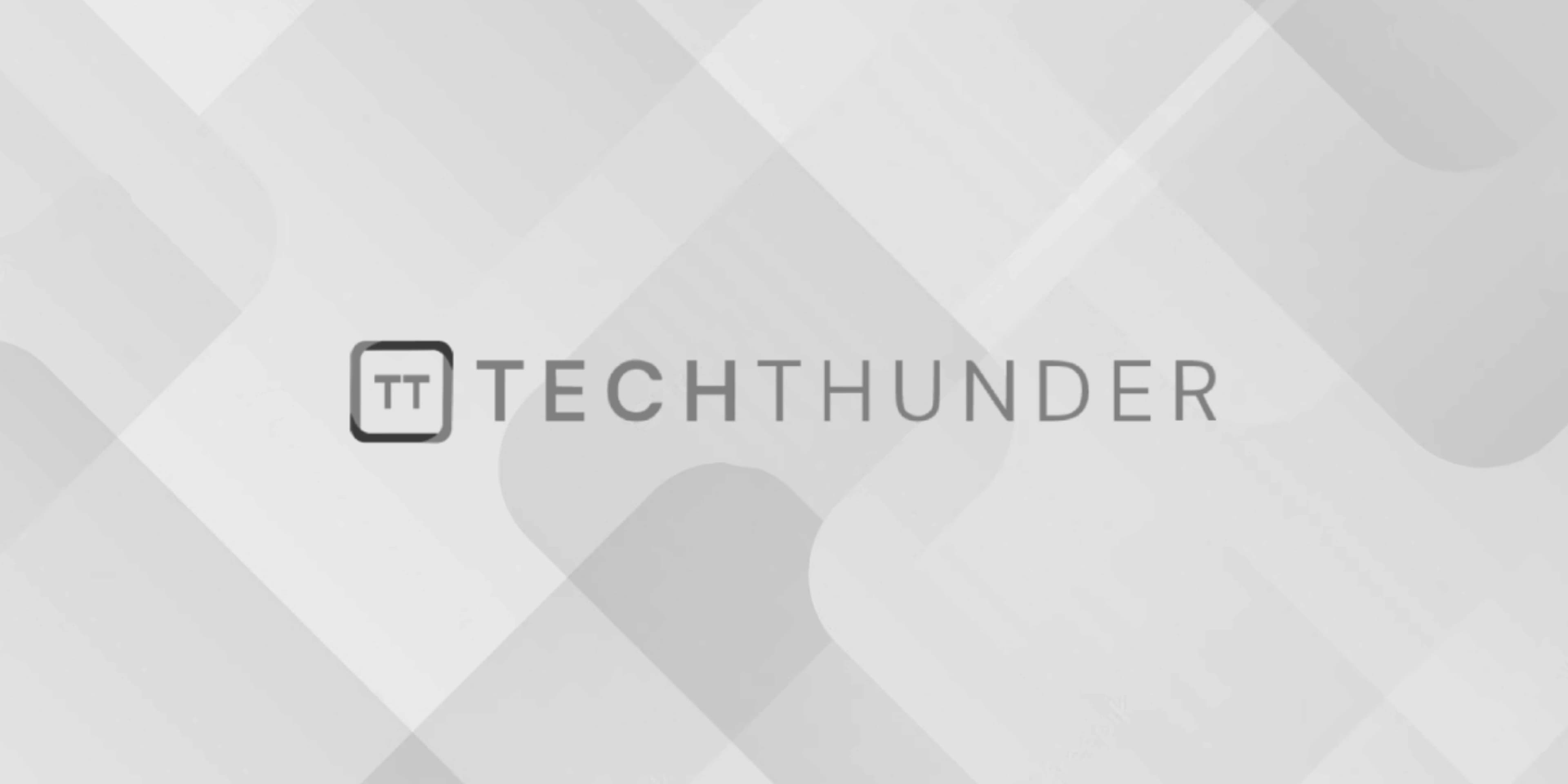
292 views
OOPs Abstract vs Class vs Interface
In object-oriented programming (OOP), abstract classes, regular classes, and interfaces are three fundamental concepts used for designing and implementing object-oriented systems. Each of them serves a different purpose and has its own characteristics.
- Abstract Class:
An abstract class is a class that cannot be instantiated on its own; it serves as a blueprint for other classes to inherit from. It can contain both abstract (unimplemented) methods and concrete (implemented) methods. Abstract methods are declared without a body and must be implemented by the classes that extend the abstract class. Abstract classes can also have regular properties and methods with implementations.
Key points about abstract classes:
- Cannot be instantiated directly.
- Can contain both abstract and concrete methods.
- Abstract methods must be implemented by the subclass.
- Can have regular properties and methods with implementations.
Example:
abstract class Shape {
abstract public function calculateArea();
abstract public function calculatePerimeter();
public function printDetails() {
echo "Shape details:\n";
echo "Area: " . $this->calculateArea() . "\n";
echo "Perimeter: " . $this->calculatePerimeter() . "\n";
}
}
- Class (Regular Class):
A regular class is a standard class that can be instantiated to create objects. It can contain properties, methods, constructors, and other class members. Unlike abstract classes and interfaces, regular classes do not have restrictions on their methods, meaning they can have concrete methods (with implementations) but cannot have abstract methods.
Example:
class Rectangle extends Shape {
private $length;
private $width;
public function __construct($length, $width) {
$this->length = $length;
$this->width = $width;
}
public function calculateArea() {
return $this->length * $this->width;
}
public function calculatePerimeter() {
return 2 * ($this->length + $this->width);
}
}
- Interface:
An interface is a contract that defines a set of method signatures without implementations. It only declares the method names, parameters, and return types that classes implementing the interface must adhere to. A class can implement multiple interfaces, allowing it to inherit and provide implementations for all the methods defined in those interfaces.
Key points about interfaces:
- Cannot be instantiated directly.
- Only contain method signatures, no implementations.
- A class can implement multiple interfaces.
- Provide a way to achieve multiple inheritance in PHP.
Example:
interface LoggerInterface {
public function logMessage($message);
}
class FileLogger implements LoggerInterface {
public function logMessage($message) {
// Implementation for logging to a file
}
}
class DatabaseLogger implements LoggerInterface {
public function logMessage($message) {
// Implementation for logging to a database
}
}
In summary, abstract classes are used when you want to define a common set of properties and methods for subclasses to inherit, regular classes are for creating objects directly, and interfaces are used for specifying method contracts that multiple classes can implement. Each of these concepts plays a vital role in OOP and helps in building flexible and maintainable code.