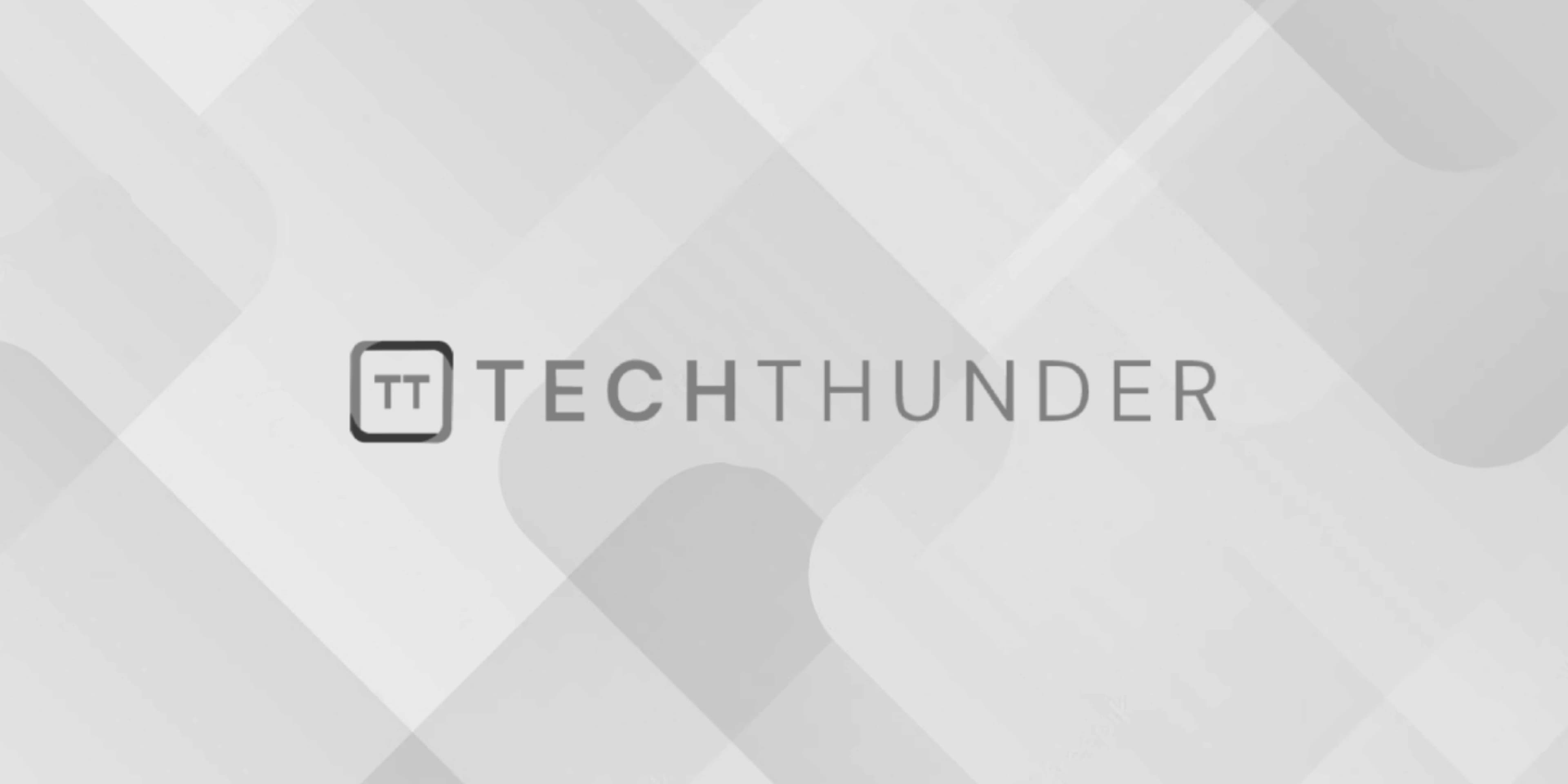
PHP MySQLi CONNECT
In PHP, you can use MySQLi (MySQL Improved) to connect to a MySQL database and perform various database operations. MySQLi provides an improved API for interacting with MySQL databases compared to the older MySQL extension, which has been deprecated.
Here’s how you can connect to a MySQL database using MySQLi in PHP:
- Connect to the MySQL Database:
To establish a connection to the MySQL database, use themysqli_connect()
function. This function takes the following parameters:
- Hostname: The server name or IP address where the MySQL database is running. Use
"localhost"
if the database is on the same server as your PHP code. - Username: The username to authenticate with the database server.
- Password: The password for the database user.
- Database Name: The name of the MySQL database you want to connect to.
<?php
$servername = "localhost"; // Replace with your server name or IP address
$username = "your_username"; // Replace with your database username
$password = "your_password"; // Replace with your database password
$dbname = "your_database"; // Replace with your database name
// Create a connection
$conn = mysqli_connect($servername, $username, $password, $dbname);
// Check the connection
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
// If the connection is successful, you can perform database operations here.
// For example, execute SQL queries, insert data, update records, etc.
// Don't forget to close the connection when you are done.
mysqli_close($conn);
?>
- Check the Connection Status:
After creating the connection, you should check whether the connection was successful or not. If the connection fails, the script will terminate with an error message. - Perform Database Operations:
Once the connection is established, you can use the$conn
variable to perform various database operations like executing SQL queries, inserting data, updating records, deleting data, and more. - Close the Database Connection:
After performing the required database operations, it’s essential to close the database connection using themysqli_close()
function to release the resources and avoid potential issues.
Remember to replace "your_username"
, "your_password"
, and "your_database"
with your actual database credentials and database name. Also, ensure that the provided credentials have sufficient privileges to access the specified database.
Using MySQLi provides advantages such as support for prepared statements, object-oriented style, improved error handling, and better security compared to the deprecated MySQL extension. It is recommended to use MySQLi or PDO (PHP Data Objects) for database interactions in PHP.