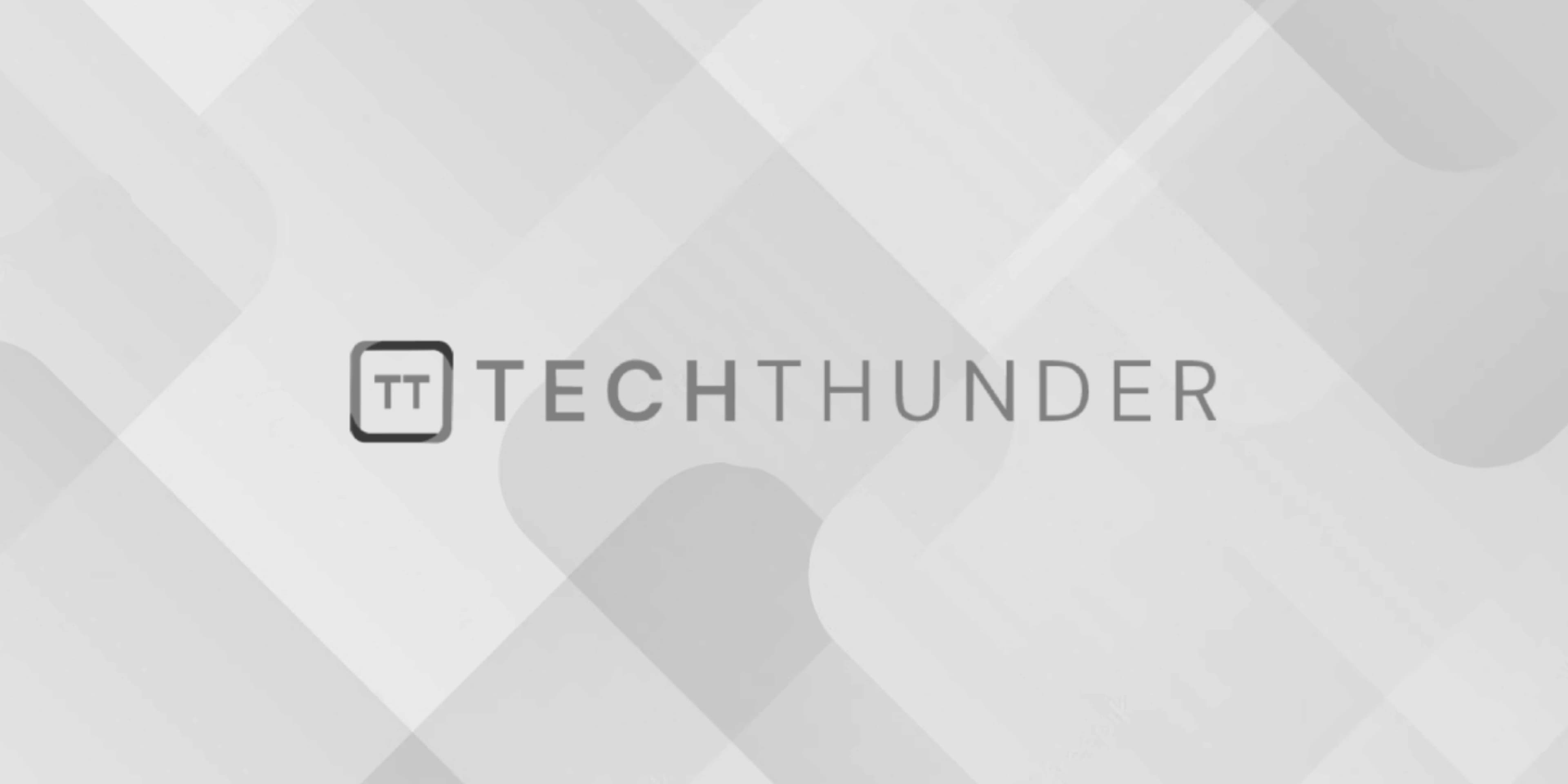
OOPs Inheritance
In object-oriented programming (OOP), inheritance is a fundamental concept that allows one class (called the subclass or derived class) to inherit the properties and behaviors of another class (called the superclass or base class). Inheritance facilitates code reuse and the creation of a hierarchical relationship between classes, allowing new classes to be built upon existing ones.
The subclass inherits the attributes (properties) and methods (functions) of the superclass, and it can also define additional attributes and methods or override existing ones. This enables you to create a more specialized version of a class while maintaining the common functionalities of the base class.
Inheritance is typically represented as an “is-a” relationship, meaning a subclass “is a” type of its superclass. For example, if you have a class Vehicle
, you can create subclasses like Car
, Bike
, and Truck
, which are all types of vehicles.
Syntax for defining inheritance in OOP:
class SuperClass {
// Properties and methods of the superclass
}
class SubClass extends SuperClass {
// Properties and methods of the subclass
}
Example:
class Animal {
protected $name;
public function __construct($name) {
$this->name = $name;
}
public function makeSound() {
echo "Animal makes a sound.\n";
}
}
class Dog extends Animal {
public function __construct($name) {
parent::__construct($name); // Call the parent class constructor
}
public function makeSound() {
echo "Dog barks.\n";
}
public function fetch() {
echo "Dog fetches the ball.\n";
}
}
$animal = new Animal("Generic Animal");
$dog = new Dog("Buddy");
$animal->makeSound(); // Output: Animal makes a sound.
$dog->makeSound(); // Output: Dog barks.
$dog->fetch(); // Output: Dog fetches the ball.
In the example above, we have a Animal
superclass and a Dog
subclass. The Dog
class extends the Animal
class using the extends
keyword. As a result, the Dog
class inherits the $name
property and the makeSound()
method from the Animal
class. The Dog
class also defines its own makeSound()
method and an additional method fetch()
.
When you create an object of the Dog
class, it has access to both its own methods (makeSound()
, fetch()
) and the methods inherited from the Animal
class (__construct()
and makeSound()
). The __construct()
method of the superclass is called using parent::__construct($name)
to set the name of the dog.
Inheritance allows you to create a class hierarchy, making your code more organized, modular, and easy to maintain. It promotes the principle of code reusability and enables you to create specialized classes while reusing common functionality defined in the base classes.