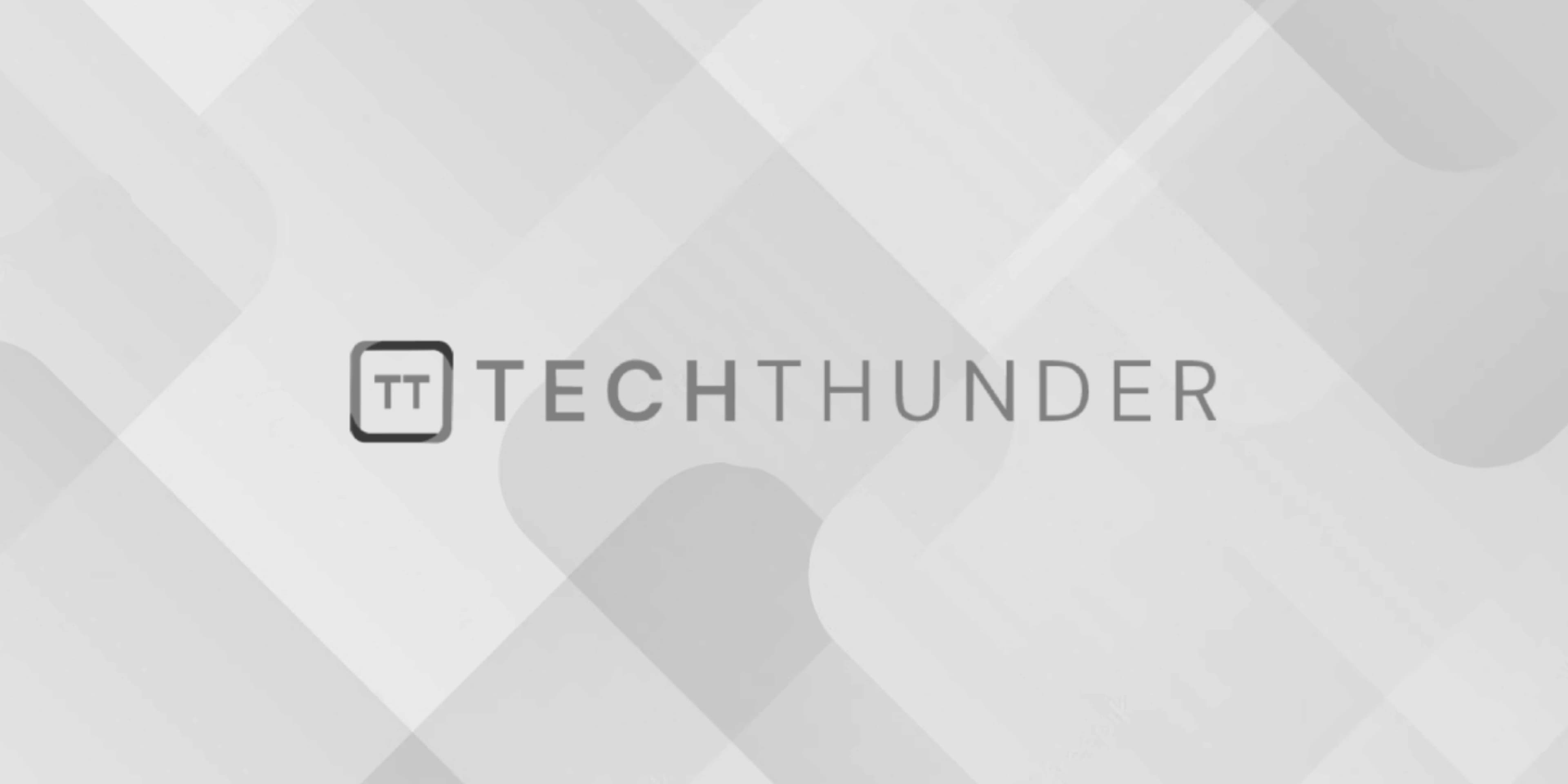
226 views
PHP String
In PHP, a string is a sequence of characters, such as letters, numbers, and special symbols, enclosed in single quotes (‘ ‘), double quotes (” “), or heredoc syntax (<<<
). Strings are one of the fundamental data types in PHP and are used to represent text and manipulate textual data.
Here are some examples of defining strings in PHP:
- Single-quoted string:
$string1 = 'This is a single-quoted string.';
- Double-quoted string:
$string2 = "This is a double-quoted string.";
- Heredoc syntax:
$string3 = <<<EOT
This is a heredoc string.
It can span multiple lines.
EOT;
- Nowdoc syntax (similar to heredoc, but does not interpret variables):
$string4 = <<<'EOT'
This is a nowdoc string.
It behaves like a single-quoted string.
EOT;
In PHP, strings support various operations and functions for manipulation, such as concatenation, substring extraction, searching, and replacement. Here are some common string operations:
- String Concatenation:
$firstName = "John";
$lastName = "Doe";
$fullName = $firstName . " " . $lastName; // Concatenates two strings with a space in between
echo $fullName; // Output: John Doe
- String Length:
$string = "Hello, world!";
$length = strlen($string); // Gets the length of the string
echo $length; // Output: 13
- Substring Extraction:
$string = "Hello, world!";
$substring = substr($string, 0, 5); // Extracts the first 5 characters
echo $substring; // Output: Hello
- Searching and Replacing:
$string = "Hello, world!";
$search = "world";
$replace = "everyone";
$newString = str_replace($search, $replace, $string); // Replaces "world" with "everyone"
echo $newString; // Output: Hello, everyone!
- Case Conversion:
$string = "Hello, world!";
$upperCase = strtoupper($string); // Converts the string to uppercase
$lowerCase = strtolower($string); // Converts the string to lowercase
echo $upperCase; // Output: HELLO, WORLD!
echo $lowerCase; // Output: hello, world!
PHP provides a wide range of built-in string functions to handle various string manipulation tasks. Always refer to the official PHP documentation for more details and a comprehensive list of available functions.