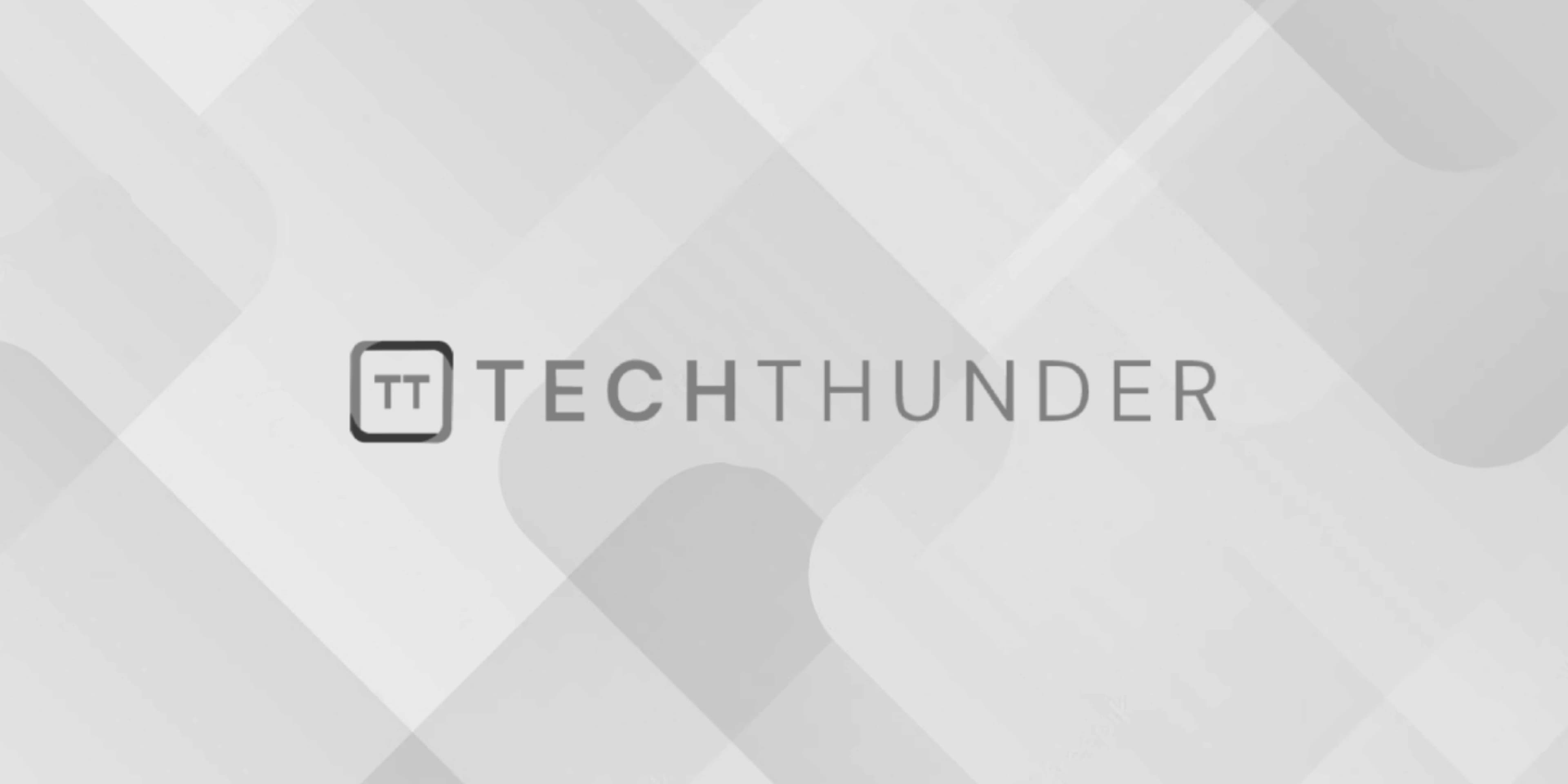
201 views
PHP Mail
In PHP, you can use the mail()
function to send email messages from your server to a specified recipient. The mail()
function sends a simple email, but it does not support attachments or complex email configurations. If you need more advanced email features, consider using third-party libraries like PHPMailer or Swift Mailer.
Here’s a basic example of how to use the mail()
function in PHP to send a simple email:
<?php
$to = "[email protected]";
$subject = "Test Email";
$message = "This is a test email sent from PHP's mail() function.";
$headers = "From: [email protected]\r\n";
$headers .= "Reply-To: [email protected]\r\n";
$headers .= "Content-type: text/plain; charset=utf-8\r\n";
// Send the email
if (mail($to, $subject, $message, $headers)) {
echo "Email sent successfully!";
} else {
echo "Failed to send email.";
}
?>
Explanation of the code:
$to
: The recipient’s email address.$subject
: The subject of the email.$message
: The content of the email message.$headers
: Additional headers for the email. In this example, we set the “From” and “Reply-To” headers and specify the content type as plain text with UTF-8 encoding.
Important Notes:
- The
mail()
function relies on the server’s configured mail system (e.g., Sendmail, Postfix) to send the email. Make sure that your server is properly configured to send emails. - The
mail()
function may not work on local development environments that lack a mail server setup. - Sending emails from localhost or local development environments might not always work as expected, especially if you don’t have a mail server configured.
- To improve the chances of successful email delivery, ensure that the “From” email address domain matches the server’s domain and that it is a valid email address with proper SPF and DKIM records set up.
As mentioned earlier, if you need more advanced features such as sending attachments, working with SMTP servers, or handling HTML emails, consider using a library like PHPMailer or Swift Mailer, which provide more robust and feature-rich email capabilities.