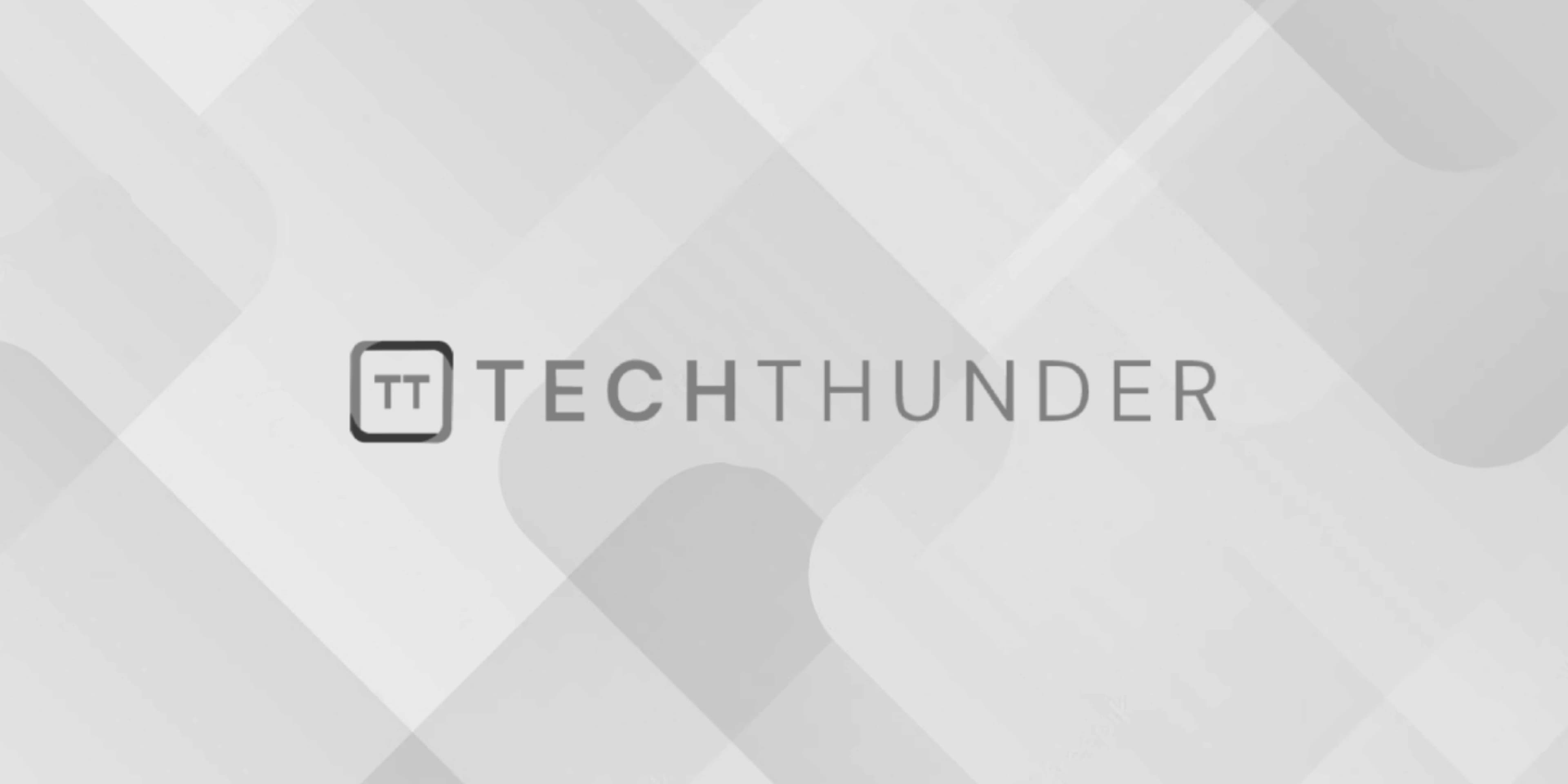
PHP Imagestring() Function
In PHP, imagestring()
is a function used to draw a string of text on an image created with the GD (GIF Draw) library. The GD library is commonly used in PHP for creating and manipulating images.
Here’s the syntax of the imagestring()
function:
bool imagestring(resource $image, int $font, int $x, int $y, string $string, int $color)
Parameters:
$image
: The GD image resource obtained from functions likeimagecreate()
orimagecreatetruecolor()
.$font
: An integer representing the font style. GD has built-in font styles from 1 to 5, where 1 is the smallest font and 5 is the largest.$x
: The x-coordinate (horizontal position) where the string will be drawn on the image.$y
: The y-coordinate (vertical position) where the string will be drawn on the image.$string
: The text string to be drawn on the image.$color
: The color identifier obtained from functions likeimagecolorallocate()
.
Return value:
- The function returns
true
on success orfalse
on failure.
Example:
// Create a new image with width 400 pixels and height 200 pixels
$imageWidth = 400;
$imageHeight = 200;
$image = imagecreatetruecolor($imageWidth, $imageHeight);
// Allocate colors for the background (white) and text (black)
$backgroundColor = imagecolorallocate($image, 255, 255, 255); // White
$textColor = imagecolorallocate($image, 0, 0, 0); // Black
// Set the background color
imagefill($image, 0, 0, $backgroundColor);
// Draw text on the image
$text = "Hello, PHP!";
imagestring($image, 5, 50, 100, $text, $textColor);
// Set the content type header to output the image as PNG
header('Content-Type: image/png');
// Output the image as PNG to the browser
imagepng($image);
// Free up memory by destroying the image resource
imagedestroy($image);
In this example, we create a new true-color image with a width of 400 pixels and a height of 200 pixels. We allocate colors for the background (white) and the text (black). We set the background color for the entire image using imagefill()
. Then, we draw the text “Hello, PHP!” on the image using imagestring()
with font style 5 at coordinates (50, 100). Finally, we output the image as PNG to the browser using imagepng()
and free up memory by destroying the image resource with imagedestroy()
.
The imagestring()
function is suitable for basic text rendering on images. For more advanced text formatting and custom fonts, consider using other GD functions like imagettftext()
, which supports TrueType fonts and provides more control over the appearance of the text.