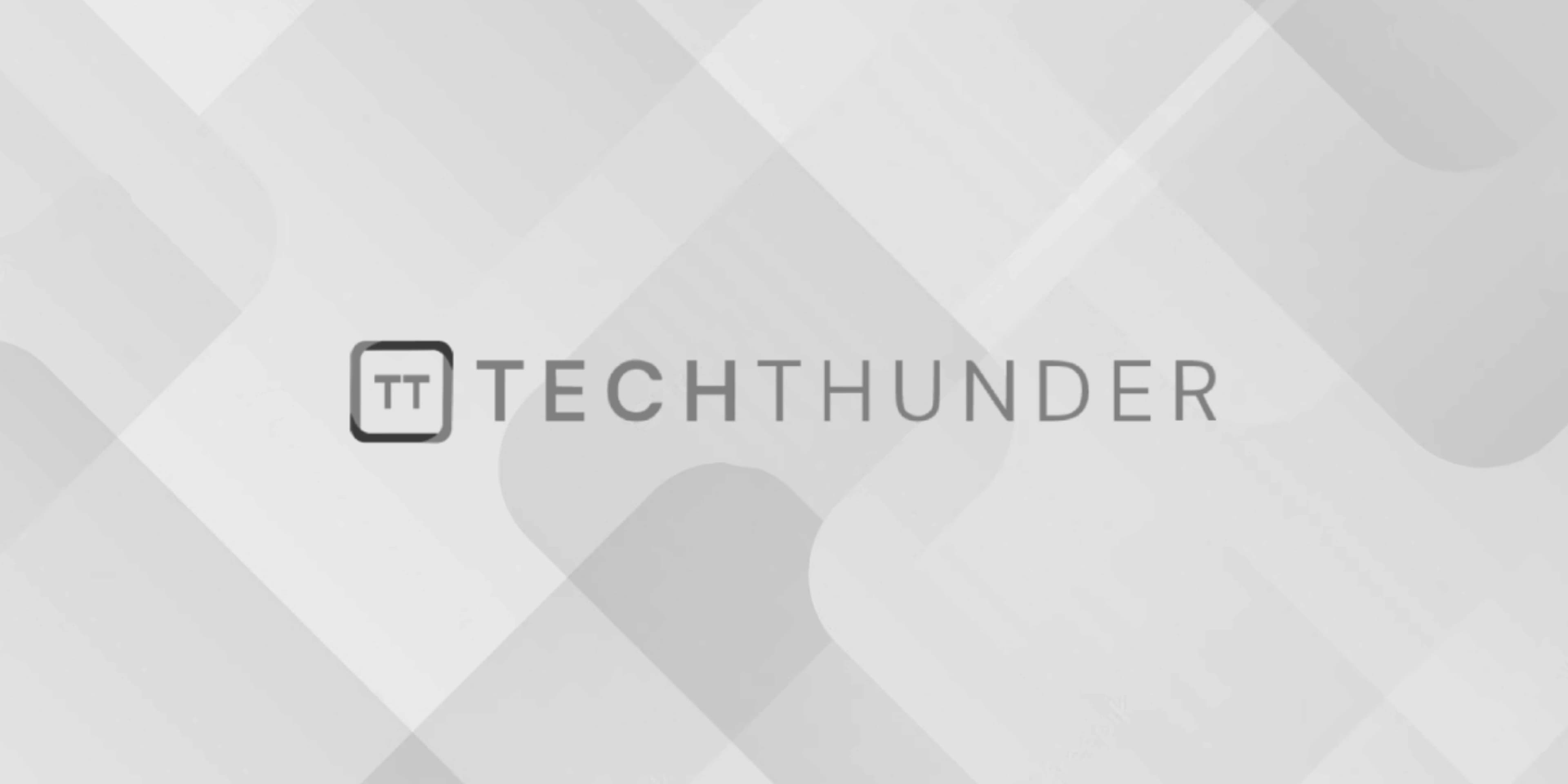
Why do we need Interfaces in PHP
Interfaces in PHP provide a way to define a contract for classes to follow. They serve as a blueprint or set of rules that classes must adhere to if they implement the interface. In other words, interfaces define a specific set of methods that participating classes must implement, but they do not provide the actual implementation of those methods. Instead, the classes that implement the interface must provide their own implementation for each method defined in the interface.
Here are some reasons why interfaces are important and beneficial in PHP:
- Encouraging Consistent Behavior: Interfaces enforce a consistent behavior among classes that implement them. By adhering to the interface, classes guarantee that they will have certain methods with specific names, arguments, and return types. This makes the code more reliable and easier to maintain, as developers can expect certain functionality from objects that implement a particular interface.
- Achieving Polymorphism: Interfaces enable polymorphism, which is the ability of different classes to be used interchangeably through a common interface. This allows for greater flexibility and code reuse. You can write functions or methods that accept objects of different classes, as long as they implement the same interface. This promotes code modularity and extensibility.
- Separation of Concerns: Interfaces help in separating the specification of behavior from the implementation details. By defining interfaces, you can focus on what needs to be done (the “what”) without worrying about how it is done (the “how”). This improves code organization and makes it easier to maintain and modify the codebase.
- Better Collaboration and Testing: Interfaces facilitate collaboration among developers. When designing a system, interface contracts provide clear communication about how classes should interact. This improves teamwork, as developers can work on their respective classes while adhering to the shared interface. Additionally, interfaces make it easier to write unit tests for individual components by allowing mock objects to be created for testing purposes.
- Loose Coupling: When a class depends on an interface rather than a concrete implementation, it reduces tight coupling between classes. This loose coupling makes the code more flexible and adaptable to changes, as it’s easier to switch out implementations that adhere to the same interface.
Example:
Let’s consider an example where we have an Animal
interface that defines a method makeSound()
. Then, two classes Dog
and Cat
implement this interface with their own specific sound implementations:
interface Animal {
public function makeSound();
}
class Dog implements Animal {
public function makeSound() {
echo "Woof! Woof!\n";
}
}
class Cat implements Animal {
public function makeSound() {
echo "Meow! Meow!\n";
}
}
function animalSounds(Animal $animal) {
$animal->makeSound();
}
$dog = new Dog();
$cat = new Cat();
animalSounds($dog); // Output: "Woof! Woof!"
animalSounds($cat); // Output: "Meow! Meow!"
In this example, both Dog
and Cat
implement the Animal
interface, which enforces the presence of the makeSound()
method. The animalSounds()
function can accept any object that implements the Animal
interface, allowing us to pass either a Dog
or a Cat
object to it, resulting in different behaviors based on the object’s implementation.
Overall, interfaces play a crucial role in defining a contract among classes, promoting code reusability, and enabling better collaboration in object-oriented programming.