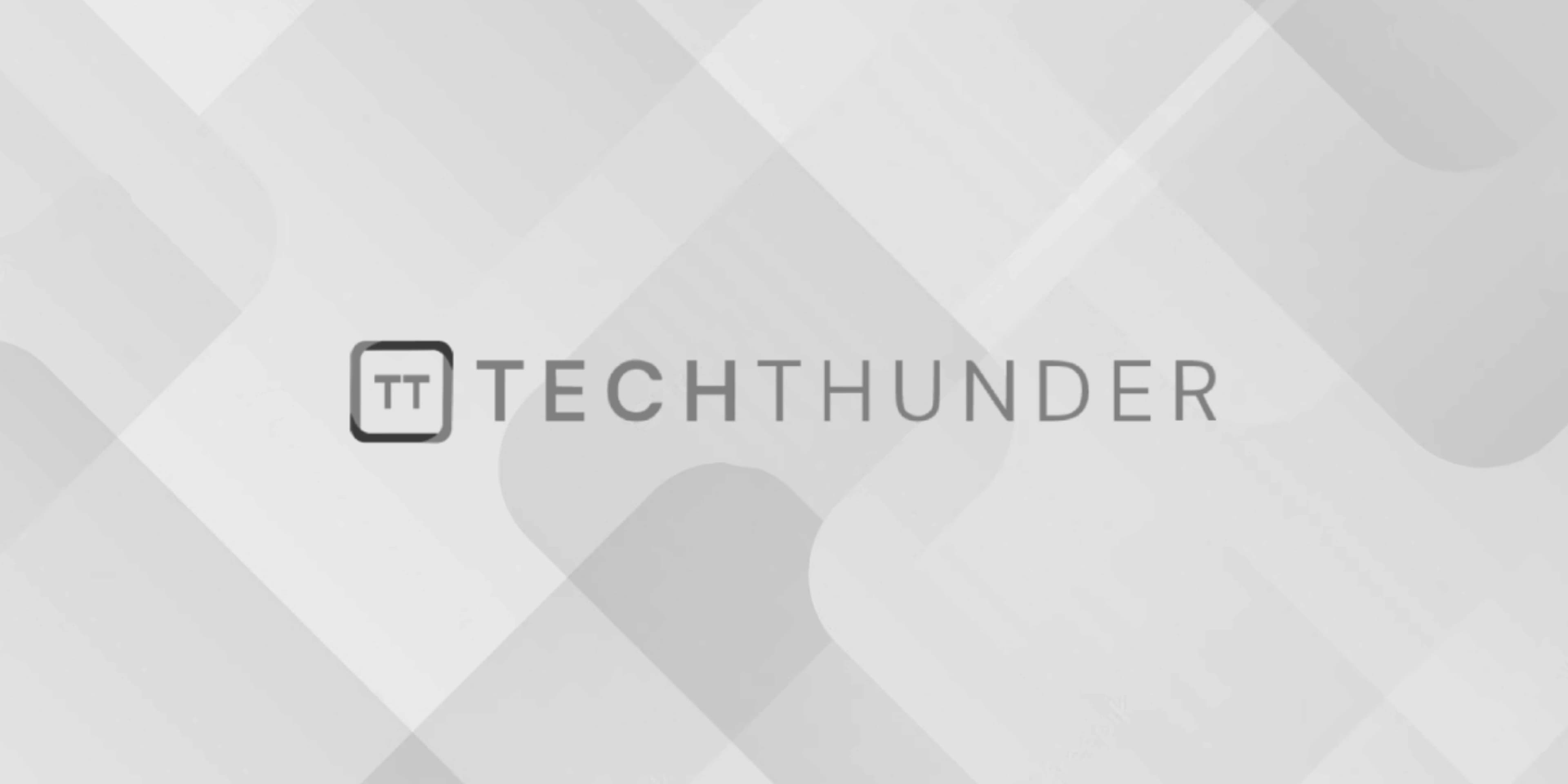
PHP Integer
In PHP, an integer is a data type used to represent whole numbers without any fractional or decimal parts. Integers can be positive, negative, or zero. They are used to store numerical values, such as counts, identifiers, and other whole number values.
The syntax to define an integer variable in PHP is as follows:
$intVar = 42; // Example of an integer variable
Integers can be specified in decimal (base 10), hexadecimal (base 16), octal (base 8), or binary (base 2) representations.
Examples of integer variables:
$age = 30; // Age of a person
$quantity = -5; // Negative quantity (e.g., for returned items)
$employeeId = 1001; // Employee ID
$octalNumber = 014; // Octal representation (014 in octal is equal to 12 in decimal)
$hexNumber = 0x1A; // Hexadecimal representation (0x1A in hexadecimal is equal to 26 in decimal)
$binaryNumber = 0b1101; // Binary representation (0b1101 in binary is equal to 13 in decimal)
It’s essential to note that PHP automatically converts numbers between different representations as needed. For example, if you perform arithmetic operations with integers in different bases, PHP will automatically convert them to a common base before the operation.
To perform arithmetic operations with integers, you can use standard arithmetic operators like +
, -
, *
, /
, %
(modulo), etc. For example:
$num1 = 10;
$num2 = 5;
$sum = $num1 + $num2; // Addition
$diff = $num1 - $num2; // Subtraction
$product = $num1 * $num2; // Multiplication
$quotient = $num1 / $num2; // Division
$remainder = $num1 % $num2; // Modulo (remainder of division)
echo "Sum: " . $sum . "\n";
echo "Difference: " . $diff . "\n";
echo "Product: " . $product . "\n";
echo "Quotient: " . $quotient . "\n";
echo "Remainder: " . $remainder . "\n";
Output:
Sum: 15
Difference: 5
Product: 50
Quotient: 2
Remainder: 0
In the example above, we performed various arithmetic operations with integer variables and displayed the results.
Keep in mind that PHP automatically converts numeric strings to integers when necessary. For example, if you use an integer in a context where a string is expected, PHP will automatically convert the integer to a string. Similarly, if you use a string that contains a valid numeric representation in a numeric context, PHP will automatically convert the string to an integer.
For example:
$intValue = 42; // Integer
$strValue = "42"; // String
// Automatic conversion from integer to string
echo "Integer to String: " . $intValue . "\n"; // Output: Integer to String: 42
// Automatic conversion from string to integer
$sum = $strValue + 5; // Adding a number to a string (PHP automatically converts the string to an integer)
echo "String to Integer: " . $sum . "\n"; // Output: String to Integer: 47
In summary, integers in PHP are used to represent whole numbers, and you can perform various arithmetic operations on them using standard arithmetic operators. Keep in mind the automatic type conversion that PHP performs in certain situations, and be aware of potential precision limitations when dealing with large numbers.