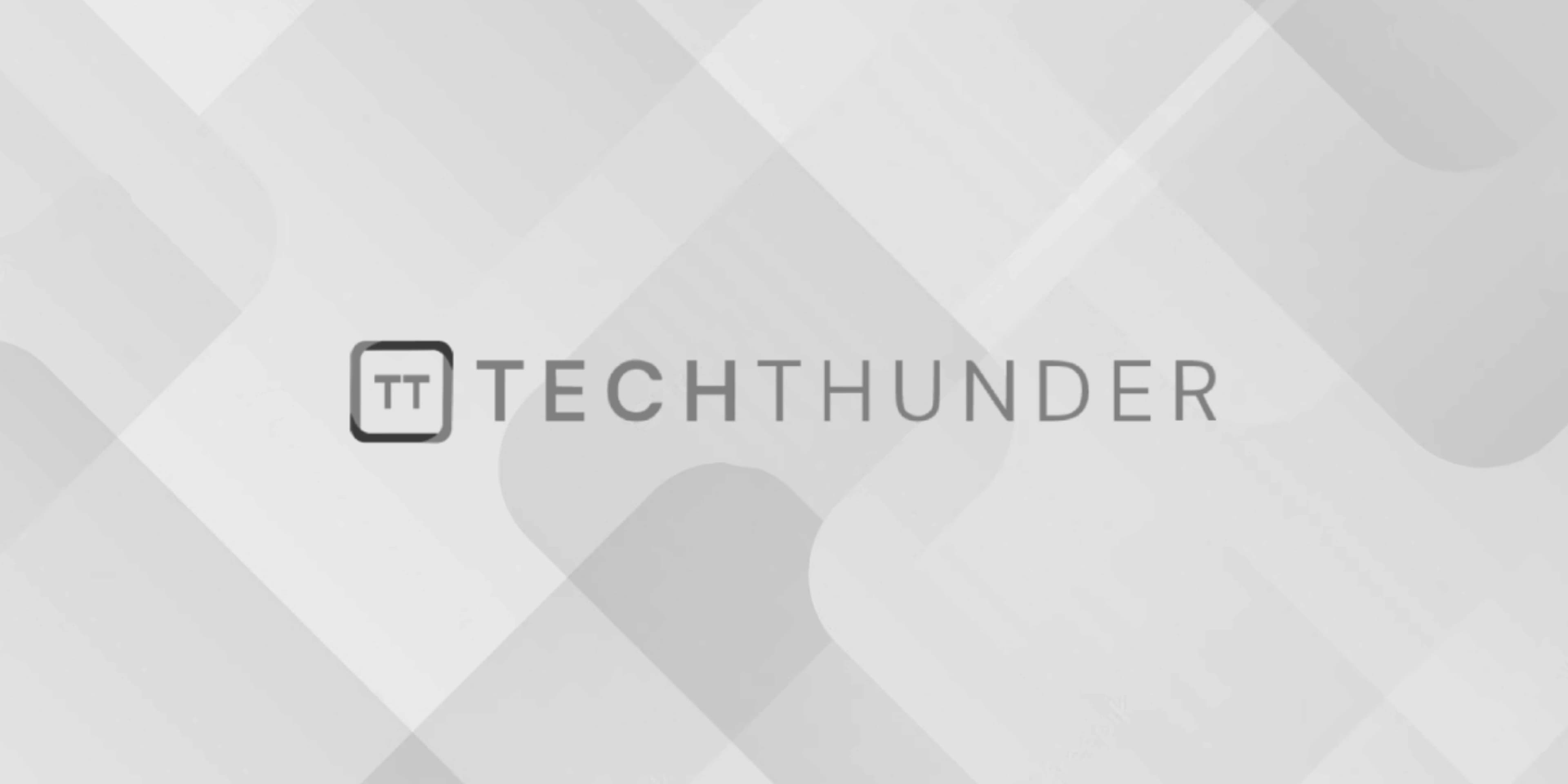
PHP Variable Arguments
In PHP, you can create functions that accept a variable number of arguments using what is known as “variable-length argument lists” or “variable arguments.” This allows you to pass an arbitrary number of arguments to a function, even if the number of parameters is not fixed.
To define a function with variable arguments, you use the func_num_args()
, func_get_arg()
, and func_get_args()
functions within the function body.
Here’s how you can create a function with variable arguments in PHP:
function sum() {
$total = 0;
$numArgs = func_num_args();
for ($i = 0; $i < $numArgs; $i++) {
$total += func_get_arg($i);
}
return $total;
}
echo sum(1, 2, 3, 4); // Output: 10
In the example above, we define a function sum
that takes a variable number of arguments. Inside the function, we use func_num_args()
to get the number of arguments passed to the function and func_get_arg($i)
to get the value of each argument based on its position.
Alternatively, you can use the func_get_args()
function, which returns an array containing all the function arguments. Here’s how you can use func_get_args()
:
function sum() {
$total = 0;
$args = func_get_args();
foreach ($args as $arg) {
$total += $arg;
}
return $total;
}
echo sum(1, 2, 3, 4); // Output: 10
Using variable arguments is beneficial when you want to create a flexible function that can handle different numbers of arguments without having to specify each possible combination separately. However, keep in mind that using variable arguments can make the function less predictable, so use them judiciously and ensure proper error handling for different argument scenarios.