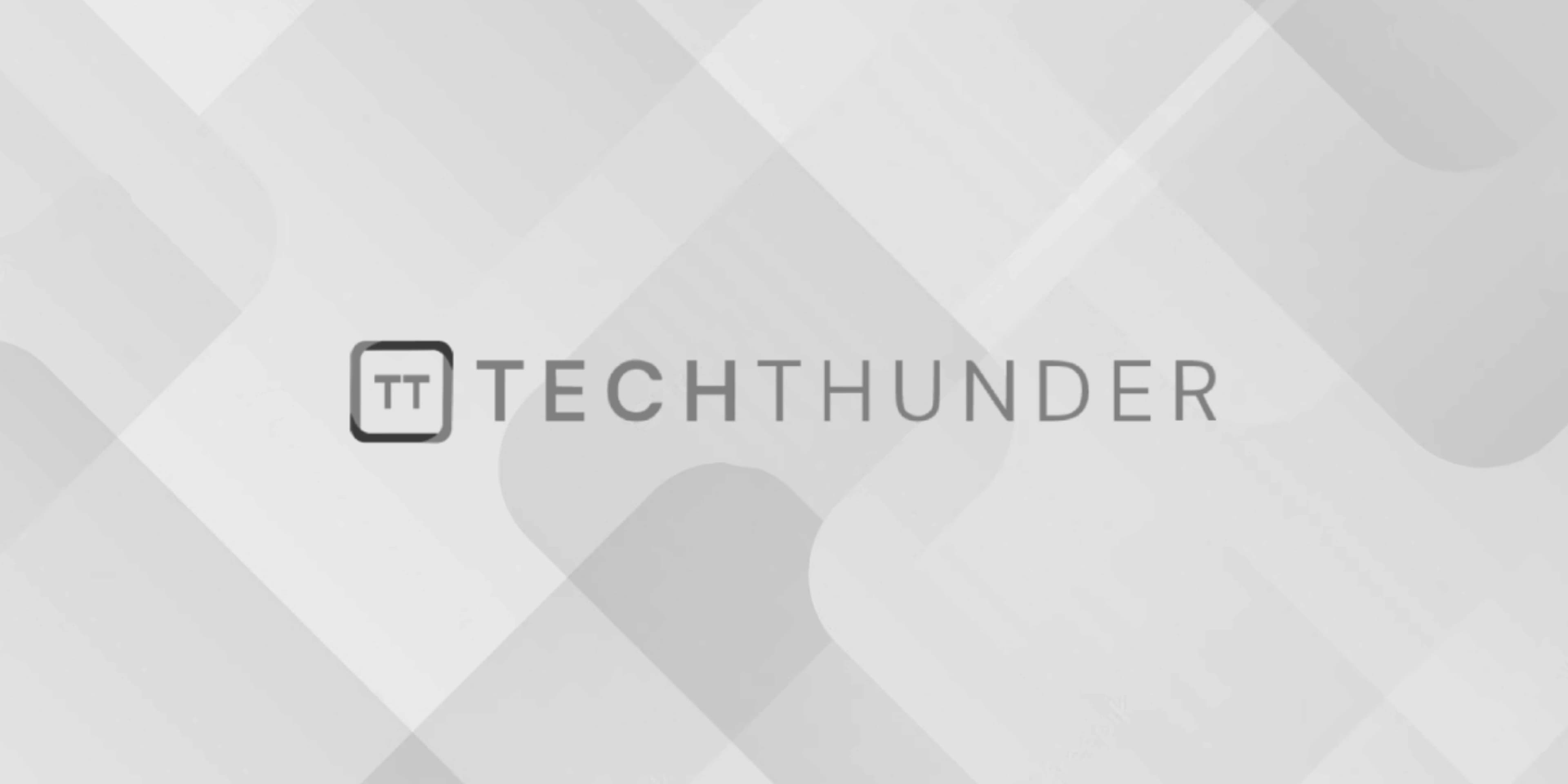
239 views
PHP File Handling
PHP provides various functions for file handling, allowing you to read, write, and manipulate files on the server’s file system. Here are some commonly used PHP file handling functions:
- Opening a File:
To open a file for reading or writing, you can use thefopen()
function. It takes two parameters: the filename and the mode (read, write, append, etc.).
Example (Opening a file for reading):
$filename = "example.txt";
$file_handle = fopen($filename, "r");
- Reading from a File:
You can read content from an opened file using functions likefgets()
orfread()
.fgets()
reads a line from the file, andfread()
reads a specified number of bytes.
Example (Reading a line from the file):
$line = fgets($file_handle);
- Writing to a File:
To write content to a file, you can use functions likefwrite()
orfile_put_contents()
.fwrite()
writes a specified number of bytes, andfile_put_contents()
writes the entire content at once.
Example (Writing to a file using fwrite()):
$content = "This is a line of text to write to the file.";
fwrite($file_handle, $content);
Example (Writing to a file using file_put_contents()):
$content = "This is a line of text to write to the file.";
file_put_contents($filename, $content);
- Closing a File:
After reading or writing to a file, it’s essential to close the file handle using thefclose()
function.
Example (Closing the file handle):
fclose($file_handle);
- Checking if a File Exists:
You can check if a file exists using thefile_exists()
function.
Example (Checking if the file exists):
$filename = "example.txt";
if (file_exists($filename)) {
echo "The file exists.";
} else {
echo "The file does not exist.";
}
- Deleting a File:
To delete a file, you can use theunlink()
function.
Example (Deleting the file):
$filename = "example.txt";
unlink($filename);
- File Size and File Information:
You can get information about a file, such as its size and other attributes, using functions likefilesize()
andfilemtime()
.
Example (Getting the file size and last modification time):
$filename = "example.txt";
$filesize = filesize($filename); // Size of the file in bytes
$last_modified = filemtime($filename); // Timestamp of the last modification
Always be cautious when working with files and ensure proper validation and sanitization of file paths and content to prevent security vulnerabilities like directory traversal attacks or code injection.