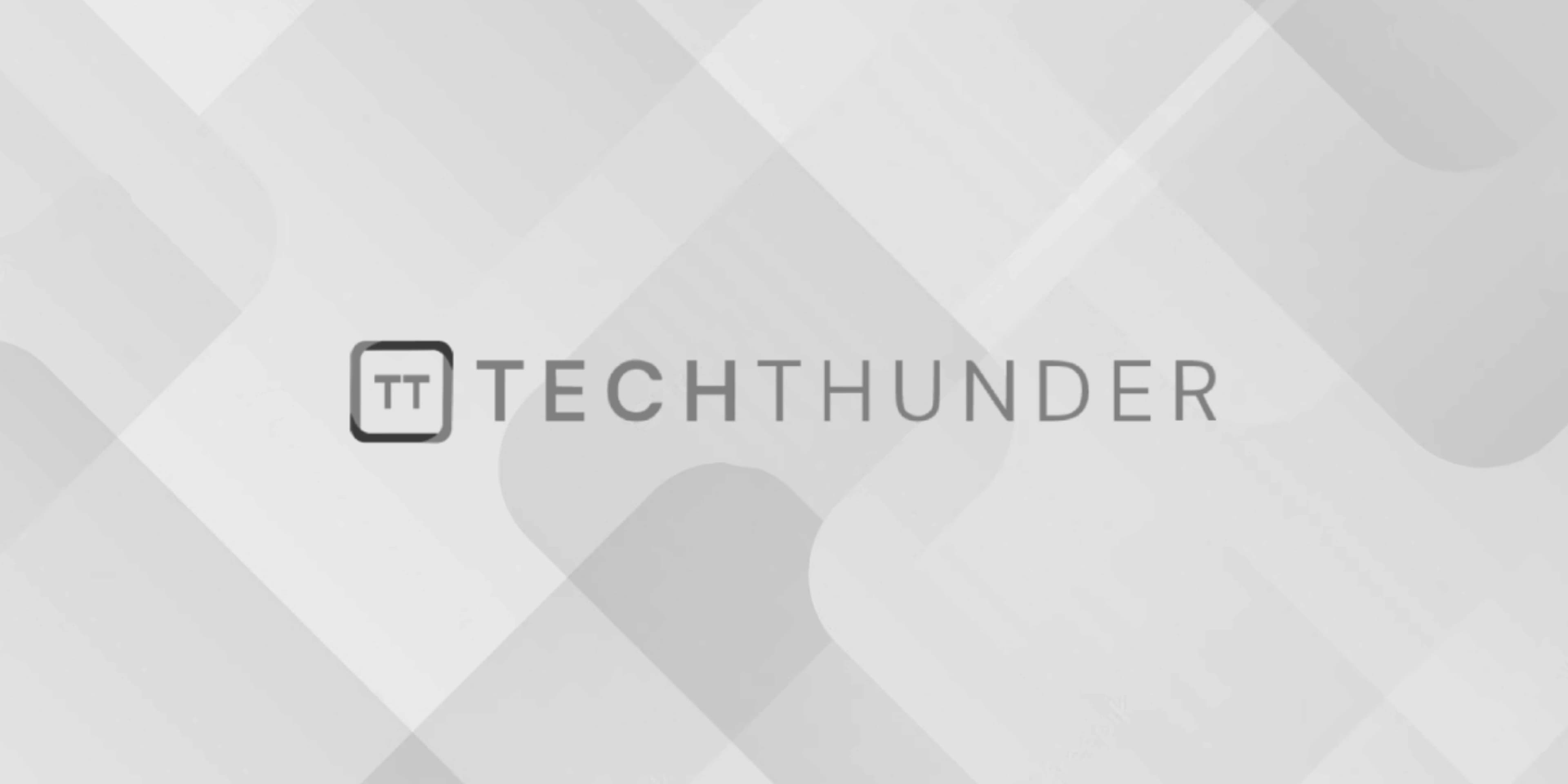
PHP Default Arguments
In PHP, you can define default values for function parameters, which allows you to call a function with fewer arguments than the number of parameters defined. If a value is not provided for a parameter during the function call, PHP will use the default value specified in the function declaration.
Here’s how you can define default arguments in PHP functions:
function greet($name, $message = "Hello") {
echo $message . ", " . $name . "!";
}
greet("John"); // Output: Hello, John!
greet("Jane", "Hi"); // Output: Hi, Jane!
In the example above, we define a function greet
that takes two parameters: $name
and $message
. The $message
parameter has a default value of “Hello” assigned to it. When we call the function with only the $name
argument (greet("John")
), the $message
parameter uses its default value (“Hello”). When we call the function with both $name
and $message
arguments (greet("Jane", "Hi")
), the $message
parameter uses the provided value (“Hi”).
Important points to remember when using default arguments:
- Default arguments must be defined after the non-default arguments in the function declaration. For example,
$name
must come before$message
in thegreet
function. - You can have multiple default arguments in a single function, but they should be declared from left to right.
- If you define default values for some parameters, all subsequent parameters must have default values as well. You cannot have non-default parameters after default parameters in the function declaration.
Default arguments are useful when you want to provide a fallback value for a function parameter, but you also want to allow callers to override the default value when needed. They help make functions more flexible and reduce the need for function overloading (creating multiple functions with different parameter lists).