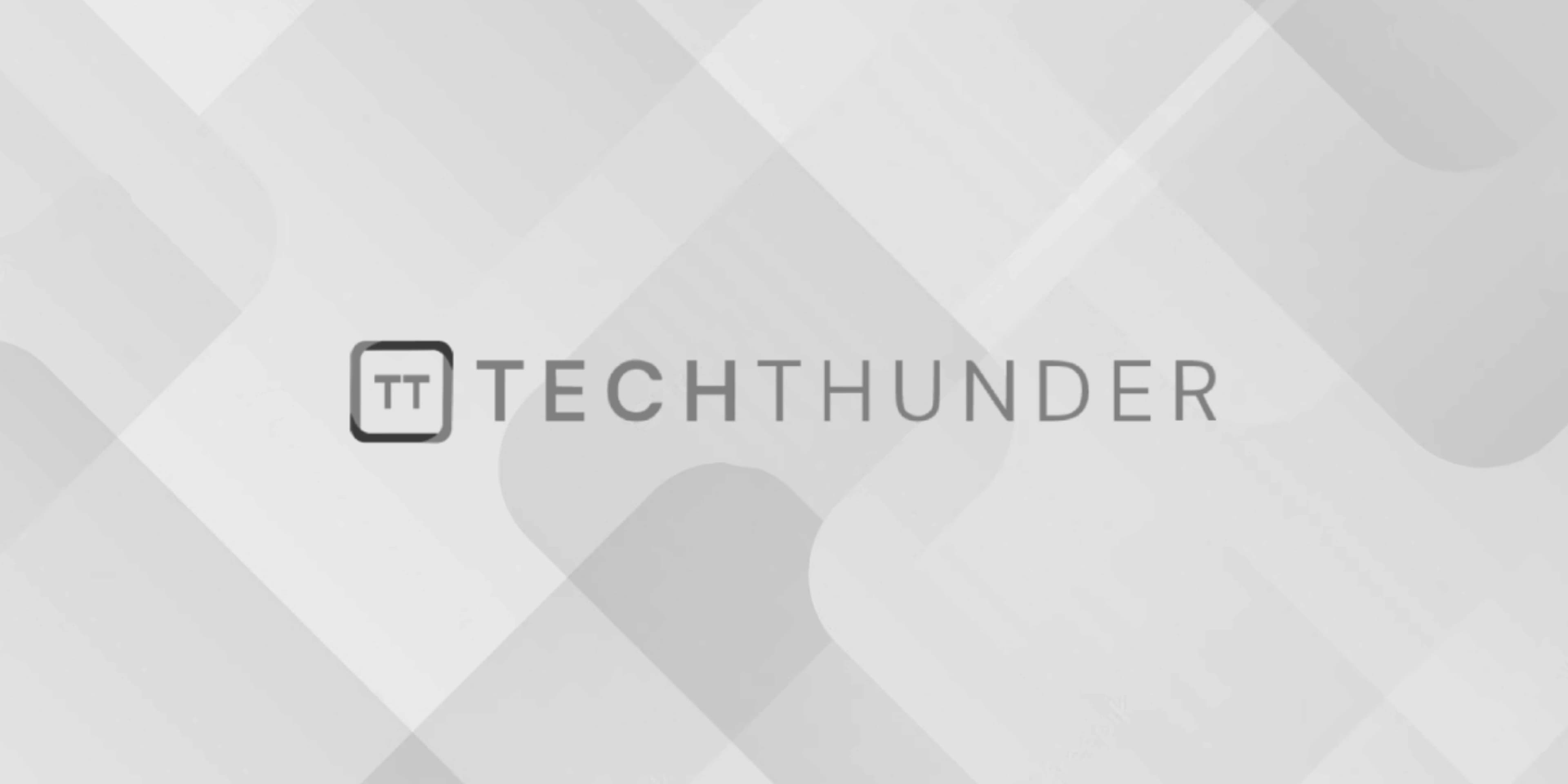
OOPs Encapsulation
In object-oriented programming (OOP), encapsulation is one of the four fundamental principles of OOP design, along with inheritance, polymorphism, and abstraction. Encapsulation is the concept of bundling data (attributes or properties) and the methods (functions or behavior) that operate on that data within a single unit called a class. The main idea behind encapsulation is to hide the internal details of an object and expose only the necessary interfaces to interact with it. In simpler terms, encapsulation allows you to protect the internal state of an object from direct access and modification by external code.
Encapsulation is achieved in OOP through the use of access specifiers (public, protected, private) that control the visibility of class members (properties and methods) from outside the class. By defining class members with the appropriate access specifiers, you can control what data and behavior are accessible to the outside world and what remains hidden and internal to the class.
The access specifiers are as follows:
- Public: Members declared as public are accessible from anywhere, both within and outside the class.
- Protected: Members declared as protected are accessible within the class itself and its subclasses (inheritance), but not from outside the class.
- Private: Members declared as private are only accessible within the class itself, not even by its subclasses.
Example:
class Car {
private $model;
protected $price;
public $color;
public function __construct($model, $price, $color) {
$this->model = $model;
$this->price = $price;
$this->color = $color;
}
public function start() {
echo "Starting the car...\n";
}
public function displayPrice() {
echo "Car price: $" . $this->price . "\n";
}
}
$car1 = new Car("Honda Civic", 25000, "Blue");
$car1->start(); // Output: Starting the car...
echo $car1->color; // Output: Blue
$car1->displayPrice(); // Output: Car price: $25000
In the example above, we have a Car
class with three properties: $model
, $price
, and $color
. The properties are declared with different access specifiers. $model
is private, $price
is protected, and $color
is public.
By declaring $model
as private, we ensure that it cannot be accessed directly from outside the class. It remains hidden and can only be accessed and modified through methods defined within the class.
By declaring $price
as protected, we allow it to be accessed from within the class and its subclasses (if any), but not from outside the class.
By declaring $color
as public, we make it accessible from anywhere, allowing direct access and modification from outside the class.
Encapsulation helps improve the security and maintainability of your code by hiding implementation details and exposing only necessary interfaces. It also allows you to change the internal implementation of a class without affecting the code that uses it, as long as the public interface remains the same.