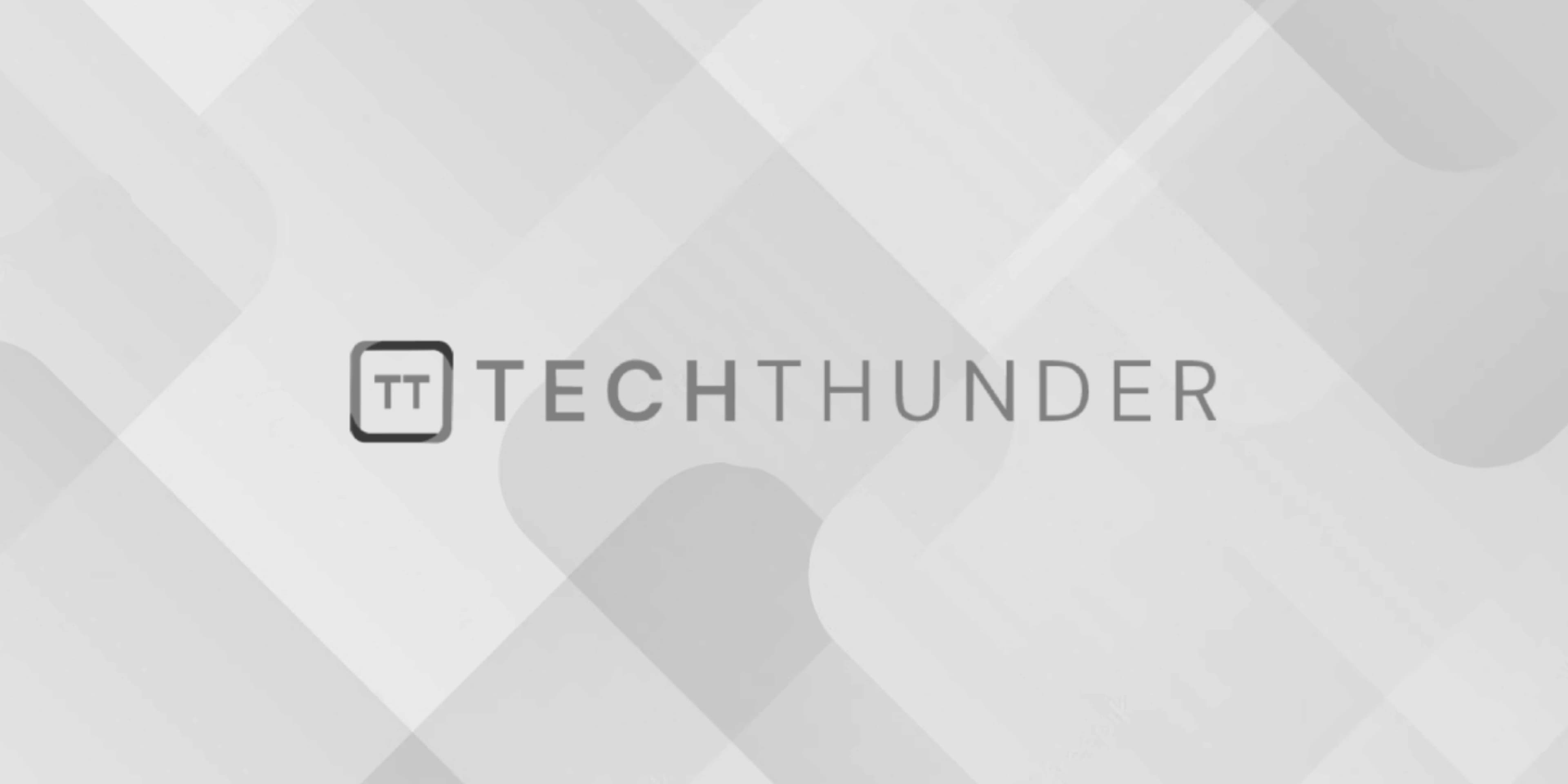
PHP PDO
PDO stands for “PHP Data Objects,” and it is a database access abstraction layer in PHP. It provides a consistent and secure way to interact with databases using a unified interface regardless of the database system being used (e.g., MySQL, PostgreSQL, SQLite, etc.). PDO is an alternative to using the traditional PHP database extension functions like MySQLi or MySQL functions, and it offers several advantages, including:
- Database Abstraction: PDO allows developers to write database-independent code. The same code can be used with different database systems without the need for significant changes.
- Security: PDO supports prepared statements, which help prevent SQL injection attacks. Prepared statements allow parameterized queries, where user input is treated as data and not executable code.
- Error Handling: PDO provides consistent and detailed error handling, making it easier to debug database-related issues.
- Transactions: PDO supports transactions, which allow a group of database operations to be treated as a single unit. Transactions ensure data integrity and consistency.
- Named Placeholders: PDO supports named placeholders for binding parameters in prepared statements, making code more readable and maintainable.
Here’s a basic example of using PDO to connect to a MySQL database and perform a simple query:
// Database configuration
$host = 'localhost';
$dbname = 'mydatabase';
$username = 'db_user';
$password = 'db_password';
try {
// Connect to the database using PDO
$pdo = new PDO("mysql:host=$host;dbname=$dbname", $username, $password);
// Set error handling to throw exceptions on error
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
// Perform a simple query
$stmt = $pdo->query("SELECT * FROM users");
$users = $stmt->fetchAll(PDO::FETCH_ASSOC);
// Loop through the results
foreach ($users as $user) {
echo "User ID: " . $user['id'] . ", Name: " . $user['name'] . "<br>";
}
} catch (PDOException $e) {
echo "Error: " . $e->getMessage();
}
In this example, we use PDO to connect to a MySQL database, fetch all rows from the “users” table, and display the user IDs and names. The use of prepared statements and error handling enhances the security and robustness of the code.
PDO is a versatile and powerful tool for database interactions in PHP, and it is recommended to use it over the older database extensions whenever possible.