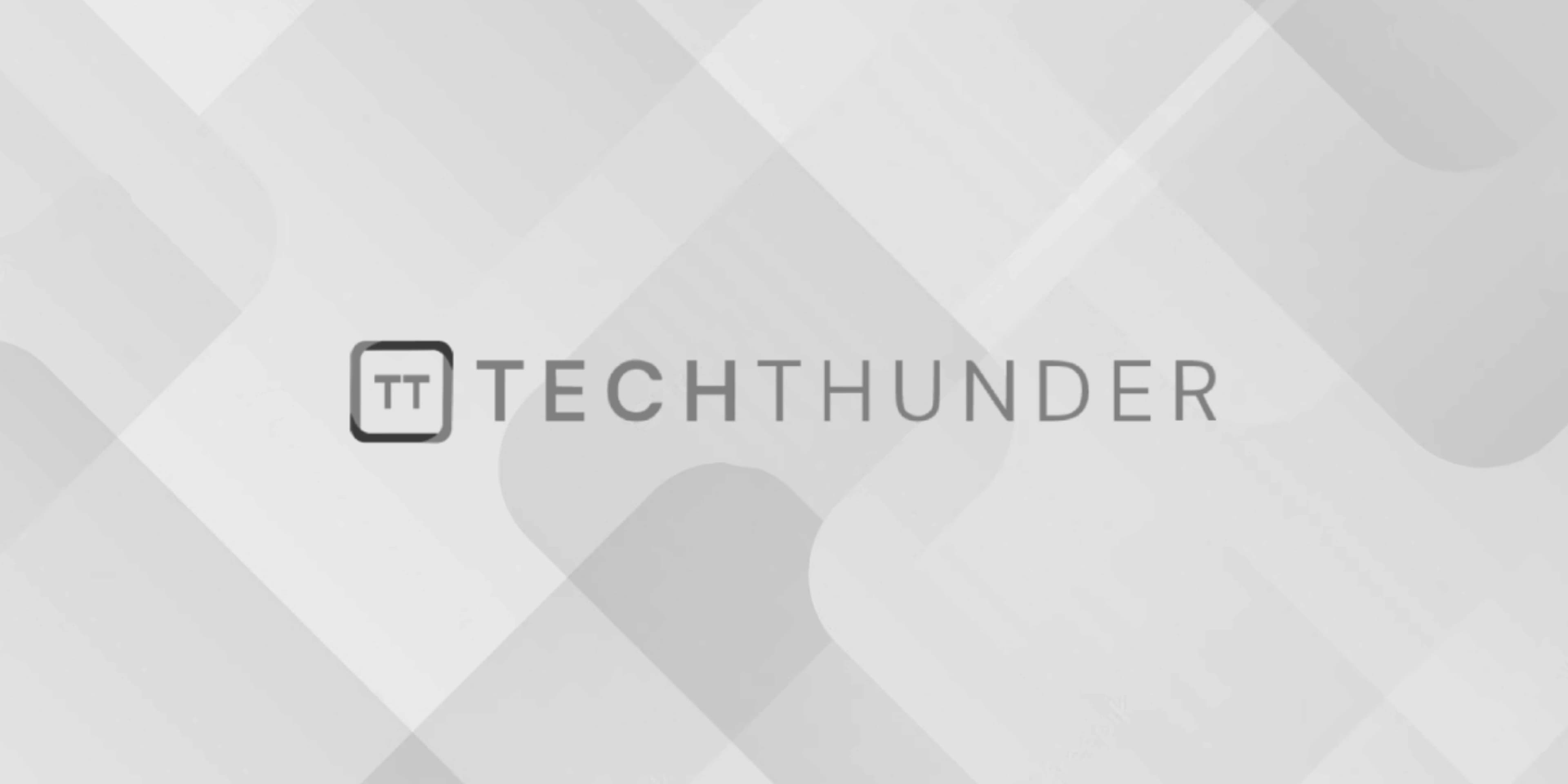
PHP gmp_random_range() function
As of my last update in September 2021, PHP does not have a built-in gmp_random_range()
function in the GMP (GNU Multiple Precision) extension. However, you can achieve the functionality of generating random numbers within a specified range using other GMP functions or PHP’s core random number functions.
One common approach is to use the gmp_random_bits()
function to generate a random number with a certain number of bits and then use other GMP functions to adjust the number to the desired range.
Here’s an example of how you can generate a random number within a specific range using GMP functions:
function gmp_random_range($min, $max) {
$range = gmp_sub($max, $min);
$bits = gmp_intval(gmp_add(gmp_nextprime($range), 1));
do {
$randomNumber = gmp_random_bits($bits);
} while (gmp_cmp($randomNumber, $range) > 0);
return gmp_add($min, $randomNumber);
}
$min = 100;
$max = 200;
$randomNumber = gmp_random_range($min, $max);
echo gmp_strval($randomNumber); // Output: Random number between 100 and 200
In this example, the gmp_random_range()
function generates a random number between the given $min
and $max
values. The function calculates the range between $min
and $max
, generates a random number within that range using gmp_random_bits()
, and then adds the generated random number to $min
to obtain a random number within the desired range.
Keep in mind that this is just one way to implement the functionality, and you can use different strategies based on your specific requirements.
If you don’t specifically need to use GMP functions, you can also use PHP’s core random number functions like rand()
or random_int()
to generate random numbers within a range. For cryptographic purposes, random_int()
is recommended as it provides cryptographically secure random numbers.