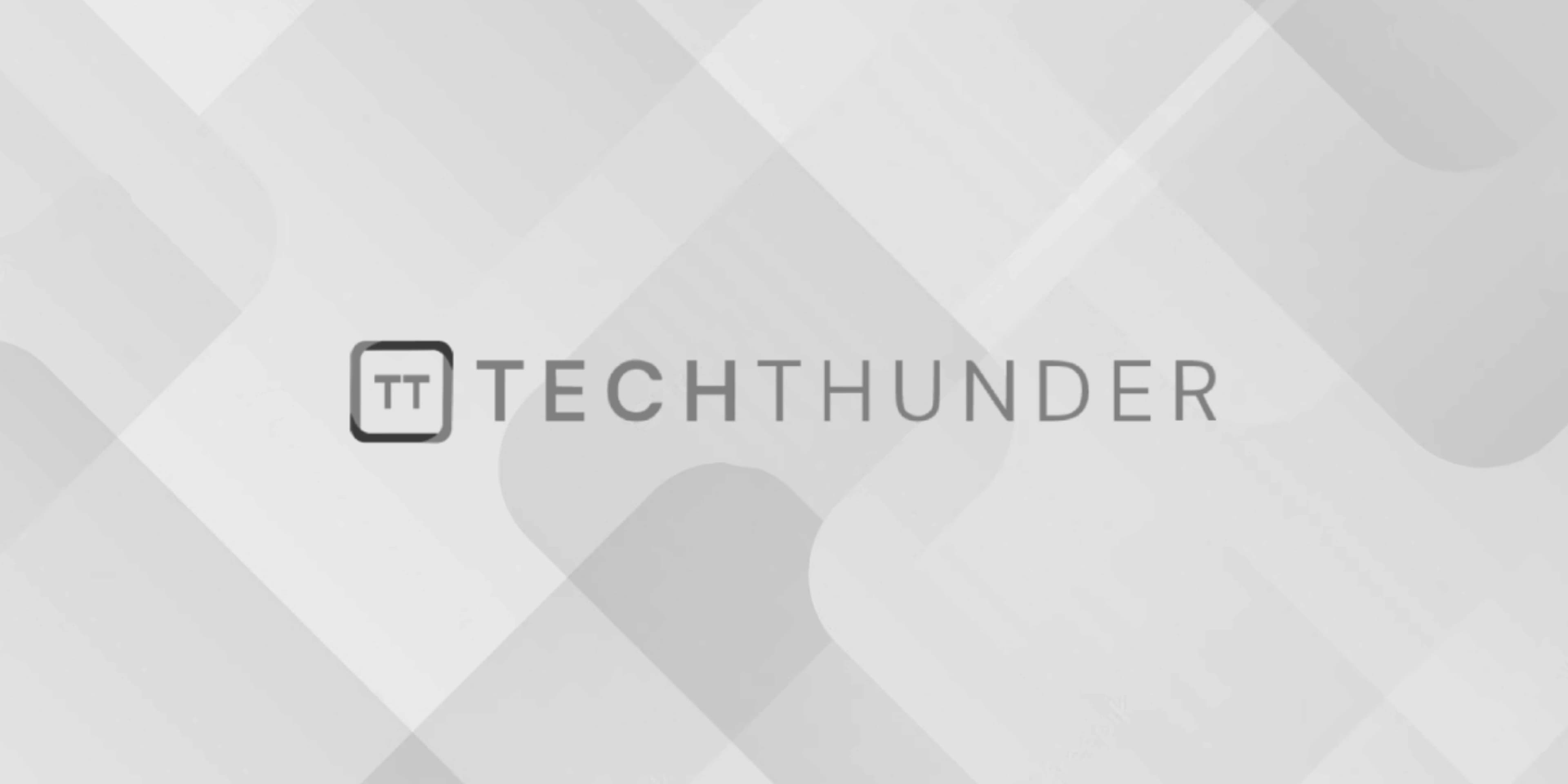
OOPs Abstract Class
In object-oriented programming (OOP), an abstract class is a class that cannot be instantiated on its own but serves as a blueprint for other classes. It acts as a base class that provides common attributes and methods that its derived classes can inherit. An abstract class may contain abstract methods, which are methods without an implementation. The responsibility of implementing these abstract methods lies with the derived classes.
An abstract class is often used to establish a common interface or contract for a group of related classes. It ensures that all derived classes provide specific functionality while allowing each class to implement that functionality in its unique way.
In many programming languages that support OOP, including Java, C#, and TypeScript, you can define an abstract class using the abstract
keyword. Here’s an example in TypeScript:
abstract class Shape {
abstract getArea(): number;
abstract getPerimeter(): number;
}
In this example, the Shape
class is defined as an abstract class using the abstract
keyword. It contains two abstract methods: getArea()
and getPerimeter()
. Any class that extends the Shape
class must implement these methods.
To implement an abstract class, you use the extends
keyword, and you must provide concrete implementations of all abstract methods. Here’s an example:
class Rectangle extends Shape {
private width: number;
private height: number;
constructor(width: number, height: number) {
super();
this.width = width;
this.height = height;
}
getArea(): number {
return this.width * this.height;
}
getPerimeter(): number {
return 2 * (this.width + this.height);
}
}
In this example, the Rectangle
class extends the Shape
abstract class and provides concrete implementations for the getArea()
and getPerimeter()
methods.
It’s important to note that you cannot create an instance of an abstract class directly. For example, the following will result in a compilation error:
const shape = new Shape(); // Error: Cannot create an instance of an abstract class.
Abstract classes are useful when you want to define a common structure and interface for a group of related classes but don’t want to instantiate the base class itself. They help enforce design contracts and promote code reuse by providing a consistent interface for a group of classes to implement.