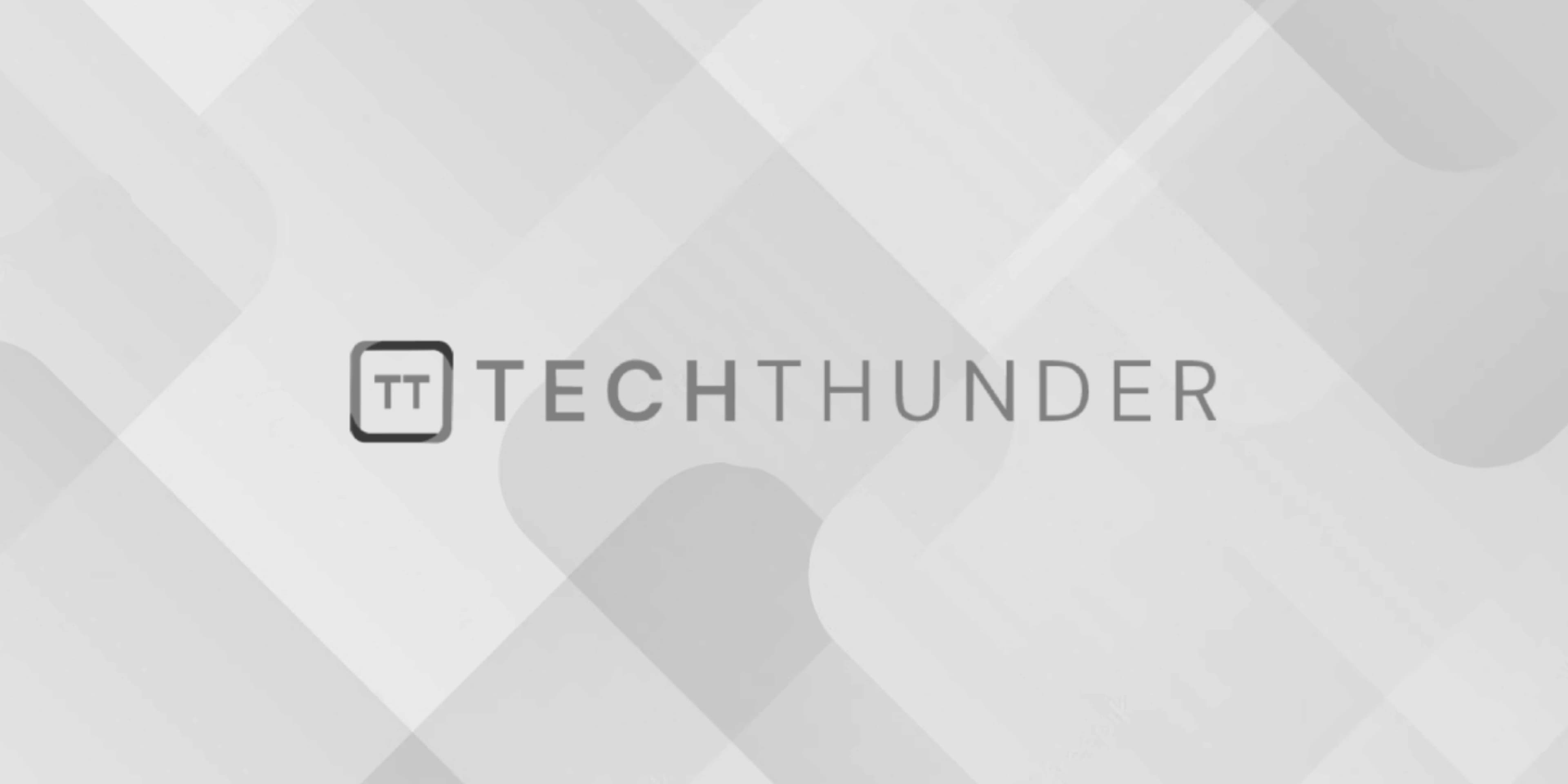
PHP Code
Sure! Here’s a simple PHP code example that demonstrates the basic syntax and features:
<!DOCTYPE html>
<html>
<head>
<title>PHP Example</title>
</head>
<body>
<?php
// PHP code starts here
// Variables and Data Types
$name = "John";
$age = 30;
$isStudent = true;
// Outputting content
echo "<h1>Hello, PHP!</h1>";
echo "My name is " . $name . ". I am " . $age . " years old.";
// Conditional statement
if ($isStudent) {
echo "<p>I am a student.</p>";
} else {
echo "<p>I am not a student.</p>";
}
// Arrays
$fruits = array("Apple", "Banana", "Orange");
echo "<ul>";
foreach ($fruits as $fruit) {
echo "<li>$fruit</li>";
}
echo "</ul>";
// Function
function multiply($a, $b) {
return $a * $b;
}
$result = multiply(5, 6);
echo "5 multiplied by 6 is: " . $result;
// PHP code ends here
?>
</body>
</html>
In this example, the PHP code is embedded within an HTML document using the <?php ... ?>
tags. The PHP code initializes variables, echoes HTML content with dynamic PHP data, demonstrates a simple conditional statement, loops through an array, and defines a custom function.
When you run this PHP script in a web server environment, it will output an HTML page with the dynamic content generated by PHP. The output will include the person’s name, age, student status, a list of fruits, and the result of the multiplication function.
Remember to save this code as a .php
file (e.g., index.php
) and place it in the appropriate web server directory (e.g., htdocs
in XAMPP or WAMP, /var/www/html/
in Ubuntu/Debian). Then, access the script through your web browser (e.g., http://localhost/index.php) to see the output.