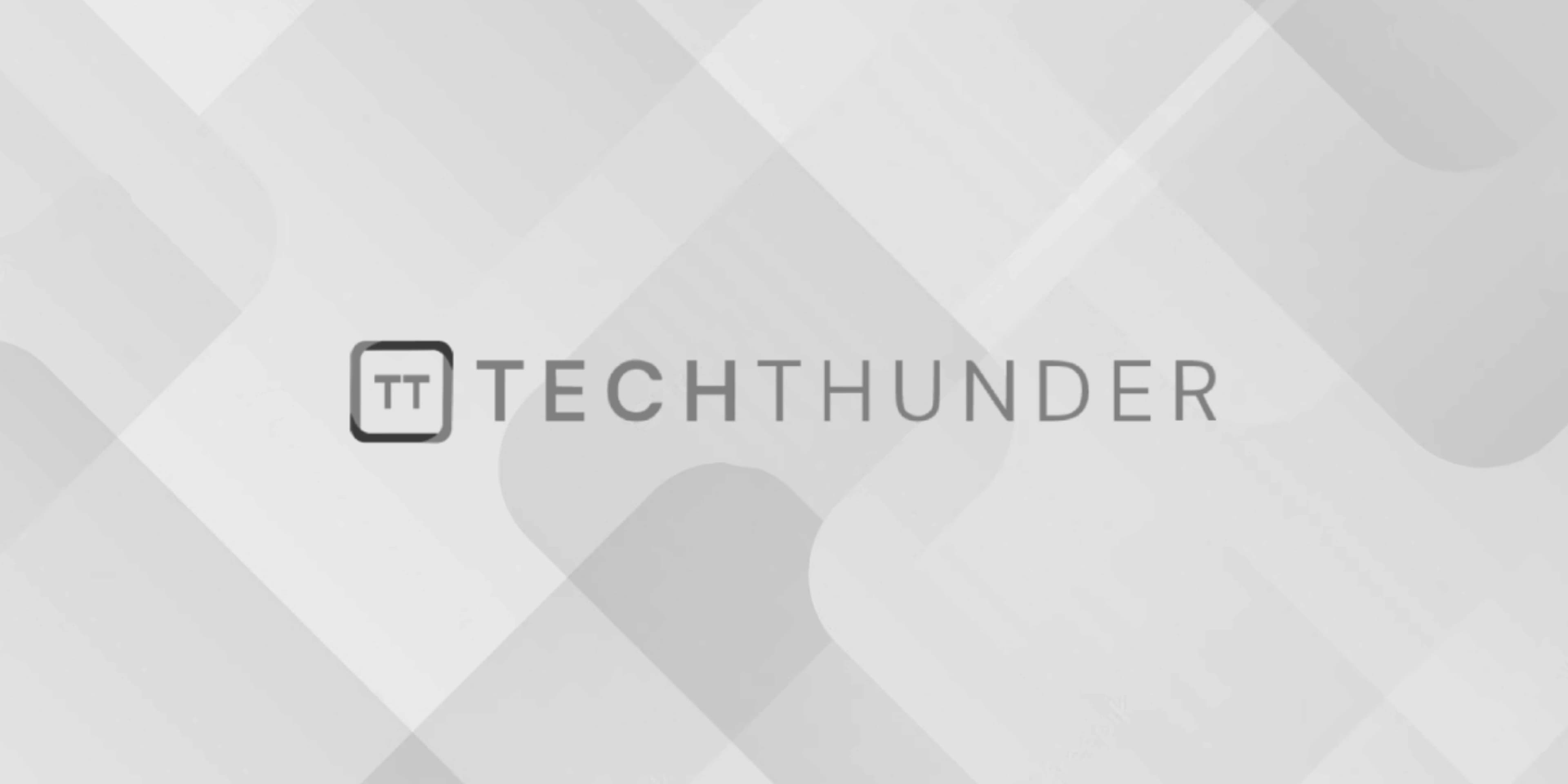
PHP GMP gmp_testbit() Function
In PHP, the gmp_testbit()
function is used to test the value of a specific bit at a given position in a GMP (GNU Multiple Precision) number. GMP is a PHP extension that allows you to perform arbitrary precision arithmetic on large integers, overcoming the limitations of native PHP integers.
The gmp_testbit()
function takes two arguments:
num
: The GMP number to test.index
: The position of the bit to test. The index is 0-based, meaning the rightmost bit has index 0, the bit to its left has index 1, and so on.
Here’s the syntax of the gmp_testbit()
function:
gmp_testbit ( GMP $num , int $index ) : bool
Parameters:
$num
: The GMP number to test.$index
: The position of the bit to test.
Return Value:
- The function returns a boolean value (
true
orfalse
) indicating whether the specified bit is set (true
) or not set (false
).
Example:
<?php
// Example usage of gmp_testbit()
$gmpNum = gmp_init("5"); // Binary representation of 5: 101
// Test the value of bit at position 0 (rightmost bit)
$isBitSet = gmp_testbit($gmpNum, 0);
echo "Is Bit Set at Position 0? " . ($isBitSet ? 'Yes' : 'No'); // Output: Is Bit Set at Position 0? Yes
// Test the value of bit at position 1
$isBitSet = gmp_testbit($gmpNum, 1);
echo "Is Bit Set at Position 1? " . ($isBitSet ? 'Yes' : 'No'); // Output: Is Bit Set at Position 1? No
?>
In this example, we use the gmp_testbit()
function to test the value of the bit at different positions in the GMP number 5 (binary representation: 101).
- The first test checks if the bit at position 0 (rightmost bit) is set, which is true (1).
- The second test checks if the bit at position 1 is set, which is false (0).
The function returns true
if the specified bit is set (1) and false
if the bit is not set (0).
Remember that GMP functions work with large integers and provide the ability to perform arithmetic operations with arbitrary precision. It is particularly useful when dealing with numbers that exceed the size limits of native PHP integers.