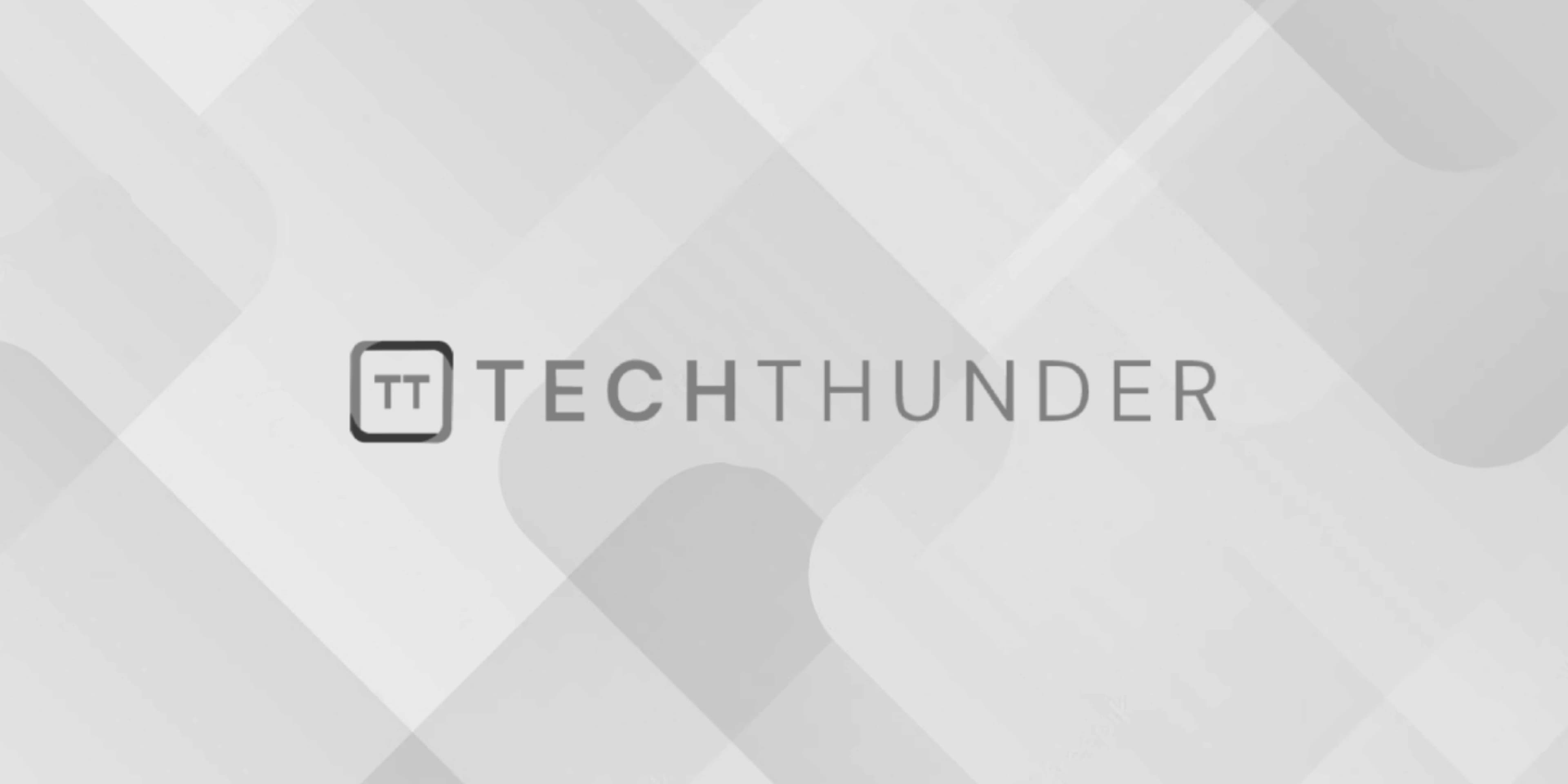
223 views
Image Gallery CRUD using PHP MySQL
Creating an image gallery with CRUD (Create, Read, Update, Delete) functionality using PHP and MySQL involves several steps. Below is a step-by-step guide to implement an image gallery with PHP and MySQL:
- Set Up Database:
Create a MySQL database and a table to store the image details. The table should have columns to store the image ID, image name, and image file path.
CREATE DATABASE image_gallery;
USE image_gallery;
CREATE TABLE images (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255) NOT NULL,
file_path VARCHAR(255) NOT NULL
);
- Create the Image Upload Form:
Create an HTML form to allow users to upload images. The form should have an input field of typefile
to select the image and a submit button to upload it.
<!DOCTYPE html>
<html>
<head>
<title>Image Gallery</title>
</head>
<body>
<h2>Upload Image</h2>
<form action="upload.php" method="post" enctype="multipart/form-data">
<label for="image">Select Image:</label>
<input type="file" name="image" id="image" required>
<input type="submit" value="Upload">
</form>
</body>
</html>
- Create the PHP Upload Script (upload.php):
Create a PHP script (upload.php) to handle the image upload. The script will move the uploaded image to a designated folder and store its details in the database.
<?php
if (isset($_FILES['image']) && $_FILES['image']['error'] === UPLOAD_ERR_OK) {
$uploadsDir = 'uploads/';
$uploadedFile = $_FILES['image'];
$imageName = $uploadedFile['name'];
$imageTmpPath = $uploadedFile['tmp_name'];
$imageFilePath = $uploadsDir . $imageName;
if (move_uploaded_file($imageTmpPath, $imageFilePath)) {
// Store image details in the database
$dbHost = "localhost";
$dbUser = "your_db_username";
$dbPass = "your_db_password";
$dbName = "image_gallery";
$conn = new mysqli($dbHost, $dbUser, $dbPass, $dbName);
if ($conn->connect_error) {
die("Database connection failed: " . $conn->connect_error);
}
$name = $conn->real_escape_string($imageName);
$filePath = $conn->real_escape_string($imageFilePath);
$sql = "INSERT INTO images (name, file_path) VALUES ('$name', '$filePath')";
if ($conn->query($sql) === TRUE) {
echo "Image uploaded successfully.";
} else {
echo "Error: " . $conn->error;
}
$conn->close();
} else {
echo "Failed to upload the image.";
}
} else {
echo "Image upload failed.";
}
?>
- Create the Image Gallery (index.php):
Create an index.php file to display the image gallery. The PHP script will retrieve the images from the database and display them as thumbnails.
<!DOCTYPE html>
<html>
<head>
<title>Image Gallery</title>
</head>
<body>
<h2>Image Gallery</h2>
<?php
// Fetch images from the database
$dbHost = "localhost";
$dbUser = "your_db_username";
$dbPass = "your_db_password";
$dbName = "image_gallery";
$conn = new mysqli($dbHost, $dbUser, $dbPass, $dbName);
if ($conn->connect_error) {
die("Database connection failed: " . $conn->connect_error);
}
$sql = "SELECT * FROM images";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
while ($row = $result->fetch_assoc()) {
echo '<img src="' . $row['file_path'] . '" alt="' . $row['name'] . '" width="200" height="150">';
}
} else {
echo "No images found.";
}
$conn->close();
?>
</body>
</html>
- Implement the Delete Functionality:
Add a “Delete” button to each image thumbnail to allow users to delete images from the gallery. When the “Delete” button is clicked, the PHP script will delete the corresponding image from the database and the uploads folder.
Note: For security reasons, always validate and sanitize user input to prevent SQL injection and other potential vulnerabilities.
This example provides a basic implementation of an image gallery with CRUD functionality using PHP and MySQL. For a complete application, you may want to enhance the design, add pagination for large image collections, implement image editing, or include user authentication to protect the gallery from unauthorized access.