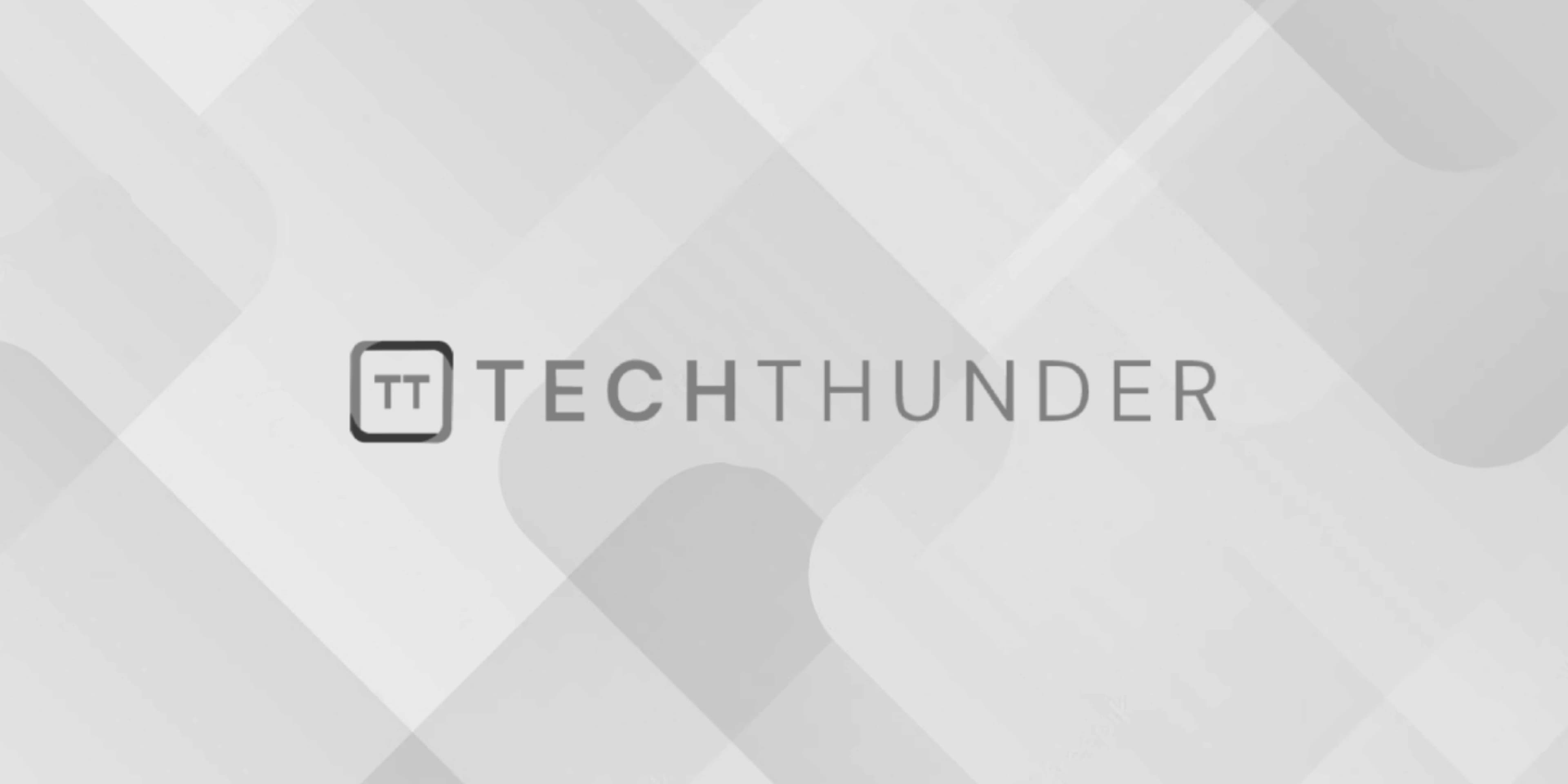
219 views
PHP Associative Array
In PHP, an associative array is a collection of key-value pairs, where each value is associated with a unique key. Unlike indexed arrays, which use numeric indices, associative arrays use custom keys that can be of different data types, such as strings or integers.
Here’s how you can create and work with associative arrays in PHP:
- Creating an Associative Array:
You can create an associative array using the array() construct or the shorter square bracket syntax.
Example:
// Using array() construct
$student = array(
"name" => "John Doe",
"age" => 25,
"gender" => "Male",
"email" => "[email protected]"
);
or
// Using square bracket syntax (since PHP 5.4)
$student = [
"name" => "John Doe",
"age" => 25,
"gender" => "Male",
"email" => "[email protected]"
];
- Accessing Elements of an Associative Array:
You can access elements of an associative array using their corresponding keys.
Example:
echo $student["name"]; // Output: John Doe
echo $student["age"]; // Output: 25
- Modifying Elements of an Associative Array:
You can modify elements of an associative array by assigning new values to their corresponding keys.
Example:
$student["age"] = 26;
echo $student["age"]; // Output: 26
- Adding Elements to an Associative Array:
You can add new key-value pairs to an associative array using the square bracket notation.
Example:
$student["location"] = "New York";
- Looping through an Associative Array:
You can use loops likeforeach
to iterate through the key-value pairs of an associative array.
Example:
foreach ($student as $key => $value) {
echo $key . ": " . $value . "\n";
}
Output:
name: John Doe
age: 26
gender: Male
email: [email protected]
location: New York
- Checking if a Key Exists in an Associative Array:
You can use theisset()
function to check if a specific key exists in an associative array.
Example:
if (isset($student["name"])) {
echo "Name exists.";
} else {
echo "Name does not exist.";
}
Associative arrays are useful for representing data with meaningful labels or identifiers, making it easier to work with and understand the data within PHP applications.