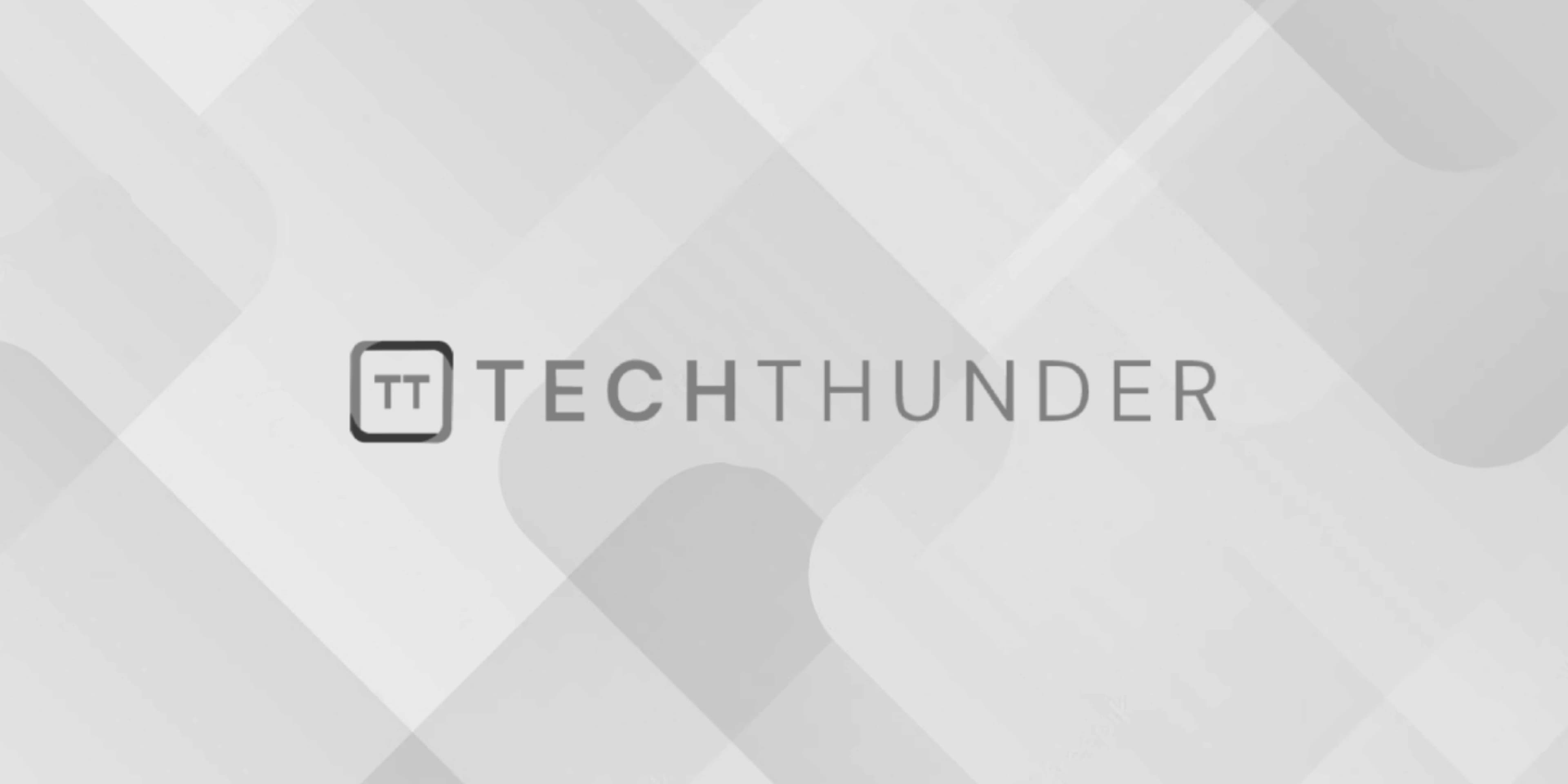
PHP Multidimensional Array Search By Value
To search for a value in a multidimensional array in PHP, you can use a recursive function that iterates through each element of the array, checking for the desired value. Here’s a PHP function that performs a search by value in a multidimensional array:
function searchInMultidimensionalArray($array, $searchValue) {
foreach ($array as $key => $value) {
if ($value === $searchValue) {
return true; // Found the value
} elseif (is_array($value)) {
// Recursively search within nested arrays
if (searchInMultidimensionalArray($value, $searchValue)) {
return true; // Found the value in nested array
}
}
}
return false; // Value not found in the array
}
Usage:
Suppose you have a multidimensional array like this:
$myArray = array(
'a' => array(1, 2, 3),
'b' => array('x', 'y', 'z'),
'c' => array('p', 'q', 'r')
);
$searchValue = 'q';
if (searchInMultidimensionalArray($myArray, $searchValue)) {
echo "Value '$searchValue' found in the array.";
} else {
echo "Value '$searchValue' not found in the array.";
}
In this example, the searchInMultidimensionalArray()
function will recursively search through the nested arrays to find the value 'q'
. If the value is found anywhere in the array, it will return true
, and if the value is not found, it will return false
.
Remember that this function performs a deep search through the entire multidimensional array, so it may not be efficient for large arrays. If you are dealing with large datasets, consider using more efficient search algorithms or data structures, such as hash tables or databases, to optimize the search process.