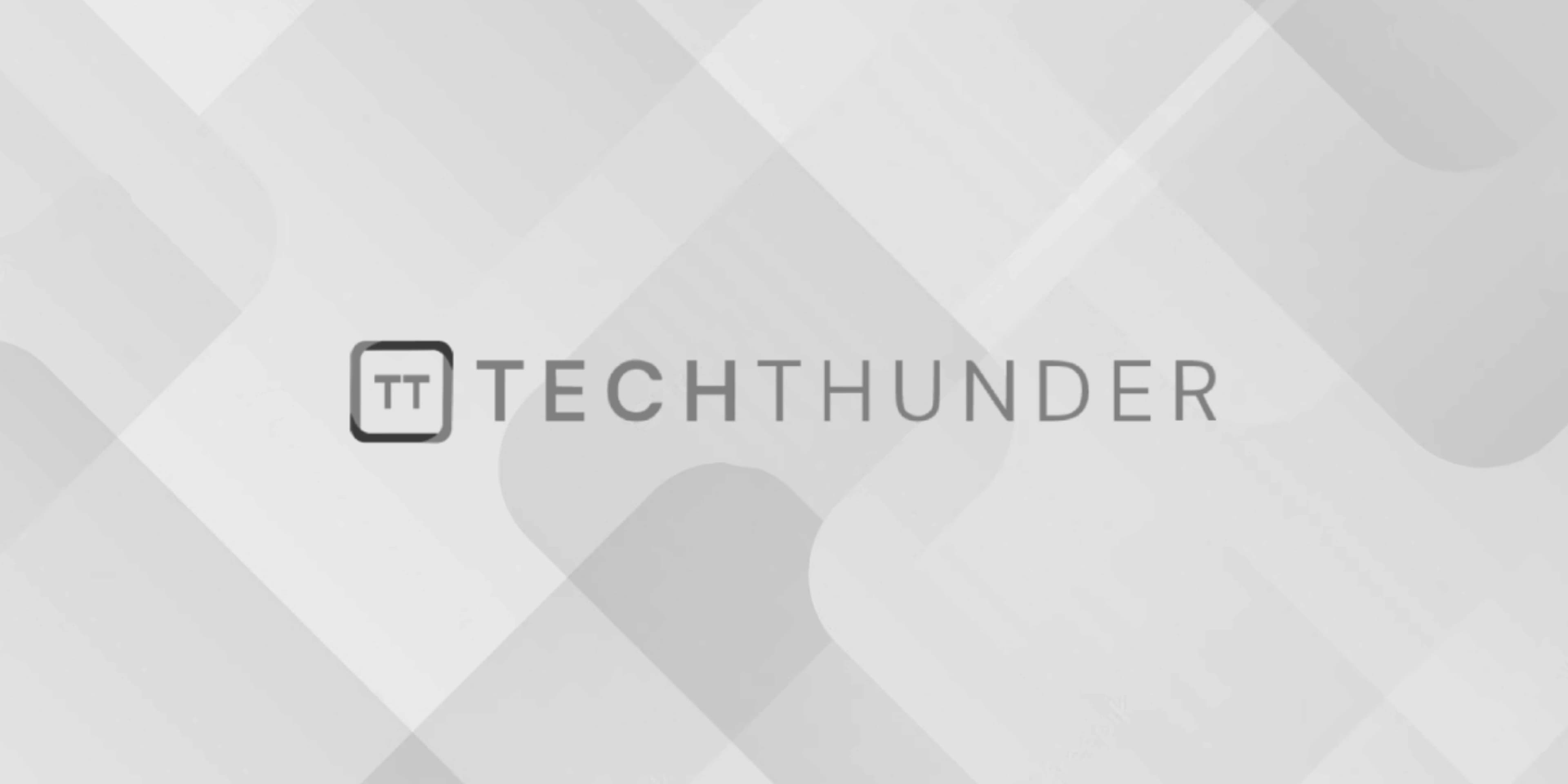
Exception Handling in PHP
Exception handling in PHP allows you to gracefully handle runtime errors and exceptions that occur during the execution of your PHP code. It helps you manage errors in a more controlled and structured manner, making your code more robust and easier to maintain.
The process of exception handling involves three main components:
- Throw an Exception:
When an exceptional situation occurs in your PHP code, you can “throw” an exception to indicate that an error has occurred. This is done using thethrow
keyword followed by an instance of theException
class or its derived classes.
function divide($numerator, $denominator) {
if ($denominator === 0) {
throw new Exception("Division by zero is not allowed.");
}
return $numerator / $denominator;
}
- Catch the Exception:
After throwing an exception, you can “catch” it using atry
block. Thetry
block contains the code that may potentially throw an exception. Immediately after thetry
block, you include one or morecatch
blocks to handle specific exceptions.
try {
$result = divide(10, 0);
echo "Result: " . $result;
} catch (Exception $e) {
echo "Exception caught: " . $e->getMessage();
}
- Handle the Exception:
In thecatch
block, you can implement the code that should be executed when a specific exception is caught. The$e
variable holds the exception object, allowing you to access information about the caught exception, such as its message, code, and stack trace.
The complete example with both throw and catch:
function divide($numerator, $denominator) {
if ($denominator === 0) {
throw new Exception("Division by zero is not allowed.");
}
return $numerator / $denominator;
}
try {
$result = divide(10, 0);
echo "Result: " . $result;
} catch (Exception $e) {
echo "Exception caught: " . $e->getMessage();
}
Output:
Exception caught: Division by zero is not allowed.
It’s worth noting that in addition to the built-in Exception
class, PHP allows you to define custom exception classes by extending the Exception
class. This allows you to handle different types of exceptions more precisely and make your exception handling more specific to your application’s needs.
By using exception handling, you can gracefully handle errors, log them for debugging purposes, and provide meaningful error messages to users while ensuring that your application continues to function correctly even when exceptions occur.