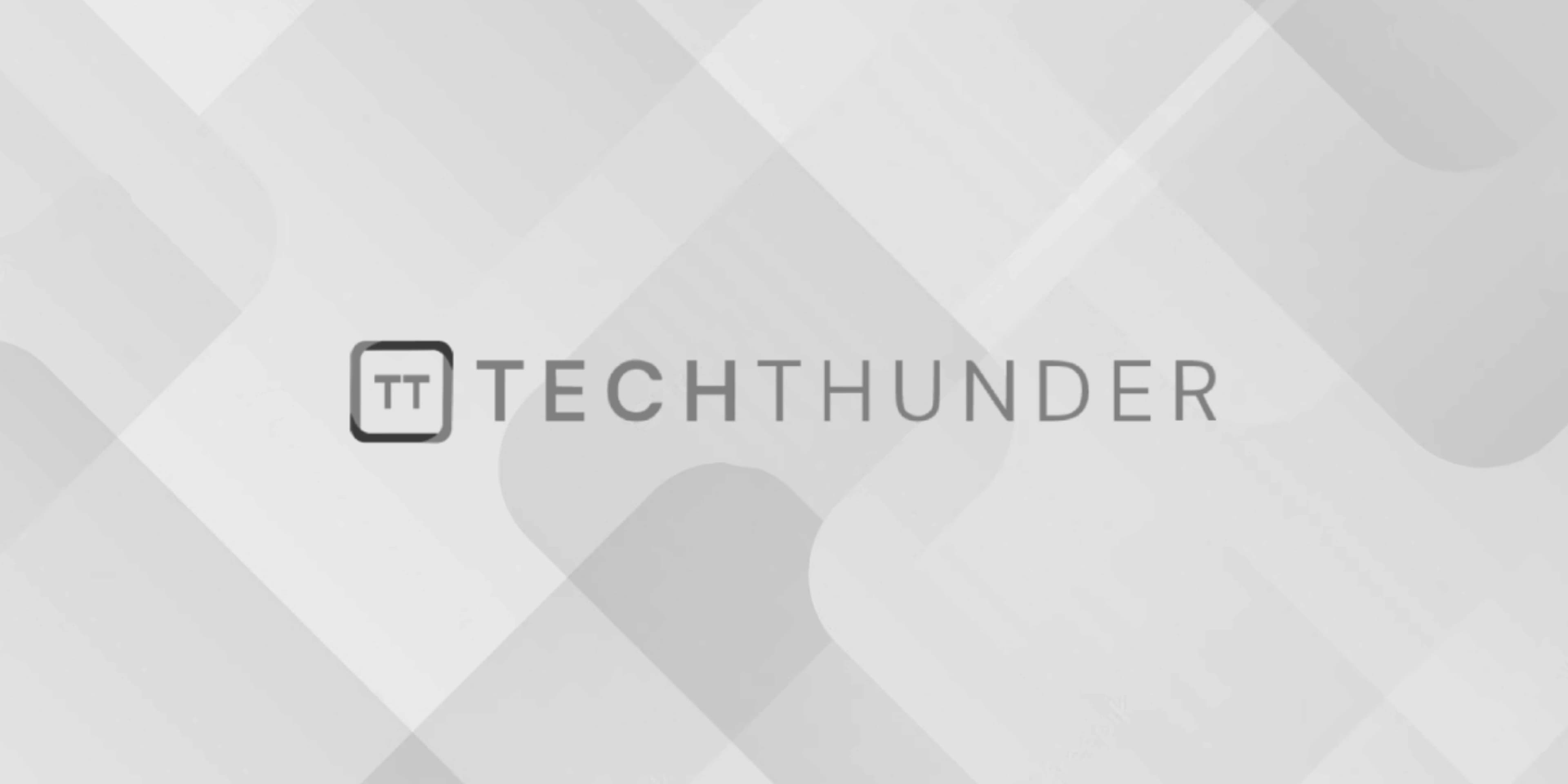
fpassthru() Function in PHP
In PHP, fpassthru()
is a function that is used to output the contents of an open file pointer (file handle) directly to the output buffer (usually the web browser) without loading the entire file into memory. It is particularly useful for streaming large files to the client, such as video files, audio files, or large downloads, without consuming excessive server memory.
Here’s the syntax of the fpassthru()
function:
int fpassthru(resource $handle)
Parameters:
$handle
: A file pointer (resource) obtained by opening a file using functions likefopen()
.
Return value:
- The function returns the number of bytes read from the file and sent to the output buffer. On failure, it returns
false
.
Example:
Let’s say we have a file named “largefile.zip” that we want to stream to the client without loading the entire file into memory. We can use fpassthru()
for this purpose:
// Open the file in binary read mode
$file = fopen('largefile.zip', 'rb');
// Check if the file was opened successfully
if ($file) {
// Set the appropriate headers for streaming the file
header('Content-Type: application/zip');
header('Content-Disposition: attachment; filename="largefile.zip"');
// Output the file contents to the browser
fpassthru($file);
// Close the file pointer
fclose($file);
exit;
} else {
// Handle file opening error
echo 'Error opening the file.';
}
In this example, we open the file “largefile.zip” in binary read mode and then set appropriate HTTP headers to indicate that the file being streamed is a ZIP file and should be downloaded with the name “largefile.zip”. We then use fpassthru()
to output the contents of the file directly to the browser.
It’s important to note that when using fpassthru()
, no further output should be sent to the browser after calling this function because it will send the contents of the entire file to the output buffer, and any subsequent output might corrupt the file being streamed. That’s why we use exit
after calling fpassthru()
to terminate the script execution.
Overall, fpassthru()
is a useful function for efficiently streaming large files to the client without overloading the server’s memory.