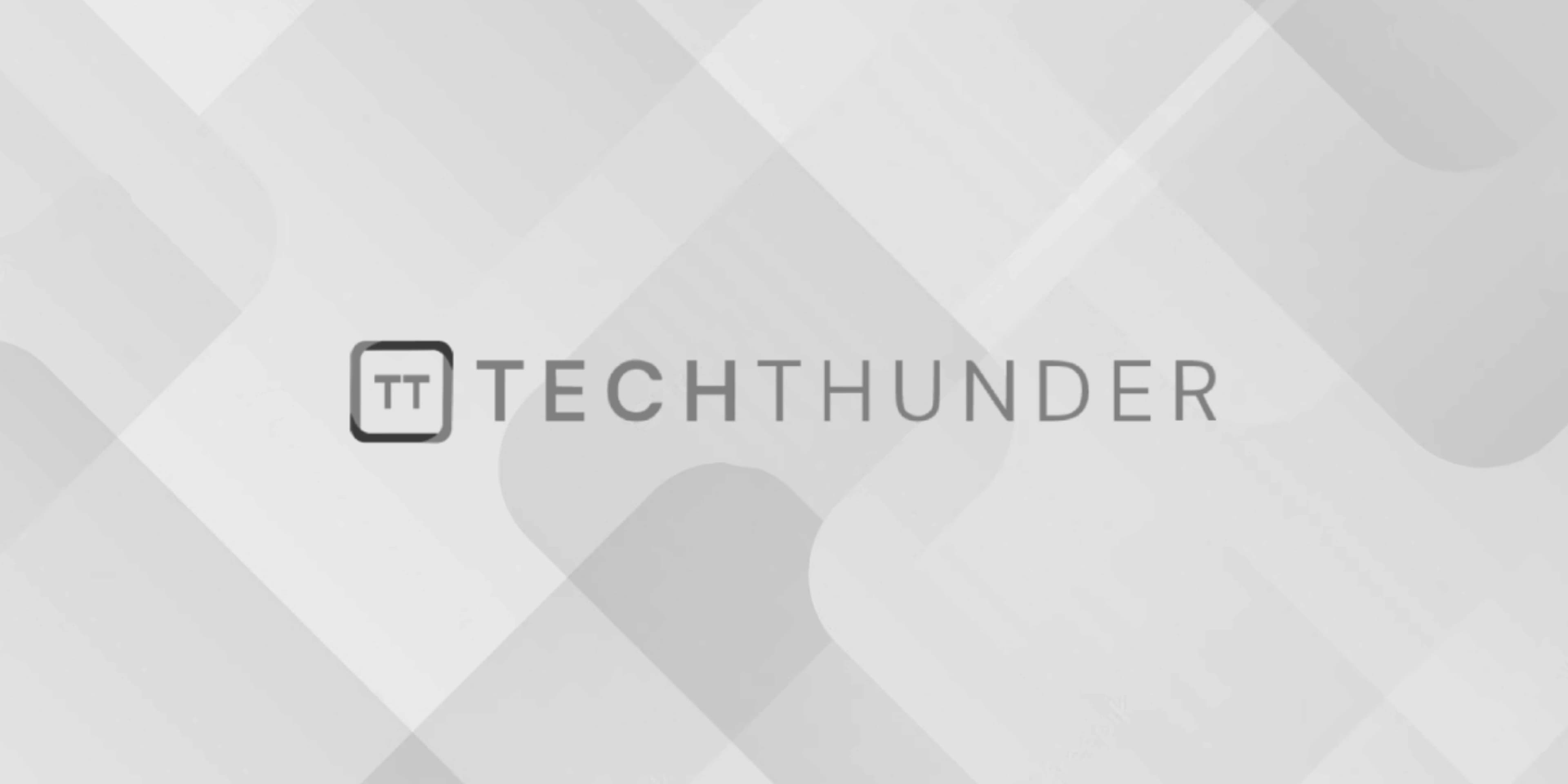
OOPs Destructor
In object-oriented programming (OOP), a destructor is a special method defined within a class that is automatically called when an object is destroyed or goes out of scope. The destructor is responsible for performing cleanup tasks, releasing resources, or any other necessary actions before the object is removed from memory.
In PHP, the destructor method is named __destruct()
(double underscore before “destruct”). When an object is no longer referenced or when the script ends, PHP automatically calls the __destruct()
method for that object, if it is defined within the class.
Here’s the syntax for defining a destructor in PHP:
class ClassName {
public function __destruct() {
// Destructor code here
}
}
Example:
class Example {
public function __construct() {
echo "Object created.\n";
}
public function __destruct() {
echo "Object destroyed.\n";
}
}
// Creating an object of the class calls the constructor
$object = new Example(); // Output: Object created.
// The destructor is automatically called when the object goes out of scope or script ends
unset($object); // Output: Object destroyed.
In the example above, we defined an Example
class with both a constructor and a destructor. When an object of the class is created, the constructor is automatically called, and when the object goes out of scope (using unset()
or when the script ends), the destructor is automatically called.
Destructors are useful for performing cleanup tasks, such as closing database connections, releasing file handles, or freeing up other resources used by the object. They help ensure that resources are properly released and that any necessary cleanup is performed, preventing potential memory leaks or resource leaks.
It’s important to note that PHP automatically manages memory and resources, so in most cases, you don’t need to explicitly call the destructor. The destructor is invoked automatically when the object is no longer needed or when the script execution ends. However, if you want to explicitly destroy an object and release its resources before it goes out of scope, you can use the unset()
function, as shown in the example.
Keep in mind that PHP’s garbage collector handles memory management, so you generally don’t need to worry about manually freeing memory or deallocating resources. Just make sure to properly clean up any resources in the destructor, and PHP will take care of the rest.