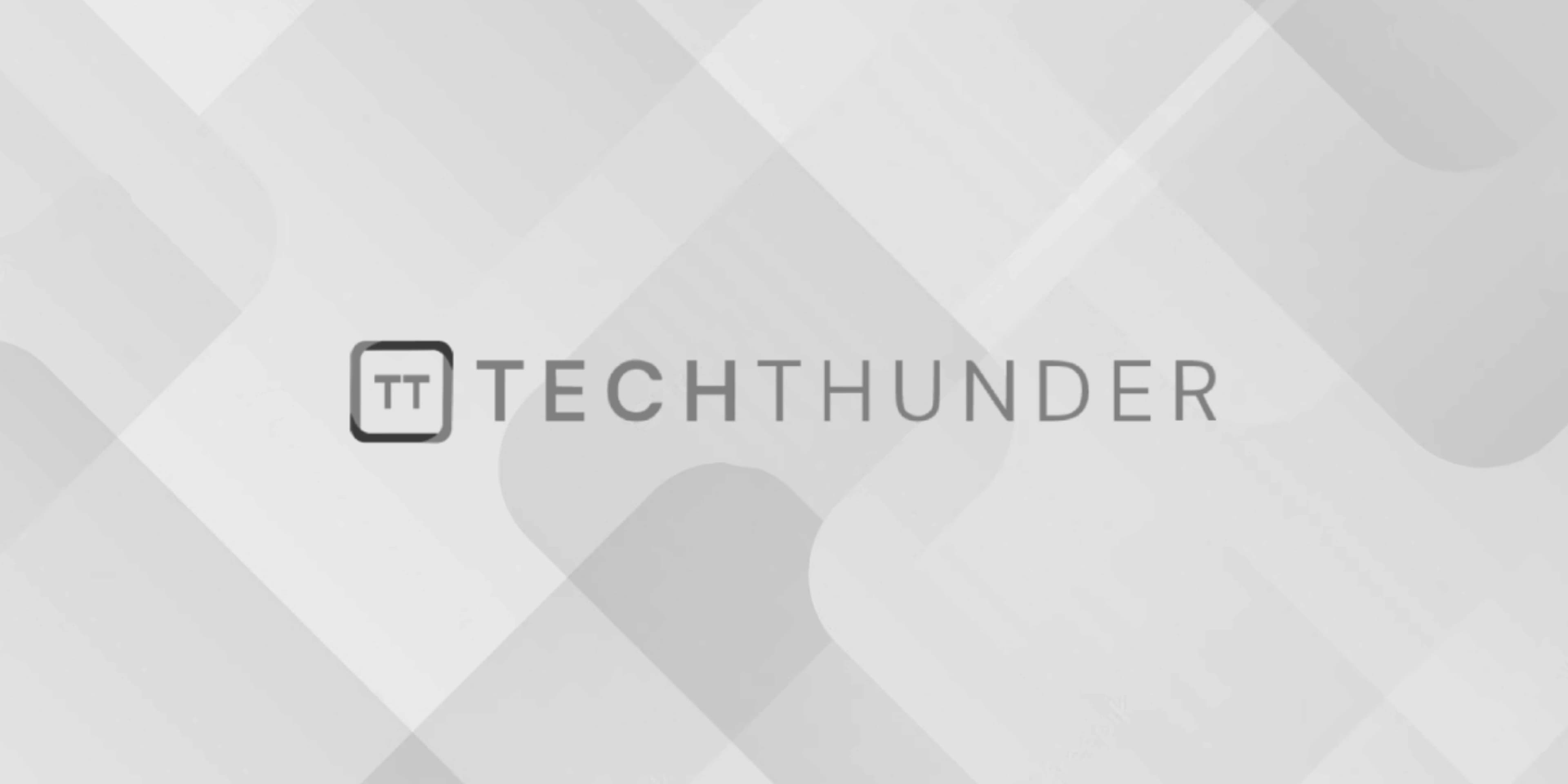
How to get selected option value in PHP
To get the selected option value from an HTML form in PHP, you need to use the $_POST
or $_GET
superglobal arrays, depending on the form’s method attribute (post or get). Here’s how you can do it:
- HTML Form:
Create an HTML form with a select dropdown input. The select element should have a name attribute to identify the field when the form is submitted.
<!DOCTYPE html>
<html>
<head>
<title>Get Selected Option Value</title>
</head>
<body>
<h2>Select Your Favorite Color</h2>
<form action="process_form.php" method="post">
<label for="color">Color:</label>
<select name="color" id="color">
<option value="red">Red</option>
<option value="blue">Blue</option>
<option value="green">Green</option>
<option value="yellow">Yellow</option>
</select>
<input type="submit" value="Submit">
</form>
</body>
</html>
- PHP Processing Script (process_form.php):
In the PHP processing script (process_form.php), you can access the selected option value by using the$_POST
superglobal array.
<?php
if (isset($_POST['color'])) {
$selectedColor = $_POST['color'];
echo "Your selected color is: " . $selectedColor;
} else {
echo "Please select a color.";
}
?>
In this example, when the user submits the form, the process_form.php
script will check if the ‘color’ key exists in the $_POST
array. If it does, it means the form was submitted with a selected option, and the selected color will be displayed. If the ‘color’ key is not present, it means the form was submitted without a selection, and a message asking the user to select a color will be displayed.
Remember to properly validate and sanitize user input before using it in your PHP code to prevent security vulnerabilities such as SQL injection or cross-site scripting (XSS) attacks.