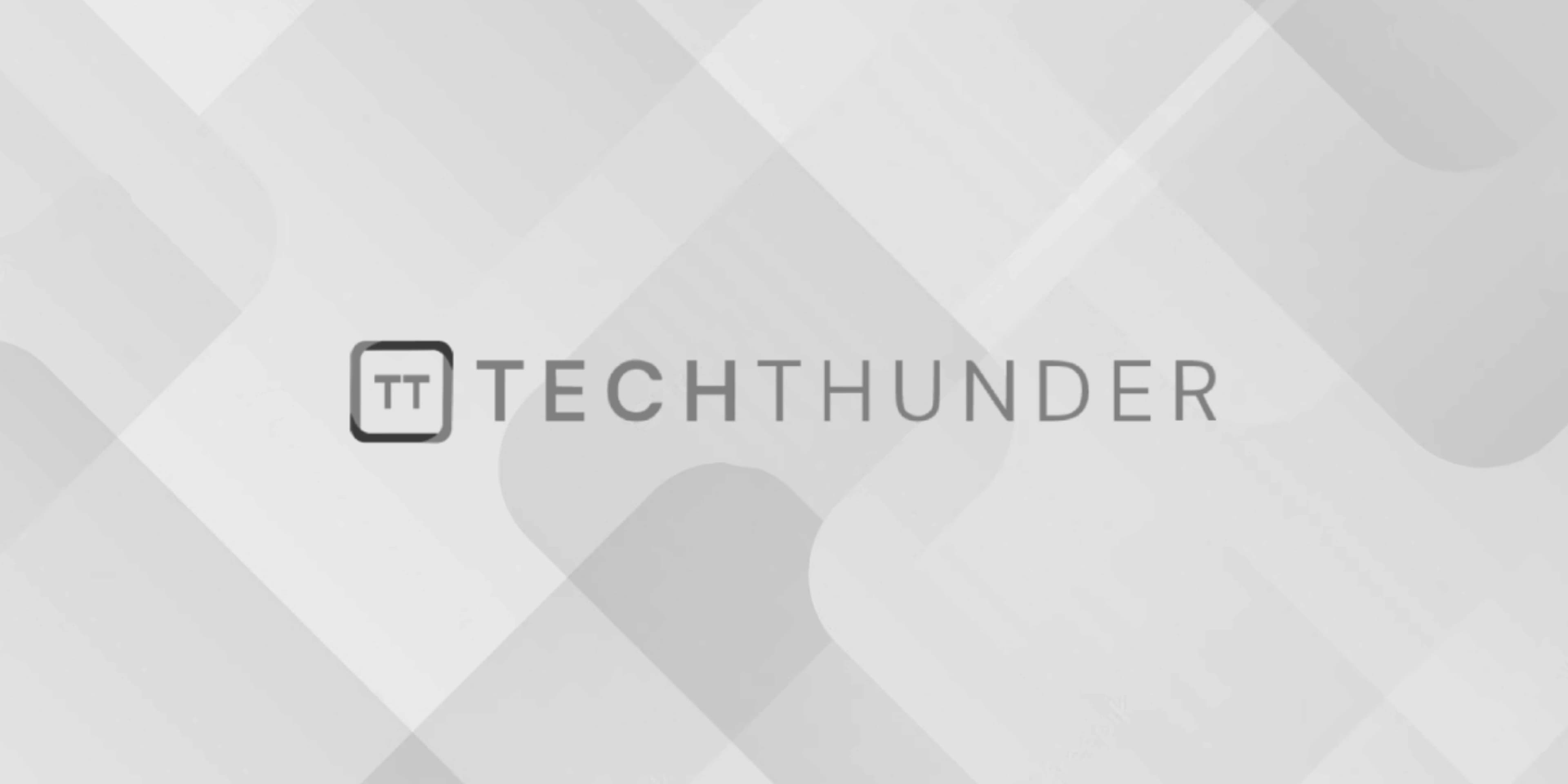
PHP If else
In PHP, the if-else
statement is used to execute different blocks of code based on a condition. The if
block contains the code that will be executed if the condition evaluates to true, and the else
block contains the code that will be executed if the condition evaluates to false.
Here’s the syntax of the if-else
statement:
if (condition) {
// Code to be executed if the condition is true
} else {
// Code to be executed if the condition is false
}
Example of the if-else
statement:
$age = 25;
if ($age >= 18) {
echo "You are an adult.";
} else {
echo "You are a minor.";
}
In this example, we have a variable $age
with a value of 25. The if-else
statement checks whether the value of $age
is greater than or equal to 18. If the condition is true (which it is in this case), the code inside the if
block will be executed, and it will output “You are an adult.” If the condition is false, the code inside the else
block will be executed, and it will output “You are a minor.”
Additionally, you can use the elseif
keyword to add more conditions to the if-else
statement:
$score = 75;
if ($score >= 90) {
echo "Excellent!";
} elseif ($score >= 80) {
echo "Very good!";
} elseif ($score >= 70) {
echo "Good!";
} else {
echo "Keep practicing!";
}
In this example, the elseif
keyword is used to add additional conditions to the if-else
statement. The code will check the value of $score
and print different messages based on the score range.
The if-else
statement allows you to control the flow of your PHP code based on specific conditions, making your code more flexible and responsive to different situations.