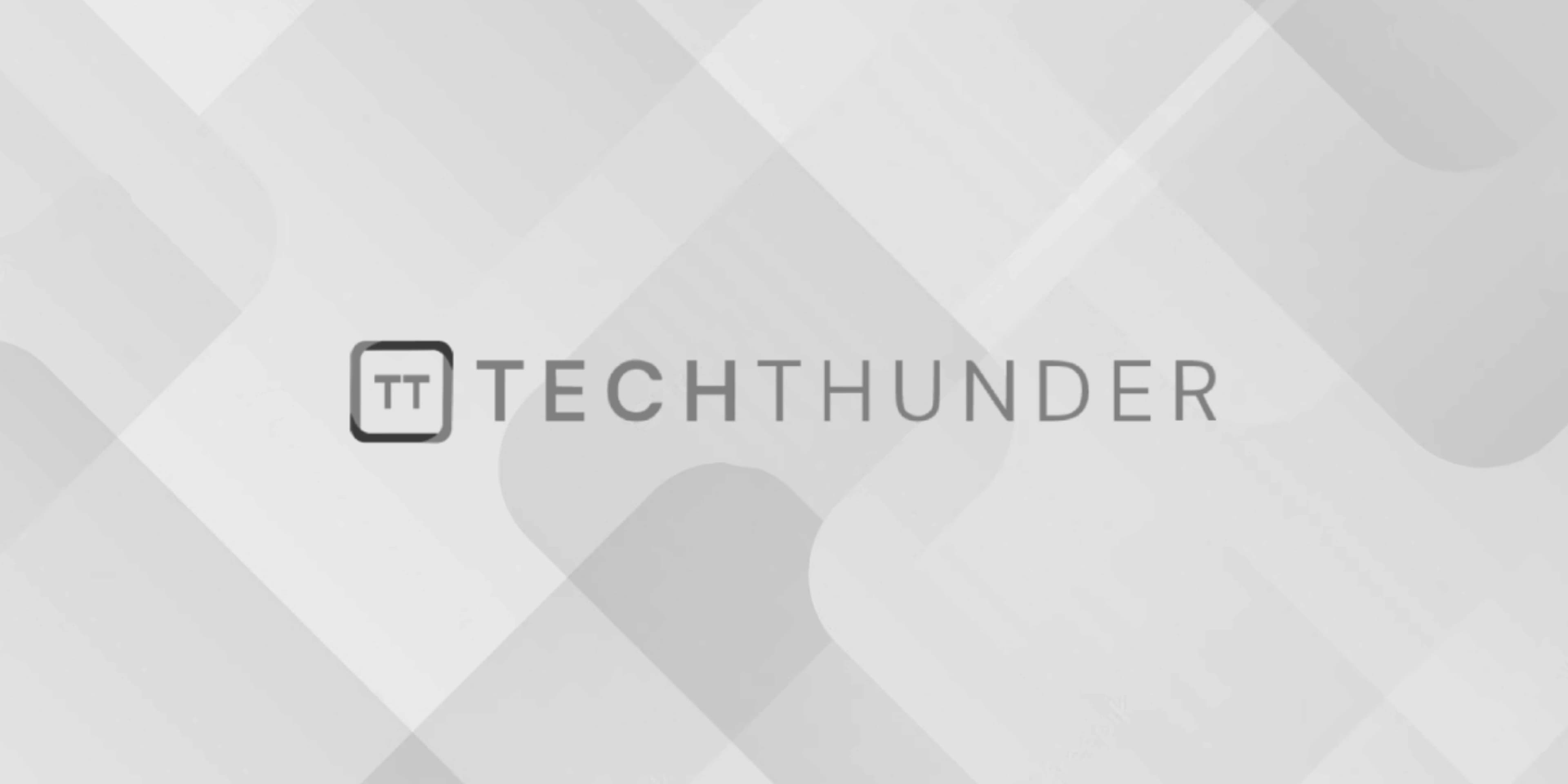
Remove Last Element from Array in PHP
To remove the last element from an array in PHP, you can use the array_pop()
function. array_pop()
removes the last element from the array and returns its value. The array keys will be reindexed automatically after removing the last element.
Here’s how you can use array_pop()
to remove the last element from an array:
$fruits = array("apple", "banana", "orange", "kiwi");
$removedElement = array_pop($fruits);
// Output the modified array
print_r($fruits);
// Output the removed element
echo "Removed Element: " . $removedElement;
Output:
Array
(
[0] => apple
[1] => banana
[2] => orange
)
Removed Element: kiwi
In the example above, we have an array $fruits
containing four elements. We use array_pop($fruits)
to remove the last element (“kiwi”) from the array. After removing the element, the array keys are reindexed, and the resulting array contains the remaining elements (“apple”, “banana”, and “orange”).
Just like array_shift()
, using array_pop()
modifies the original array by removing its last element. If you want to keep the original array intact while creating a new array without the last element, you can use array slicing with array_slice()
:
$fruits = array("apple", "banana", "orange", "kiwi");
$modifiedArray = array_slice($fruits, 0, -1);
// Output the modified array
print_r($modifiedArray);
// Output the original array remains unchanged
print_r($fruits);
Output:
Array
(
[0] => apple
[1] => banana
[2] => orange
)
Array
(
[0] => apple
[1] => banana
[2] => orange
[3] => kiwi
)
In this case, $modifiedArray
contains the elements from the beginning to the second-to-last element of the original $fruits
array, effectively excluding the last element. The original array $fruits
remains unchanged.