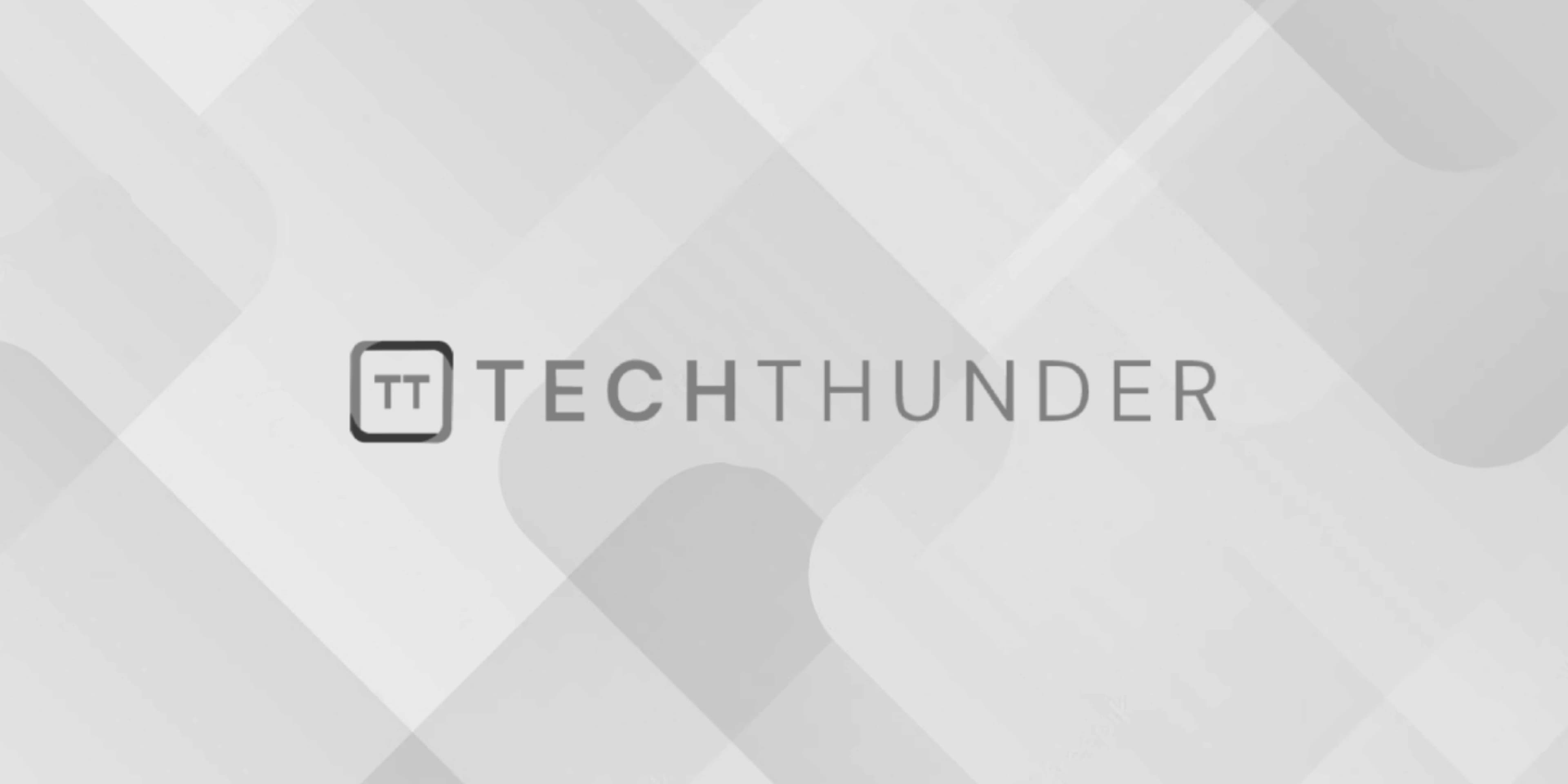
PHP Array
In PHP, an array is a data structure that can hold multiple values of different data types under a single variable name. Arrays are widely used in PHP for storing and organizing data, making it easier to work with collections of elements.
There are two types of arrays in PHP:
- Indexed Arrays:
An indexed array is a collection of elements where each element is identified by an index (numeric key) starting from 0. The elements are stored in a sequence, and you can access them using their index.
// Creating an indexed array
$fruits = array("Apple", "Banana", "Orange");
You can also use square brackets []
syntax for creating an indexed array in modern PHP versions:
$fruits = ["Apple", "Banana", "Orange"];
To access the elements of an indexed array, use their respective index:
echo $fruits[0]; // Output: Apple
echo $fruits[1]; // Output: Banana
echo $fruits[2]; // Output: Orange
- Associative Arrays:
An associative array is a collection of elements where each element is identified by a unique key (string or numeric). Unlike indexed arrays, the order of elements in an associative array does not matter; the elements are accessed using their keys.
// Creating an associative array
$person = array(
"name" => "John",
"age" => 30,
"city" => "New York"
);
In modern PHP versions, you can create an associative array using the square brackets syntax as well:
$person = [
"name" => "John",
"age" => 30,
"city" => "New York"
];
To access the elements of an associative array, use their respective keys:
echo $person["name"]; // Output: John
echo $person["age"]; // Output: 30
echo $person["city"]; // Output: New York
Arrays in PHP are versatile and support various functions and operations for manipulation, iteration, and data processing. You can add or remove elements, merge arrays, search for values, sort, filter, and perform many other operations on arrays. Always refer to the official PHP documentation for a comprehensive list of array functions and their usages.