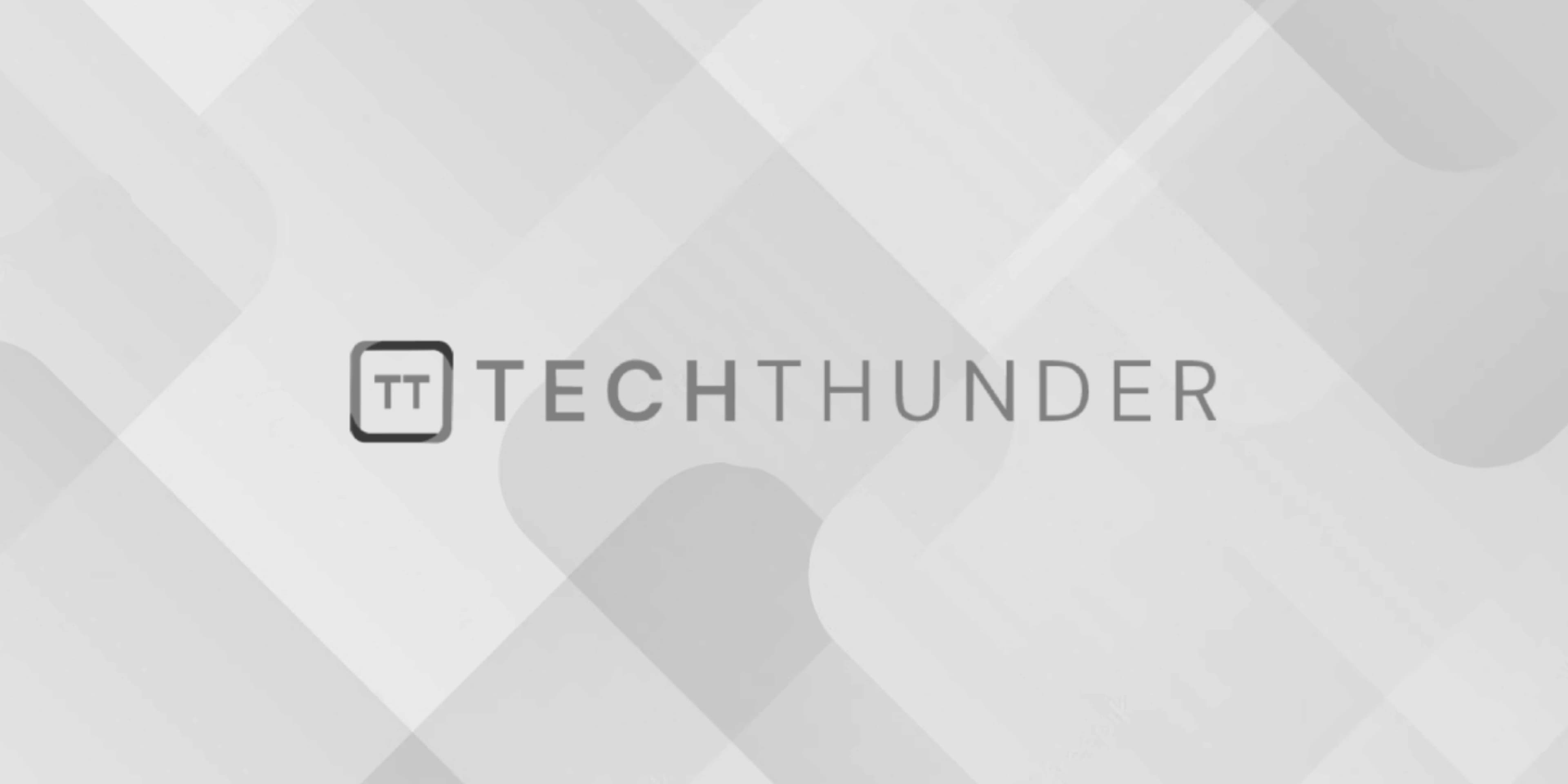
PHP Regular Expressions
In PHP, regular expressions (regex or regexp) are powerful tools used for pattern matching and manipulating strings. They provide a flexible and concise way to search, extract, and replace specific patterns within strings. PHP provides built-in functions that support regular expressions for performing various operations on strings.
Basic Syntax:
In PHP, you use regular expressions with pattern delimiters (usually /
) to define the pattern you want to match. The basic syntax to perform regular expression operations is as follows:
preg_match($pattern, $subject, $matches);
preg_match_all($pattern, $subject, $matches);
preg_replace($pattern, $replacement, $subject);
preg_split($pattern, $subject);
Here’s a brief explanation of each function:
preg_match($pattern, $subject, $matches)
: Searches the subject string for the first occurrence of the pattern and returns true if a match is found. If a match is found, the matched parts are stored in the$matches
array.preg_match_all($pattern, $subject, $matches)
: Searches the subject string for all occurrences of the pattern and returns the number of matches. The matched parts are stored in the$matches
multidimensional array.preg_replace($pattern, $replacement, $subject)
: Searches the subject string for matches to the pattern and replaces them with the specified replacement string.preg_split($pattern, $subject)
: Splits the subject string into an array of substrings based on the occurrences of the pattern.
Regex Patterns:
Regex patterns consist of a combination of characters and special metacharacters that define the rules for matching specific patterns. For example:
/abc/
will match the exact substring “abc”./[0-9]+/
will match one or more consecutive digits.
Common Regex Metacharacters:
.
: Matches any single character except a newline.*
: Matches zero or more occurrences of the preceding character or group.+
: Matches one or more occurrences of the preceding character or group.?
: Matches zero or one occurrence of the preceding character or group.^
: Matches the start of a string.$
: Matches the end of a string.[]
: Matches any character within the brackets.|
: Acts like an OR operator, matches either the expression before or after it.
Example:
// Using preg_match to find a pattern in a string
$subject = "Hello, my name is John.";
$pattern = "/John/";
if (preg_match($pattern, $subject, $matches)) {
echo "Match found: " . $matches[0];
} else {
echo "No match found.";
}
Output: Match found: John
Regex can be quite powerful, but it may also be complex and difficult to read for those unfamiliar with its syntax. Regular expressions are often used for tasks such as validating input, extracting data, and manipulating strings in a more flexible and efficient way. To learn more about regular expressions and explore different patterns, you can refer to the official PHP documentation on regular expressions or use online regex tools for testing and experimenting with patterns.