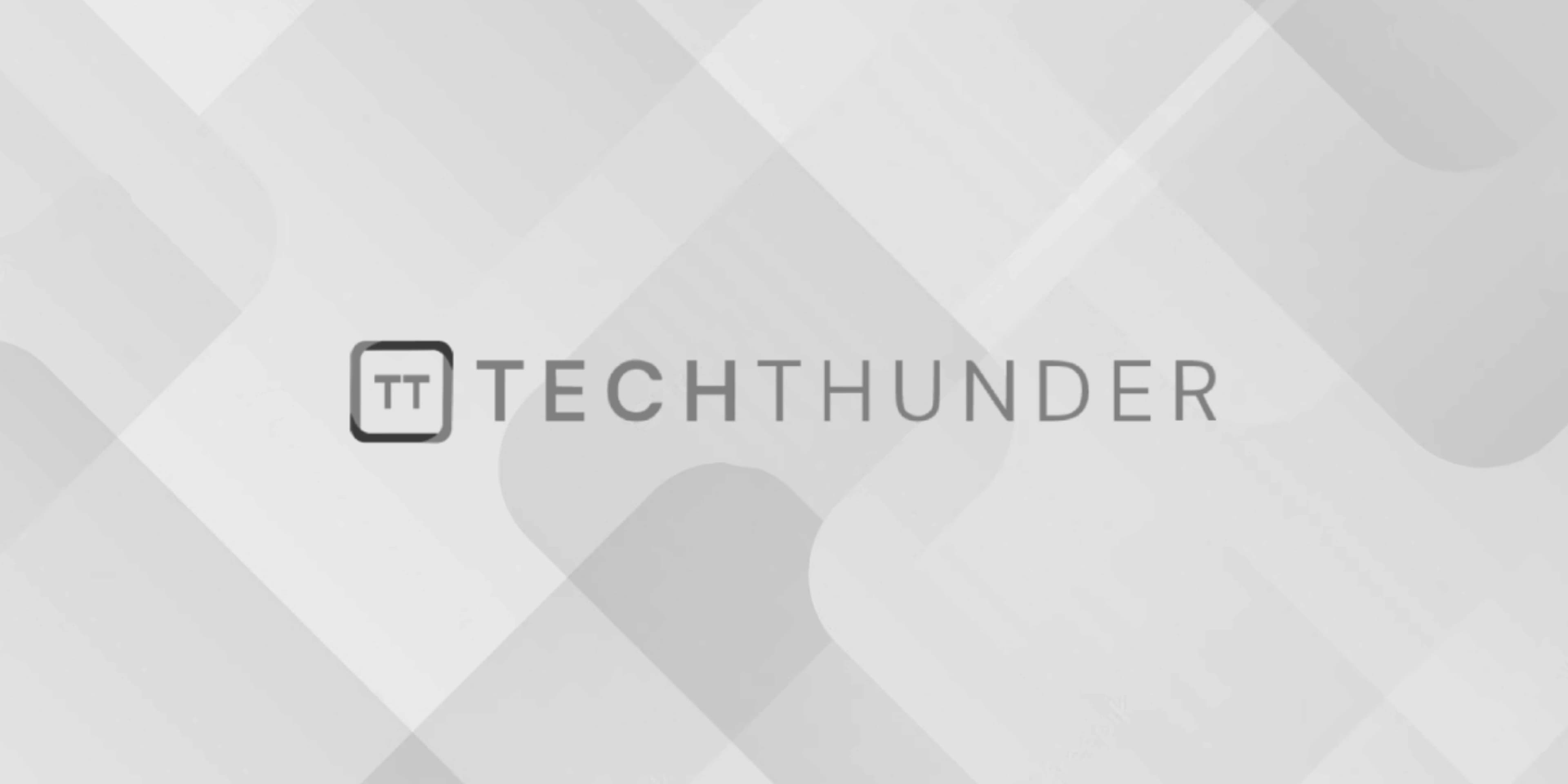
500 views
PHP MySQLi INSERT
In PHP, you can use MySQLi (MySQL Improved) to perform database operations, including inserting data into a MySQL database table. Here’s how you can use MySQLi to perform an INSERT operation:
- Connect to the MySQL Database:
First, you need to establish a connection to the MySQL database using themysqli_connect()
function. This function takes the database server hostname, username, password, and database name as parameters. Make sure to replace the placeholders with your actual database credentials.
<?php
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$dbname = "your_database";
// Create a connection
$conn = mysqli_connect($servername, $username, $password, $dbname);
// Check the connection
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
?>
- Prepare and Execute the INSERT Query:
Once the connection is established, you can prepare and execute an INSERT query to insert data into the database table. Use themysqli_query()
function to execute the query.
<?php
// Assuming you have a table named "users" with columns "id", "name", and "email"
$name = "John Doe";
$email = "[email protected]";
// Prepare the INSERT query
$sql = "INSERT INTO users (name, email) VALUES ('$name', '$email')";
// Execute the query
if (mysqli_query($conn, $sql)) {
echo "New record inserted successfully.";
} else {
echo "Error: " . $sql . "<br>" . mysqli_error($conn);
}
?>
- Close the Database Connection:
After executing the query, it’s essential to close the database connection using themysqli_close()
function to free up resources.
<?php
// Close the connection
mysqli_close($conn);
?>
Always remember to use proper data sanitization and validation techniques to prevent SQL injection and other security issues. For better security, consider using prepared statements or parameterized queries instead of directly embedding user input in the SQL query.
Important Note: The use of plain SQL queries like the above example can lead to SQL injection vulnerabilities if user input is not properly sanitized. It is highly recommended to use prepared statements or other safe database access methods to prevent potential security risks.