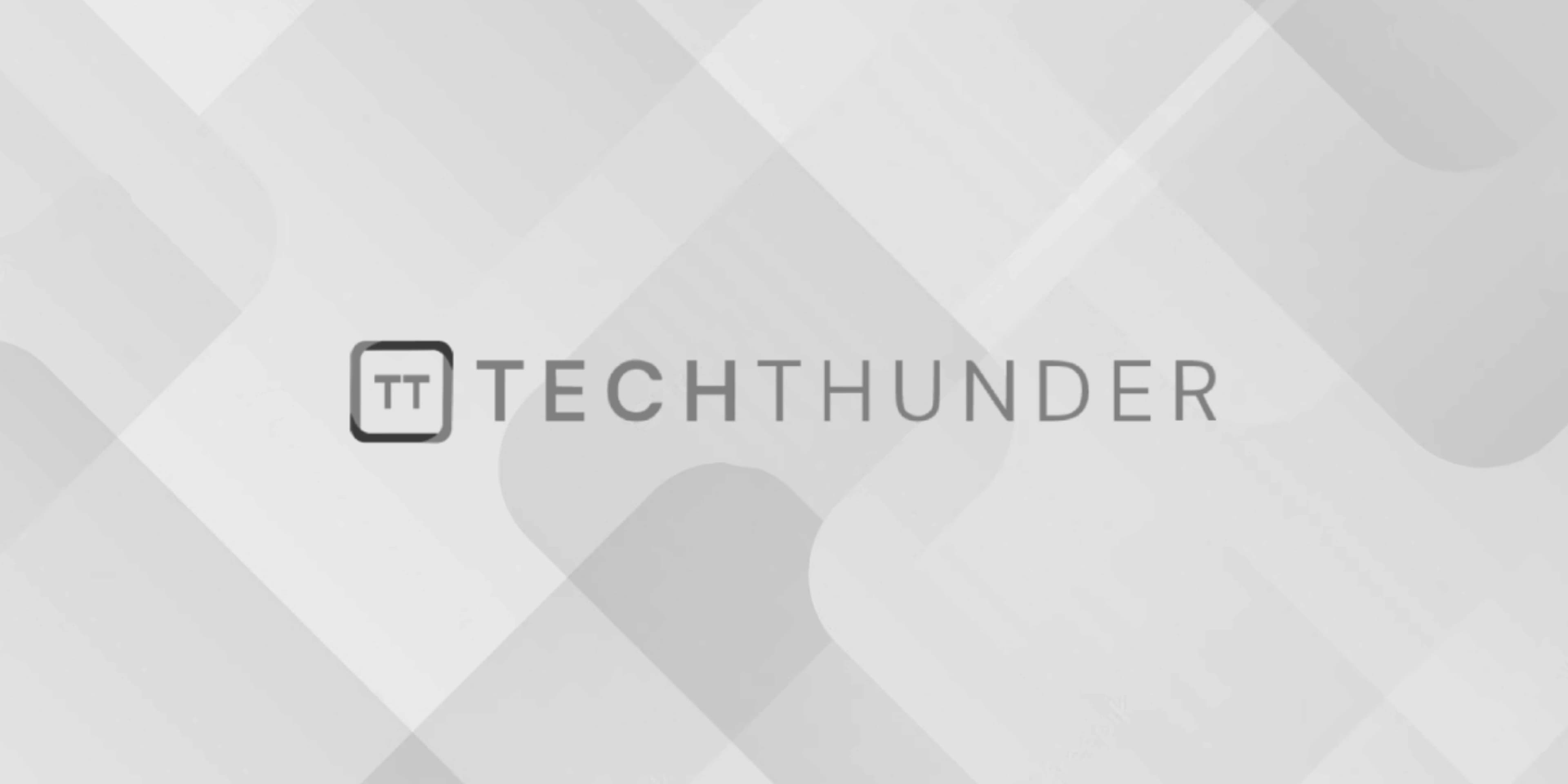
PHP gmp_xor() function
In PHP, the gmp_xor()
function is used to perform bitwise XOR (exclusive OR) operation on two GMP (GNU Multiple Precision) numbers. GMP is a PHP extension that allows you to perform arbitrary precision arithmetic on large integers, overcoming the limitations of native PHP integers.
The gmp_xor()
function takes two GMP numbers as its arguments and returns a new GMP number that represents the result of the XOR operation between the two input numbers.
Here’s the syntax of the gmp_xor()
function:
gmp_xor ( GMP $num1 , GMP $num2 ) : GMP
Parameters:
$num1
: The first GMP number for the XOR operation.$num2
: The second GMP number for the XOR operation.
Return Value:
- The function returns a new GMP number representing the result of the XOR operation between
$num1
and$num2
.
Example:
<?php
// Perform bitwise XOR on two GMP numbers
$gmpNum1 = gmp_init("10", 2); // Binary representation of 10
$gmpNum2 = gmp_init("6", 2); // Binary representation of 6
$result = gmp_xor($gmpNum1, $gmpNum2);
// Convert the result back to binary representation
$binaryResult = gmp_strval($result, 2);
echo "Binary Result: " . $binaryResult; // Output: Binary Result: 100
?>
In this example, we perform a bitwise XOR operation between two GMP numbers representing the binary values 10 and 6. The result of the XOR operation is 100, which is the binary representation of 4 in decimal.
Since GMP functions work with large integers, they are particularly useful when dealing with numbers that exceed the size limits of native PHP integers. They allow you to handle integer arithmetic with arbitrary precision and without losing precision due to integer overflow.