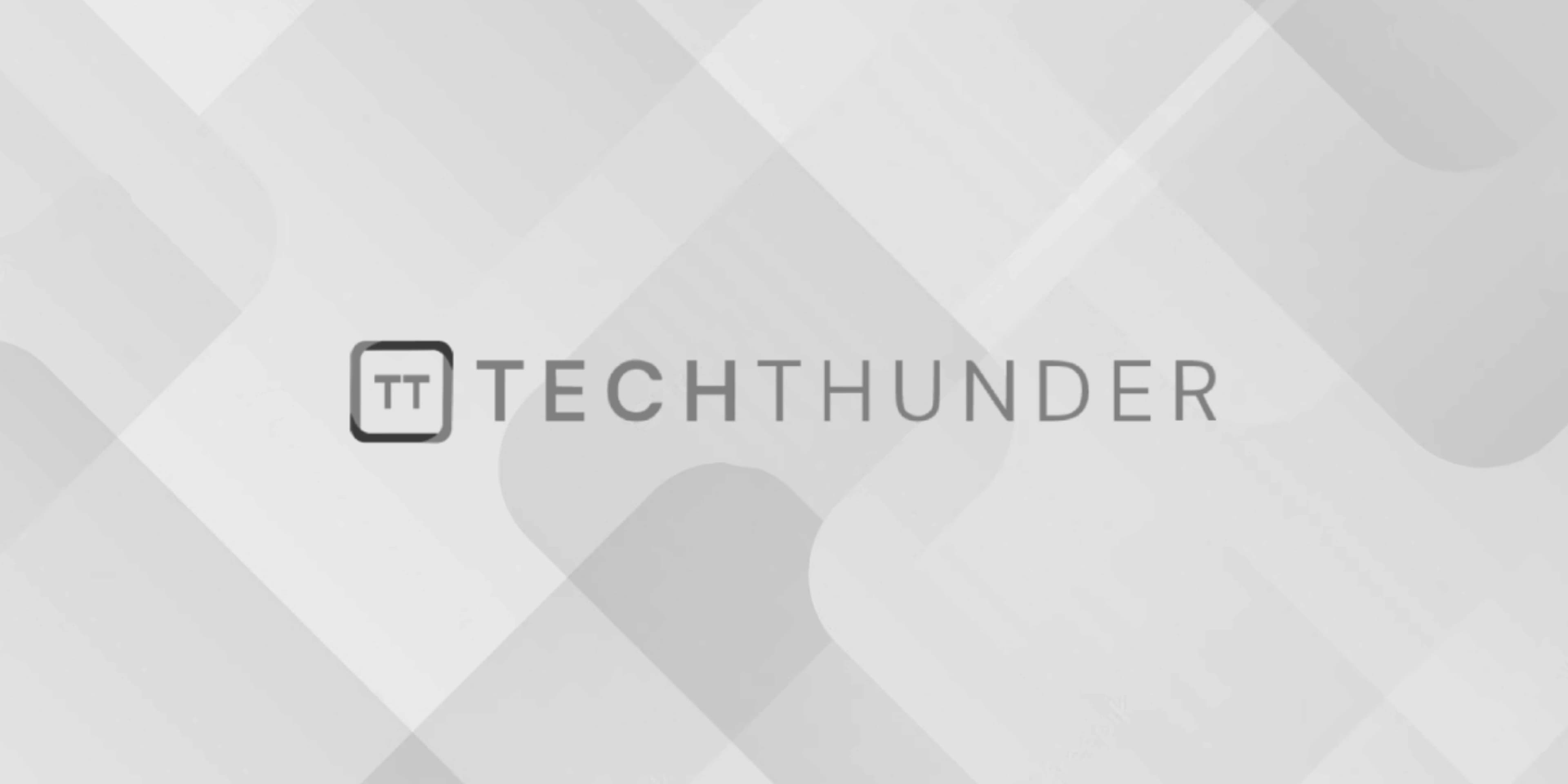
177 views
break vs continue in PHP
In PHP, both break
and continue
are control flow statements used within loops (like for
, while
, and do-while
) to alter the flow of execution based on certain conditions.
break
statement:
Thebreak
statement is used to exit the current loop prematurely. When PHP encounters thebreak
statement within a loop, it immediately terminates the loop, and the program execution continues with the code after the loop. This is useful when you want to stop the loop early based on a particular condition.
Example:
for ($i = 1; $i <= 10; $i++) {
if ($i === 5) {
break; // Exit the loop when $i equals 5
}
echo $i . ' ';
}
Output:
1 2 3 4
continue
statement:
Thecontinue
statement is used to skip the current iteration of the loop and move on to the next iteration. When PHP encounters thecontinue
statement within a loop, it skips the remaining code in the current iteration and jumps to the next iteration. This is useful when you want to skip specific elements in an array or perform conditional processing for certain values.
Example:
for ($i = 1; $i <= 10; $i++) {
if ($i % 2 === 0) {
continue; // Skip even numbers
}
echo $i . ' ';
}
Output:
1 3 5 7 9
In this example, the continue
statement is used to skip the even numbers (2, 4, 6, 8, 10) in the loop, and only odd numbers are displayed.
Both break
and continue
statements are powerful tools for controlling the flow of loops and can be used to improve the efficiency and readability of your code. However, it’s essential to use them judiciously and ensure that they are used in a way that makes the logic of your code clear and easy to understand.