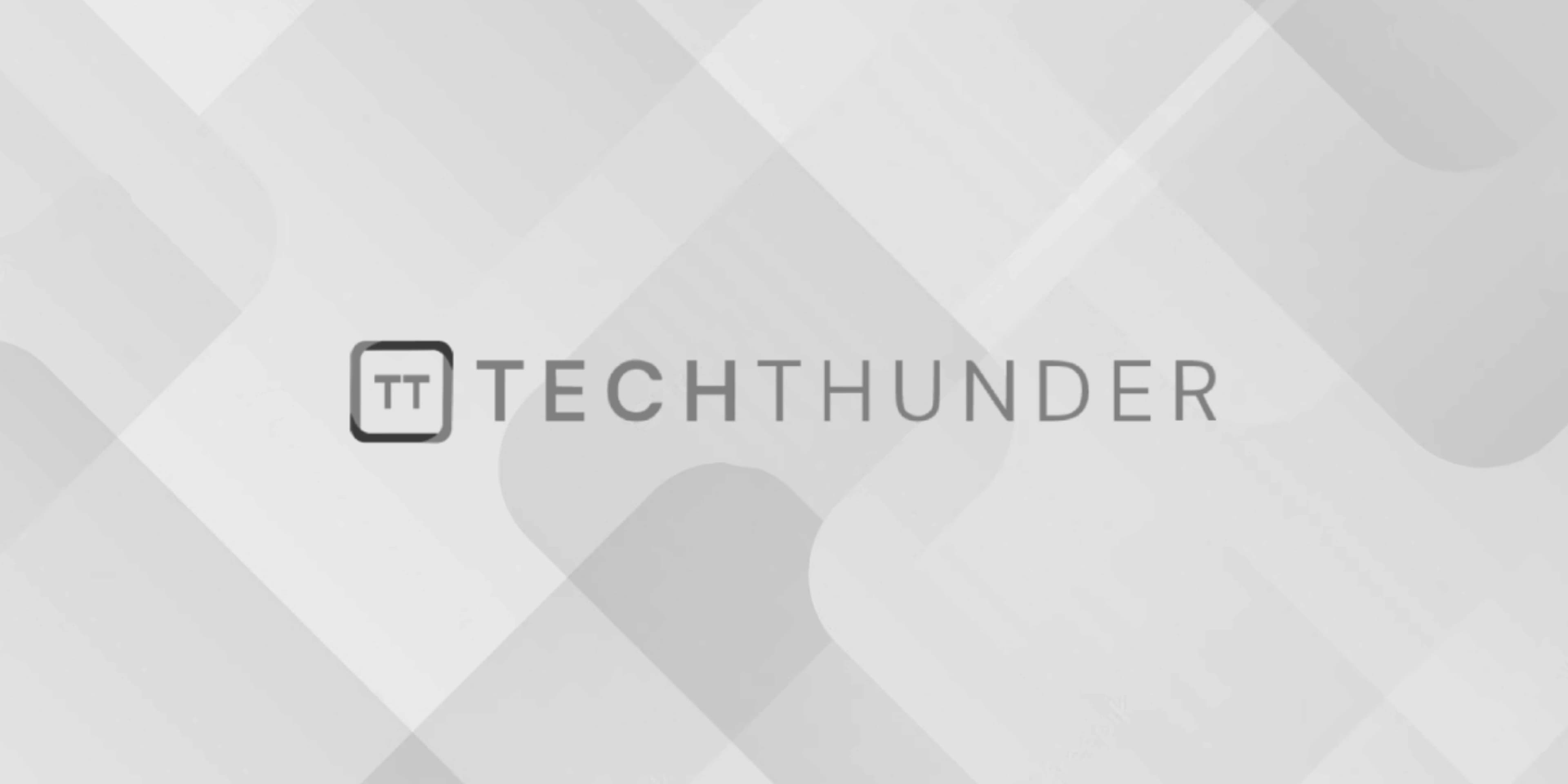
PHP Two-way Encryption
Two-way encryption, also known as symmetric encryption, is a method of encrypting and decrypting data using the same secret key. The data is encrypted using the key and can be decrypted back to its original form using the same key. PHP provides various encryption functions that can be used for two-way encryption. In this example, I’ll demonstrate how to use the openssl_encrypt()
and openssl_decrypt()
functions for two-way encryption.
<?php
// Data to encrypt
$data = "Sensitive information that needs to be encrypted.";
// Encryption key (should be kept secret and securely stored)
$encryptionKey = "YourEncryptionKey"; // Replace with your own strong encryption key
// Encryption algorithm
$algorithm = "AES-256-CBC"; // AES encryption with 256-bit key and CBC mode
// Initialization vector (IV) - random bytes
$iv = openssl_random_pseudo_bytes(openssl_cipher_iv_length($algorithm));
// Encrypt the data
$encryptedData = openssl_encrypt($data, $algorithm, $encryptionKey, OPENSSL_RAW_DATA, $iv);
// To store or transmit the encrypted data, you might want to encode it using base64
$encodedEncryptedData = base64_encode($iv . $encryptedData);
// Decrypt the data
$decodedEncryptedData = base64_decode($encodedEncryptedData);
$decryptedData = openssl_decrypt($decodedEncryptedData, $algorithm, $encryptionKey, OPENSSL_RAW_DATA, $iv);
echo "Original Data: " . $data . "\n";
echo "Decrypted Data: " . $decryptedData . "\n";
?>
In this example, we use the openssl_encrypt()
function to encrypt the data using AES encryption with a 256-bit key and Cipher Block Chaining (CBC) mode. The encryption key is used for both encryption and decryption. The initialization vector (IV) is used to add randomness to the encryption process, ensuring that the same data encrypted with the same key results in different ciphertexts.
After encrypting the data, we encode it using base64 to ensure safe storage or transmission. During decryption, we decode the base64-encoded data and use openssl_decrypt()
to retrieve the original data.
Remember to handle encryption keys securely and store them in a safe place, as the security of your encrypted data relies on the strength and secrecy of the encryption key. Additionally, consider using HTTPS for transmitting sensitive data to enhance security during data transmission.