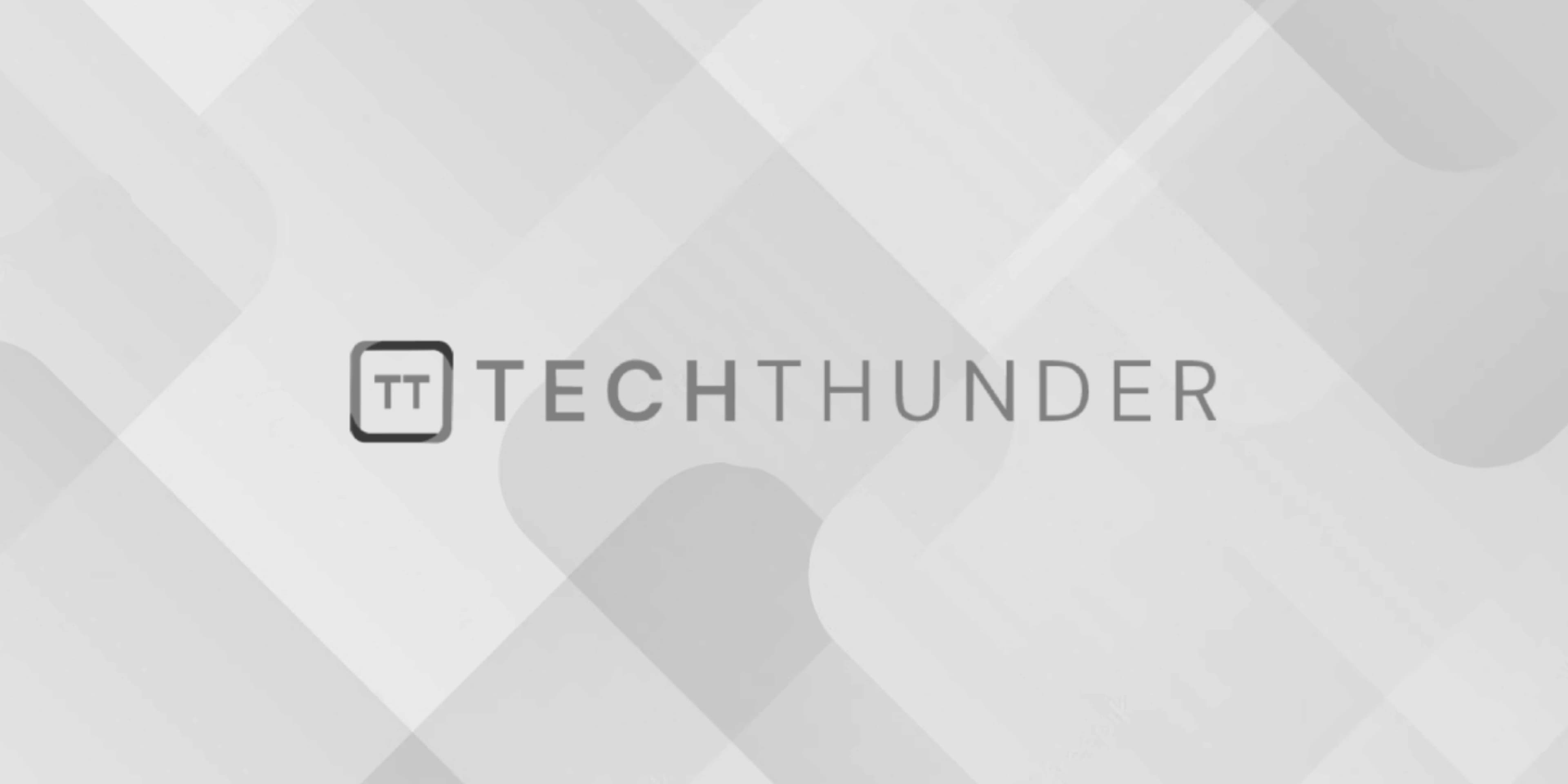
PHP is_null() Function
In PHP, the is_null()
function is used to determine whether a variable is null
or not. It returns a boolean value (true
or false
) depending on whether the variable is null
.
Here’s the syntax of the is_null()
function:
bool is_null ( mixed $variable )
Parameters:
$variable
: The variable that you want to check fornull
value.
Return Value:
- The function returns
true
if the$variable
isnull
, andfalse
otherwise.
Example:
<?php
$var1 = null;
$var2 = 10;
// Check if $var1 is null
if (is_null($var1)) {
echo "\$var1 is null.\n";
} else {
echo "\$var1 is not null.\n";
}
// Check if $var2 is null
if (is_null($var2)) {
echo "\$var2 is null.\n";
} else {
echo "\$var2 is not null.\n";
}
?>
Output:
$var1 is null.
$var2 is not null.
In the example above, we have two variables, $var1
and $var2
. The first variable $var1
is explicitly set to null
, while the second variable $var2
contains the value 10
.
The is_null()
function is used to check whether each variable is null
or not. In the output, we can see that $var1
is null
, and $var2
is not null
.
The is_null()
function is useful when you need to validate if a variable is set to null
before performing certain operations. It helps to handle cases where a variable may or may not have a value assigned to it.