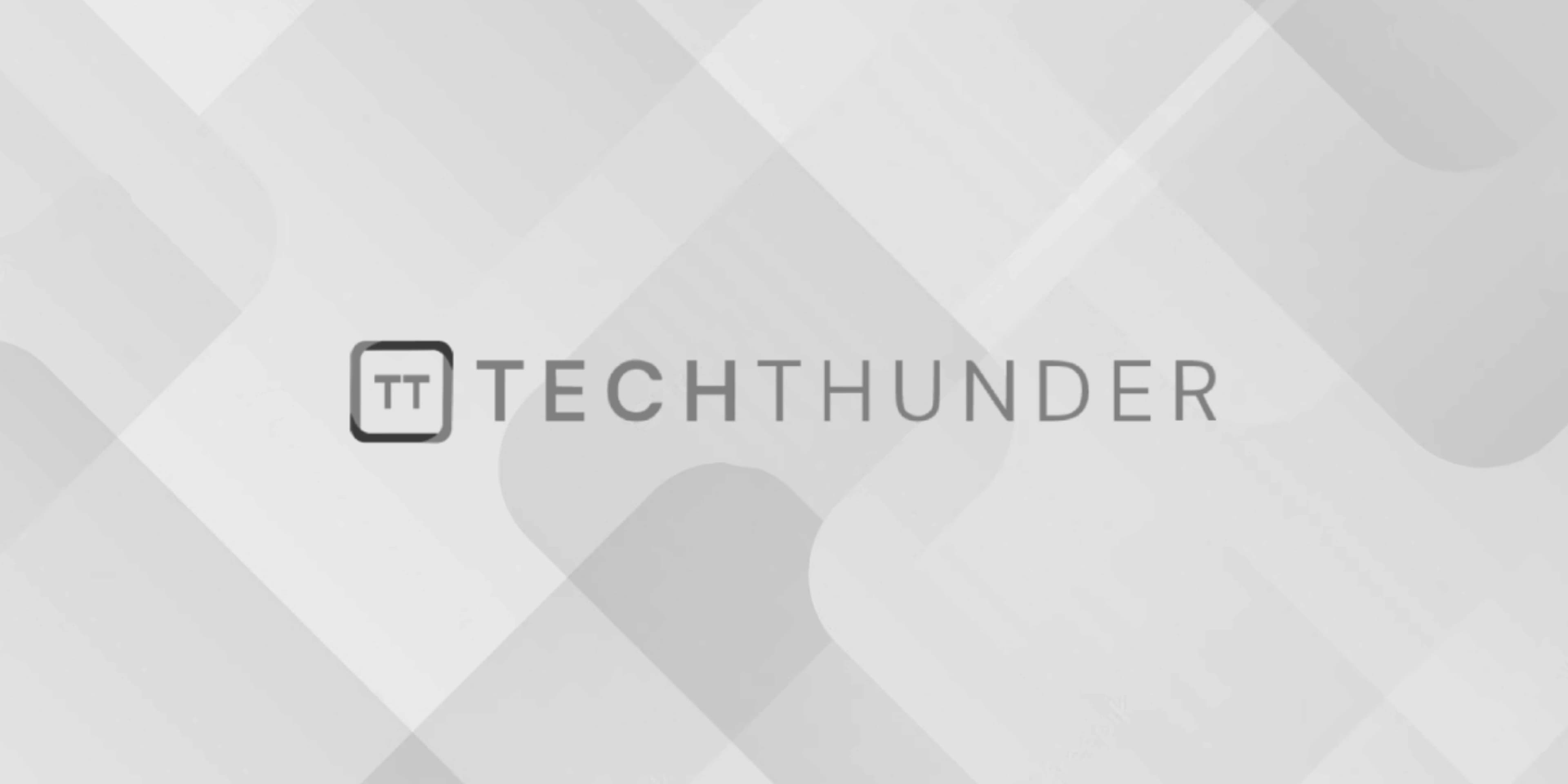
PHP Ajax Multiple Image Upload
To implement a PHP Ajax multiple image upload, you’ll need to create an HTML form with the necessary input fields for file selection and use JavaScript (specifically, Ajax) to handle the asynchronous file upload. Below is a step-by-step guide to creating a PHP Ajax multiple image upload:
- Create the HTML Form:
Create an HTML form that includes an input element with thetype="file"
attribute set to allow multiple file selections (multiple
), and a button to trigger the file upload.
<!DOCTYPE html>
<html>
<head>
<title>Multiple Image Upload</title>
</head>
<body>
<h2>Multiple Image Upload</h2>
<form id="uploadForm" enctype="multipart/form-data">
<input type="file" name="images[]" multiple>
<input type="submit" value="Upload">
</form>
<div id="response"></div>
</body>
</html>
- Implement the JavaScript (Ajax) Code:
Create a JavaScript code to handle the form submission using Ajax. This will allow you to upload the selected images without reloading the entire page.
<script>
document.getElementById("uploadForm").addEventListener("submit", function(event) {
event.preventDefault(); // Prevent default form submission behavior
var formData = new FormData(this);
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
document.getElementById("response").innerHTML = xhr.responseText;
}
};
xhr.open("POST", "upload.php", true);
xhr.send(formData);
});
</script>
- Create the PHP Upload Script:
Create a PHP script (e.g.,upload.php
) to handle the file upload. This script will receive the uploaded images, process them, and move them to a desired folder.
<?php
if (isset($_FILES['images']) && !empty($_FILES['images'])) {
$uploadsDir = 'uploads/'; // Directory to save uploaded images
$uploadedImages = $_FILES['images'];
$fileCount = count($uploadedImages['name']);
$successCount = 0;
for ($i = 0; $i < $fileCount; $i++) {
$tempFilePath = $uploadedImages['tmp_name'][$i];
$targetFilePath = $uploadsDir . basename($uploadedImages['name'][$i]);
if (move_uploaded_file($tempFilePath, $targetFilePath)) {
$successCount++;
}
}
echo "$successCount out of $fileCount images uploaded successfully.";
} else {
echo "No images selected for upload.";
}
?>
- Create the Uploads Directory:
Ensure that you have created a folder named ‘uploads’ in the same directory as the PHP scripts to save the uploaded images.
With this setup, users can select multiple images using the HTML form, and when they click the “Upload” button, the selected images will be asynchronously uploaded to the server using Ajax. The PHP script will handle the file upload and move the images to the ‘uploads’ directory.
Remember to set appropriate permissions on the ‘uploads’ directory to allow the web server to write files to it. Additionally, always validate and sanitize user inputs to ensure the security of your application.