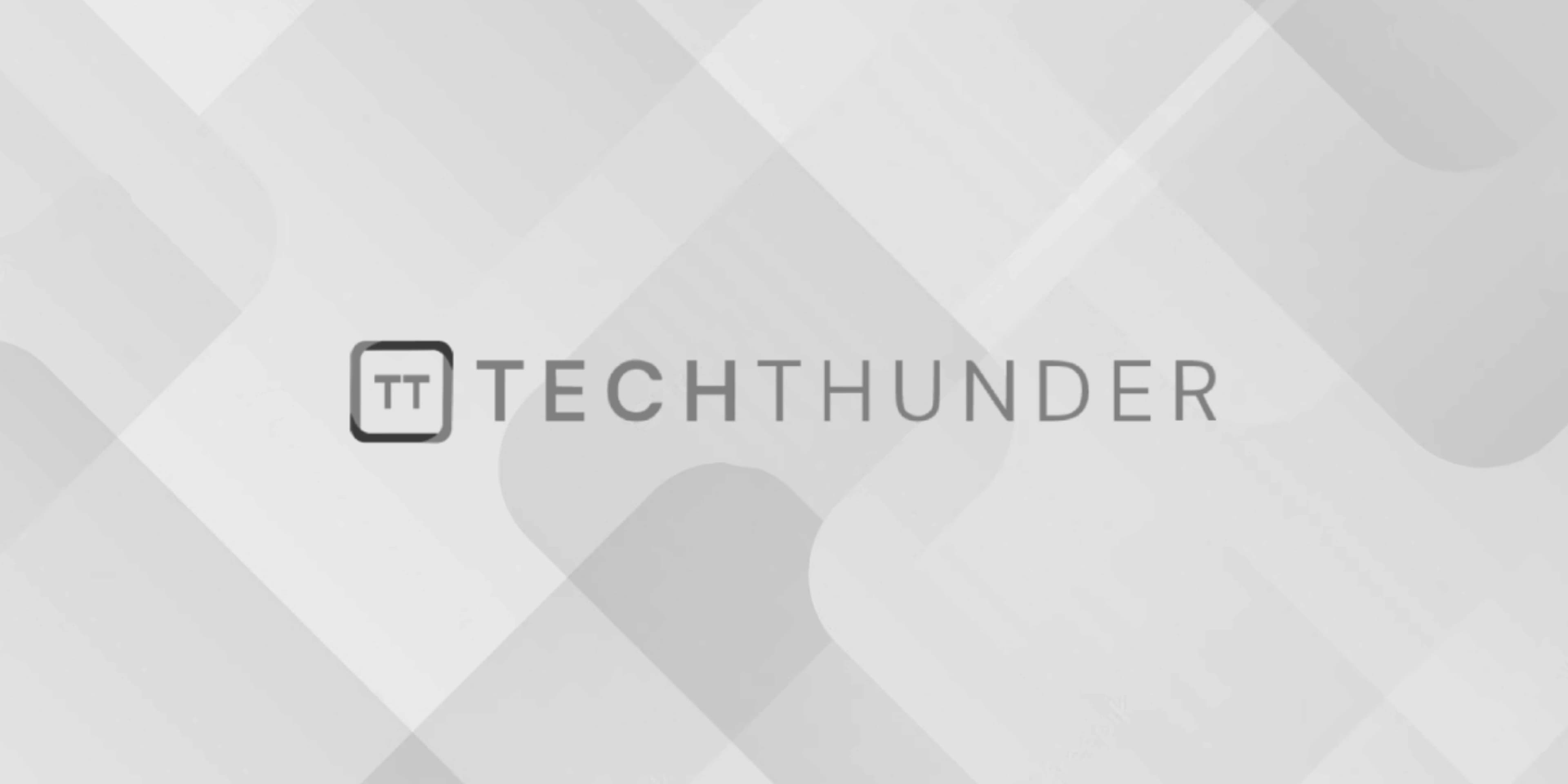
PHP Switch
In PHP, the switch
statement is used to perform different actions based on the value of a single variable or expression. It provides an alternative way to write complex branching logic compared to using multiple if
and elseif
statements.
The basic syntax of the switch
statement is as follows:
switch (expression) {
case value1:
// Code to be executed if expression matches value1
break;
case value2:
// Code to be executed if expression matches value2
break;
// More cases can be added as needed
default:
// Code to be executed if none of the cases match
break;
}
Here’s an example of a simple switch
statement:
$day = "Monday";
switch ($day) {
case "Monday":
echo "Today is Monday.";
break;
case "Tuesday":
echo "Today is Tuesday.";
break;
case "Wednesday":
echo "Today is Wednesday.";
break;
default:
echo "Today is not Monday, Tuesday, or Wednesday.";
break;
}
In this example, the variable $day
is used as the expression for the switch
statement. The code checks the value of $day
against each case
and executes the corresponding code block for the matching case. If none of the cases match, the code inside the default
block will be executed.
You can have multiple cases that share the same code block by omitting the break
statement:
$day = "Monday";
switch ($day) {
case "Monday":
case "Tuesday":
case "Wednesday":
echo "It's a weekday.";
break;
case "Saturday":
case "Sunday":
echo "It's the weekend.";
break;
default:
echo "Invalid day.";
break;
}
In this example, if the value of $day
is “Monday”, “Tuesday”, or “Wednesday”, the code will output “It’s a weekday.” If the value is “Saturday” or “Sunday”, it will output “It’s the weekend.” If the value is anything else, it will output “Invalid day.”
The switch
statement can be a concise and clean way to handle multiple conditions and improve the readability of your code when dealing with many possible cases based on a single variable or expression.