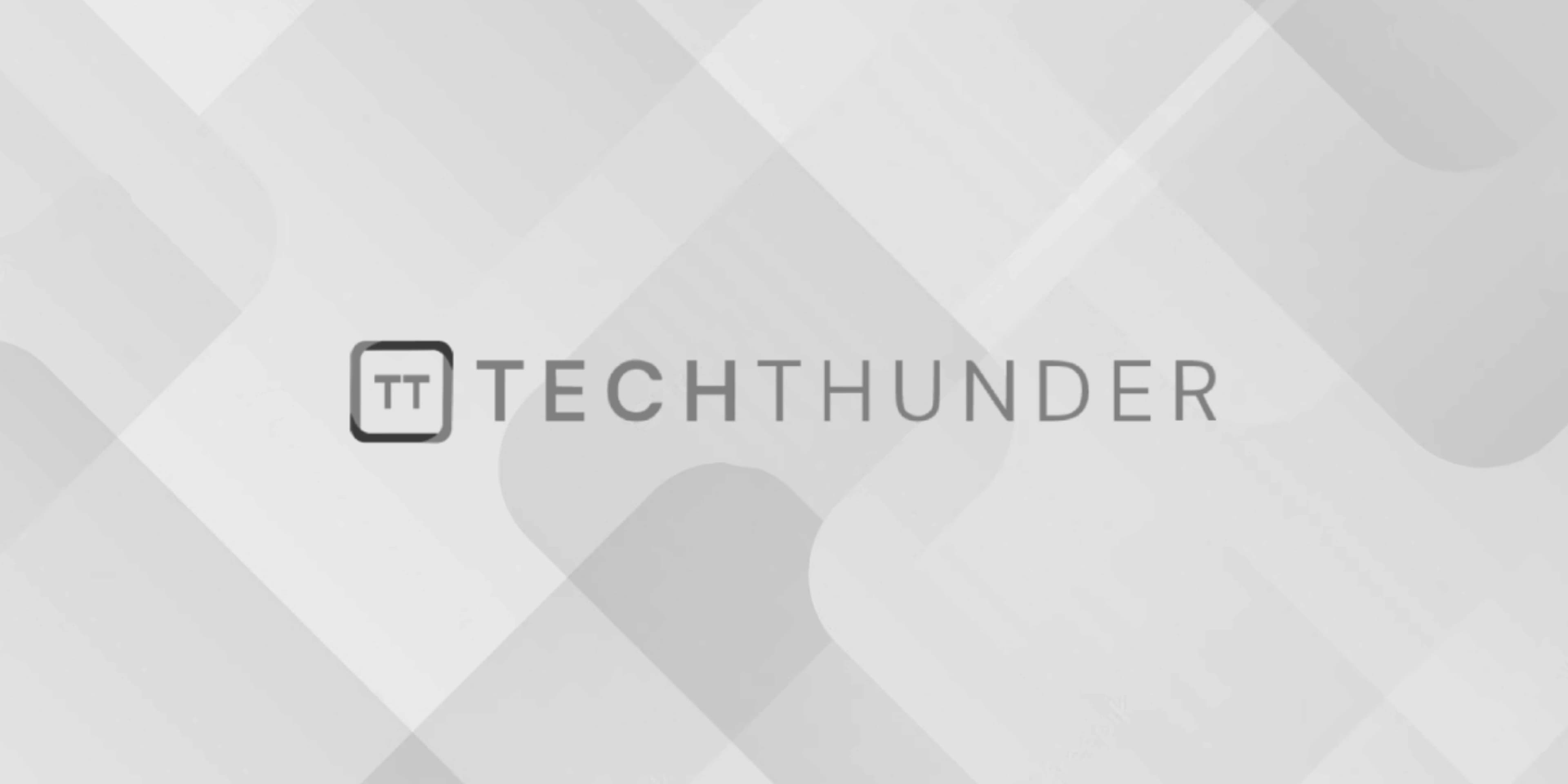
Polymorphism in PHP
Polymorphism is a fundamental object-oriented programming concept that allows objects of different classes to be treated as objects of a common base class. In PHP, polymorphism is achieved through inheritance and method overriding. It enables code to be more flexible, reusable, and easier to maintain.
Let’s see how polymorphism works in PHP using an example:
Suppose we have a base class called Shape
, and two derived classes called Circle
and Square
. The Shape
class has a common method calculateArea()
that calculates the area of different shapes. Both Circle
and Square
inherit from the Shape
class and implement their versions of the calculateArea()
method.
// Base class
class Shape {
public function calculateArea() {
// The implementation of this method will be overridden by derived classes.
}
}
// Derived class Circle
class Circle extends Shape {
private $radius;
public function __construct($radius) {
$this->radius = $radius;
}
public function calculateArea() {
return 3.14 * $this->radius * $this->radius;
}
}
// Derived class Square
class Square extends Shape {
private $side;
public function __construct($side) {
$this->side = $side;
}
public function calculateArea() {
return $this->side * $this->side;
}
}
Now, we can create objects of both Circle
and Square
and treat them as objects of the common base class Shape
. This is where polymorphism comes into play. We can use the calculateArea()
method on both objects, and PHP will automatically call the appropriate implementation based on the actual type of the object at runtime.
$circle = new Circle(5);
$square = new Square(4);
// Polymorphism in action
function printArea(Shape $shape) {
echo "Area: " . $shape->calculateArea() . "\n";
}
printArea($circle); // Output: Area: 78.5
printArea($square); // Output: Area: 16
In this example, both Circle
and Square
are treated as Shape
objects when passed to the printArea()
function. The actual implementation of calculateArea()
called depends on the object’s type. This flexibility allows us to write generic code that can work with different types of objects and still achieve the correct behavior for each specific subclass.