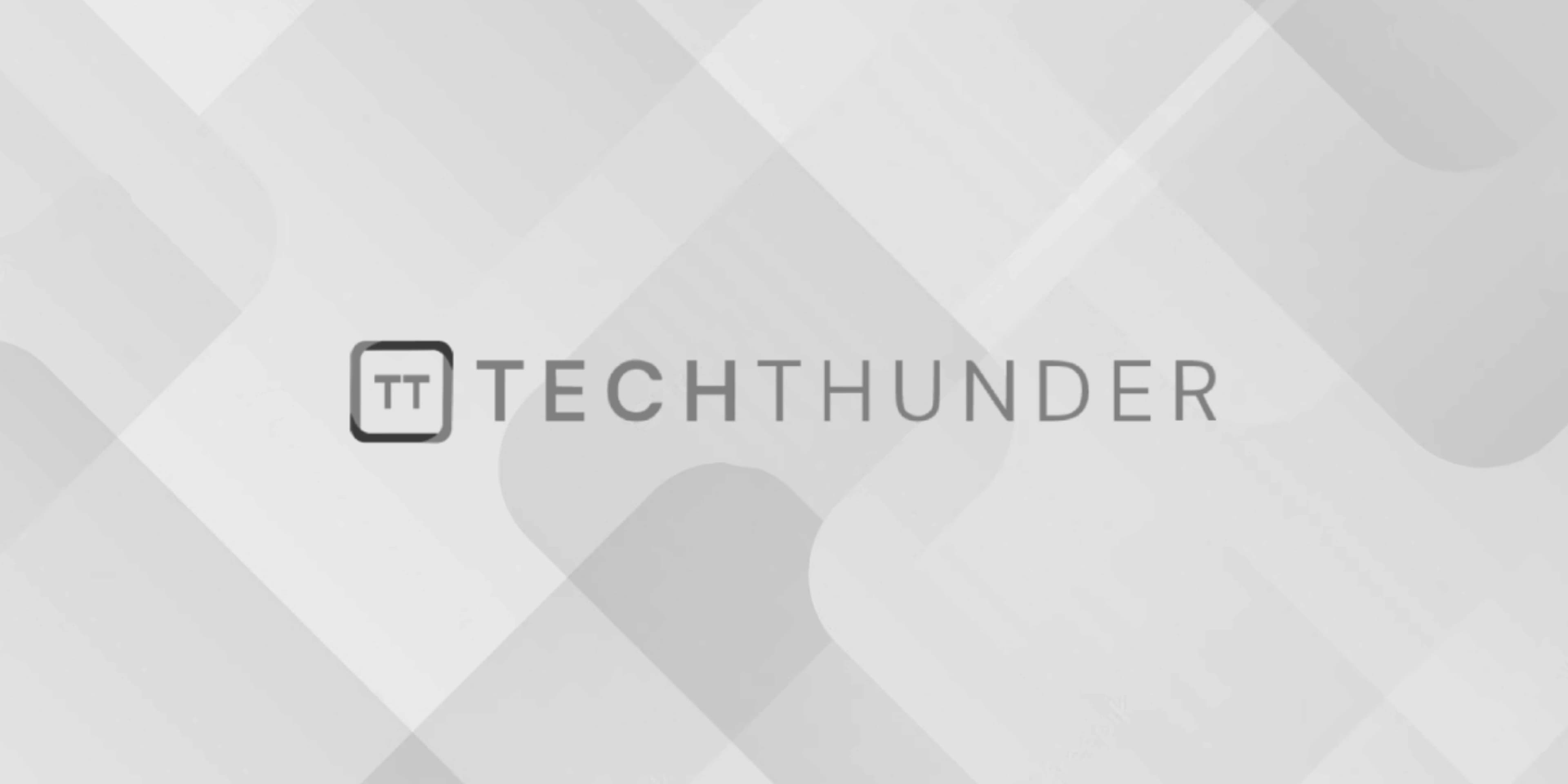
PHP MVC Architecture
PHP MVC (Model-View-Controller) is a software architectural pattern widely used in web development to structure and organize PHP applications. The MVC pattern separates an application into three main components, each responsible for specific tasks:
- Model:
- The Model represents the application’s data and business logic. It deals with data retrieval, manipulation, and validation. The Model interacts with the database or other data sources to fetch and store data. It does not contain any presentation or user interface-related code.
- View:
- The View is responsible for presenting the data to the user and displaying the user interface. It represents the HTML templates, CSS styles, and user interface elements. The View receives data from the Model and renders it in a format that users can interact with. The View does not contain any business logic.
- Controller:
- The Controller acts as an intermediary between the Model and the View. It receives user input and processes it, then interacts with the Model to update data or retrieve data based on the user’s actions. After processing the user input, the Controller selects the appropriate View to display the updated data or interface to the user.
The primary benefits of using the MVC architecture in PHP are:
- Separation of Concerns: The MVC pattern separates data manipulation, business logic, and presentation, making the codebase more organized and easier to maintain.
- Code Reusability: The separation of components allows for code reusability. For example, the same Model can be used in different Views or Controllers.
- Testability: By separating the application into distinct components, each part can be individually unit tested, leading to better code quality and easier debugging.
- Collaboration: The MVC pattern allows developers to work on different components independently, making team collaboration more efficient.
Here’s a basic example of how the MVC pattern is typically implemented in PHP:
Model (model.php):
class UserModel {
public function getUser($userId) {
// Fetch user data from the database and return it
}
public function updateUser($userId, $userData) {
// Update user data in the database
}
}
View (view.php):
class UserView {
public function showUserProfile($userData) {
// Display the user profile using HTML templates
}
}
Controller (controller.php):
class UserController {
private $model;
private $view;
public function __construct() {
$this->model = new UserModel();
$this->view = new UserView();
}
public function showProfile($userId) {
// Get user data from the Model
$userData = $this->model->getUser($userId);
// Display the user profile using the View
$this->view->showUserProfile($userData);
}
public function updateProfile($userId, $userData) {
// Update user data in the Model
$this->model->updateUser($userId, $userData);
// Redirect to the user's profile page
header("Location: profile.php?userId=$userId");
}
}
In this example, the UserModel
represents the data and business logic related to the user, while the UserView
is responsible for displaying the user profile. The UserController
acts as the intermediary, handling user requests, interacting with the Model, and rendering the appropriate View.
This is a simplified example, and in a real-world application, you would likely use a routing mechanism to map URLs to specific controllers and views, allowing for a more extensive and modular application structure. Implementing MVC helps to create scalable and maintainable PHP applications, making it a popular choice for web development projects.