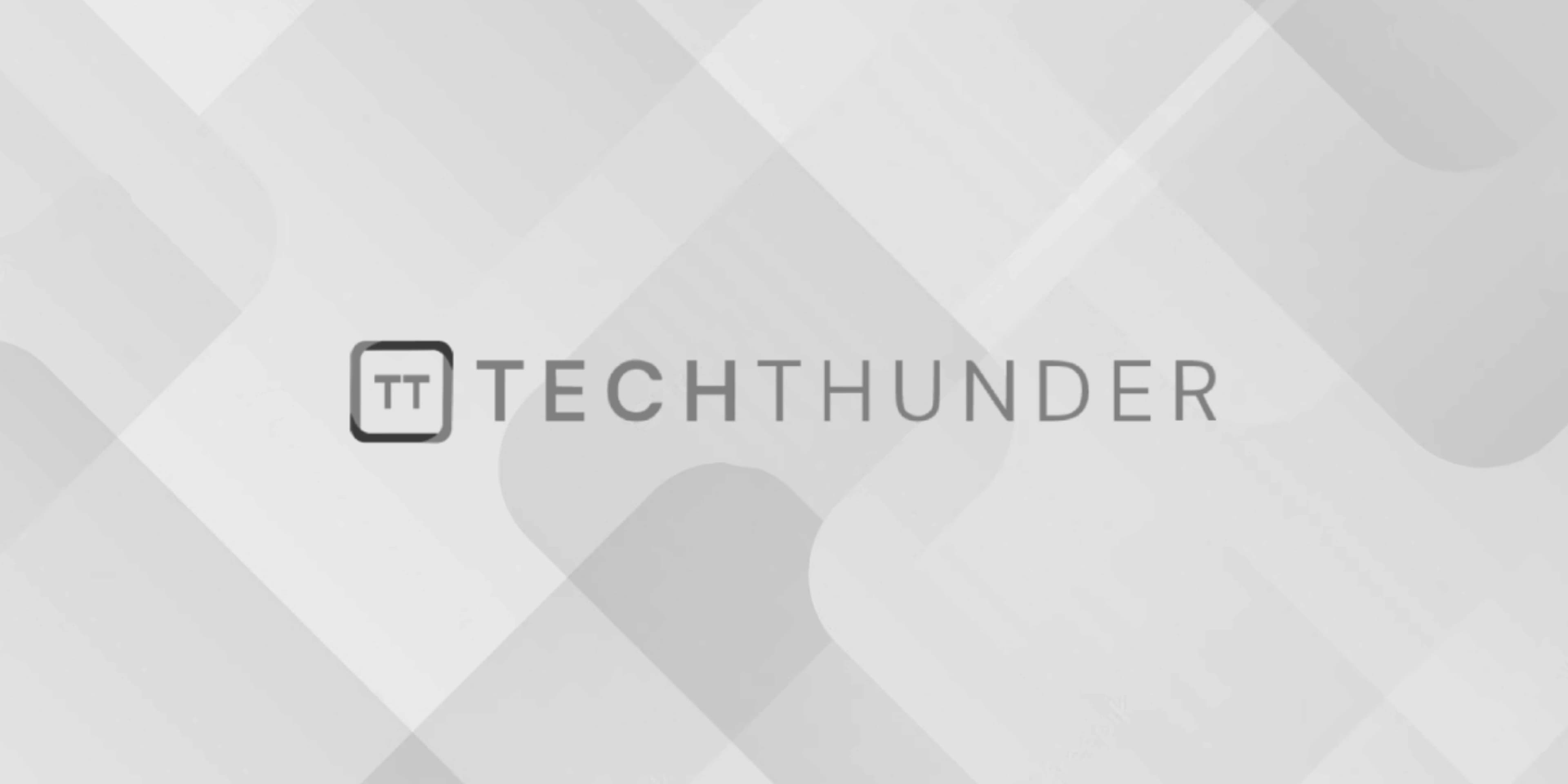
185 views
PHP Cookie
In PHP, cookies are small pieces of data that can be stored on the client’s web browser and are used to persistently store information between HTTP requests. Cookies are often used to remember user preferences, session data, or any other information that needs to be remembered across multiple visits or pages.
Here’s how you can set, retrieve, and delete cookies in PHP:
- Setting a Cookie:
You can set a cookie using thesetcookie()
function in PHP. The syntax for setting a cookie is as follows:
setcookie(name, value, expiration, path, domain, secure, httponly);
name
: The name of the cookie (a string).value
: The value of the cookie (can be a string or an array).expiration
: The expiration time of the cookie (in seconds since the Unix Epoch). If omitted, the cookie will expire when the browser session ends.path
: The path on the server where the cookie will be available. If omitted, it will be available for the entire domain.domain
: The domain for which the cookie is valid. If omitted, it will default to the current domain.secure
: If set totrue
, the cookie will only be sent over HTTPS connections.httponly
: If set totrue
, the cookie will be inaccessible to JavaScript, improving security.
Example:
// Set a cookie that expires in one day
setcookie("username", "JohnDoe", time() + (86400 * 1), "/");
- Retrieving a Cookie:
You can access the value of a cookie using the$_COOKIE
superglobal array in PHP.
Example:
if (isset($_COOKIE["username"])) {
echo "Welcome back, " . $_COOKIE["username"];
} else {
echo "Welcome, guest!";
}
- Deleting a Cookie:
To delete a cookie, you can set its expiration time to a past date. This will cause the browser to remove the cookie.
Example:
// Set the expiration time to a past date (e.g., one hour ago)
setcookie("username", "", time() - 3600, "/");
Keep in mind that cookies have limitations in terms of storage size (typically limited to a few kilobytes), and users can choose to disable cookies in their browsers. Therefore, do not store sensitive information in cookies and always handle the absence of cookies gracefully in your PHP code.