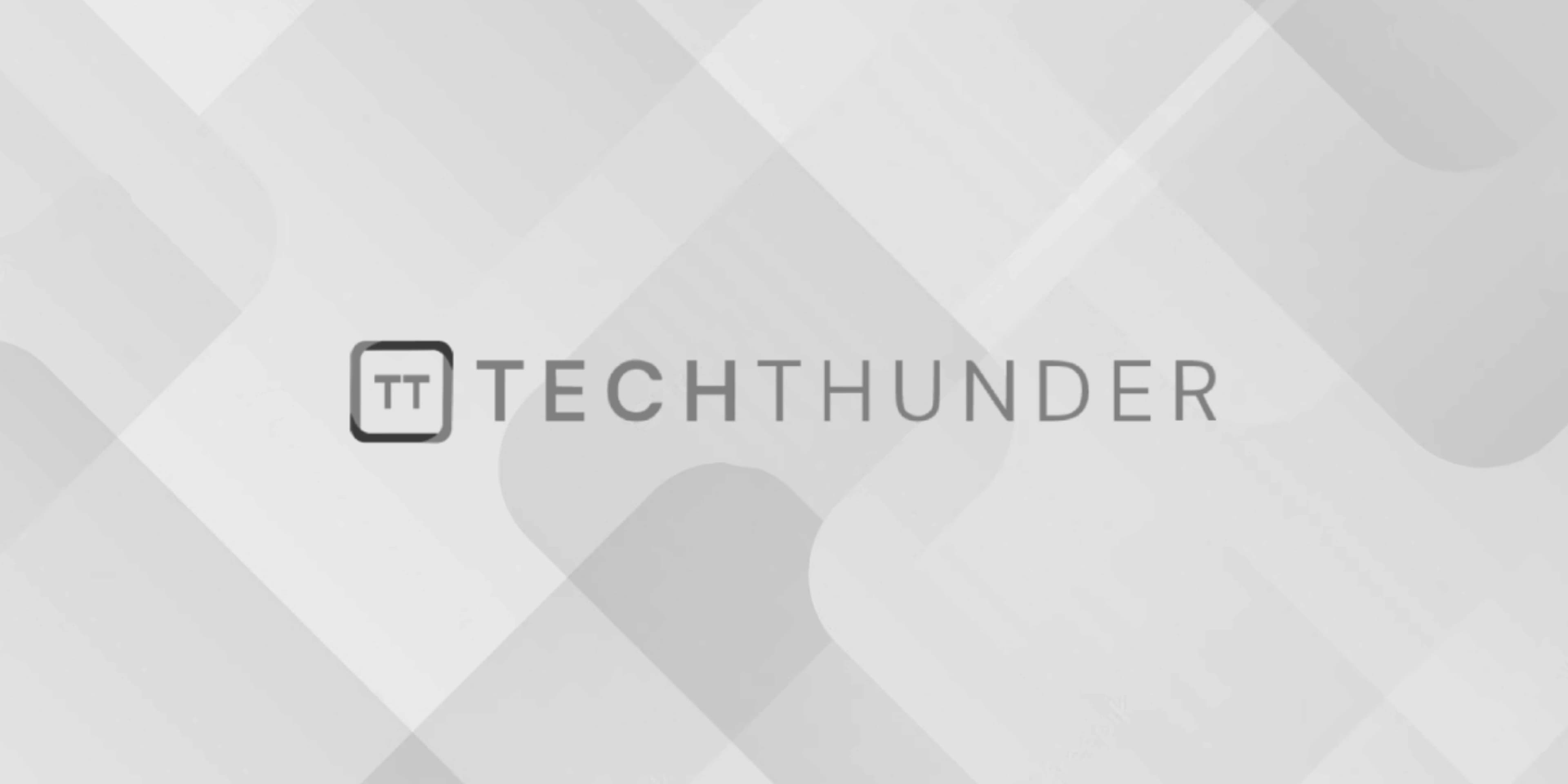
How to create html table with a while loop in PHP
To create an HTML table using a while loop in PHP, you first need to fetch data from a data source (e.g., a database) using the while loop. Then, you can use the fetched data to dynamically generate the table rows and cells. Here’s a step-by-step guide on how to achieve this:
Assuming you have data in an array or fetched from a database, here’s a basic example of how to create an HTML table using a while loop in PHP:
<?php
// Sample data (can be fetched from a database query)
$data = array(
array('Name' => 'John Doe', 'Age' => 30, 'Email' => '[email protected]'),
array('Name' => 'Jane Smith', 'Age' => 25, 'Email' => '[email protected]'),
array('Name' => 'Michael Johnson', 'Age' => 35, 'Email' => '[email protected]'),
);
// Start the HTML table
echo '<table border="1">';
echo '<tr><th>Name</th><th>Age</th><th>Email</th></tr>';
// Loop through the data and generate table rows and cells
foreach ($data as $row) {
echo '<tr>';
echo '<td>' . $row['Name'] . '</td>';
echo '<td>' . $row['Age'] . '</td>';
echo '<td>' . $row['Email'] . '</td>';
echo '</tr>';
}
// End the HTML table
echo '</table>';
?>
In this example, we have a sample $data
array with three records. The while loop is replaced by a foreach
loop to iterate through each row of data. Inside the loop, we use echo
statements to generate the table rows (<tr>
) and table cells (<td>
). The foreach
loop dynamically creates the rows and cells based on the data, resulting in a complete HTML table.
The output of this code will be an HTML table with three rows and three columns, displaying the Name, Age, and Email of each person from the sample data.
Note: In real-world scenarios, you will typically fetch data from a database using a while loop and then use a while
loop instead of foreach
to iterate through the fetched data and generate the HTML table. The concept is the same; you replace the sample data with your actual data source.