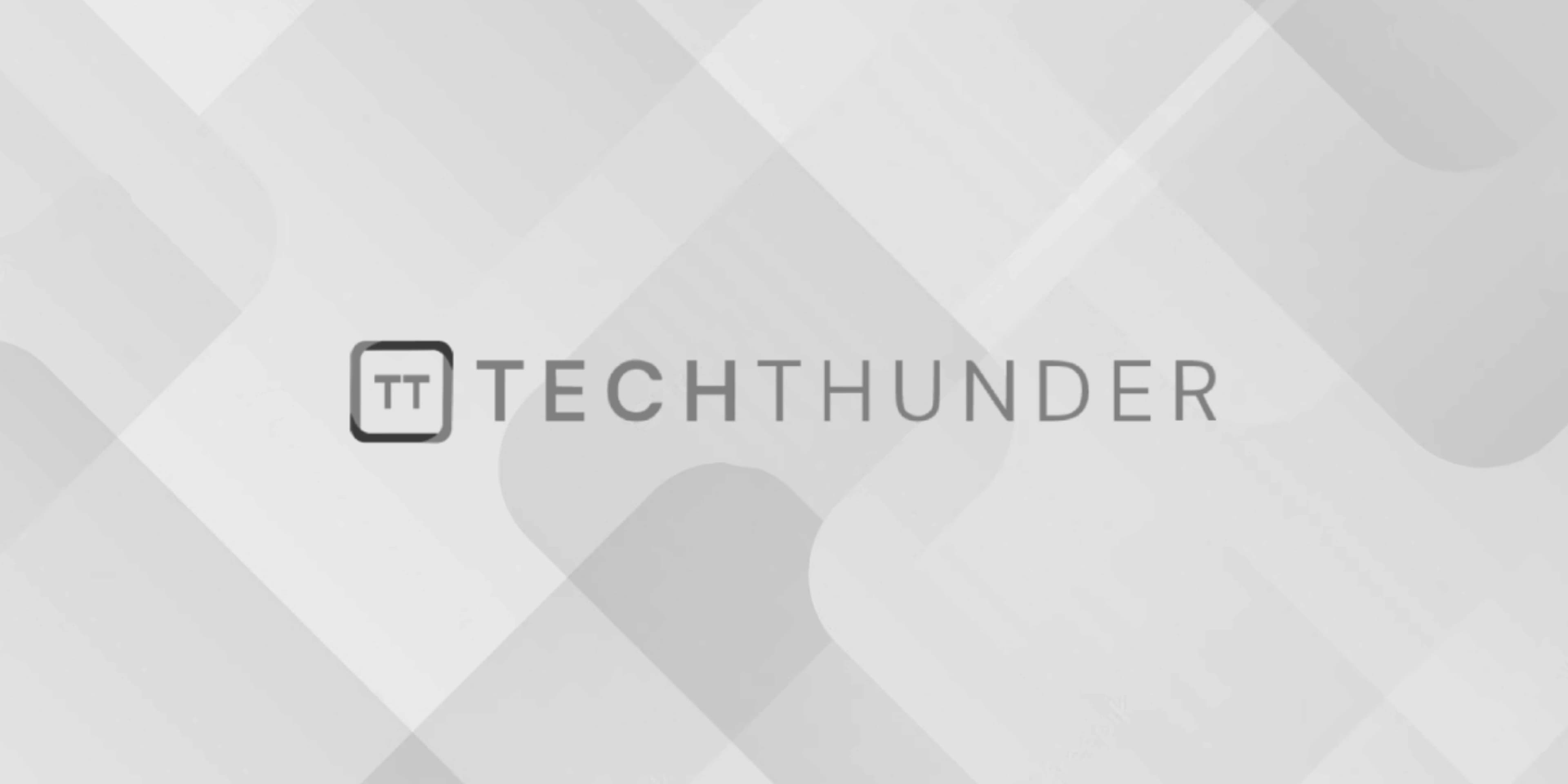
PHP unset() function
In PHP, unset()
is a built-in function used to unset or destroy a specified variable or array element. When you unset a variable or array element, it frees up the memory associated with that variable, making it no longer accessible in the current scope.
Syntax:
The syntax for the unset()
function is as follows:
unset($variable);
unset($array[index]);
Parameters:
$variable
: The variable to unset. It can be any valid PHP variable, such as a simple variable, an array, or an object.$array
: The array from which you want to unset a specific element.index
: The index of the element you want to unset within the array.
Return Value:
The unset()
function does not return any value. It directly modifies the variable or array, removing the specified element.
Examples:
- Unsetting a Variable:
$var = "Hello";
unset($var);
// $var is now unset and no longer accessible
- Unsetting an Array Element:
$fruits = array("apple", "banana", "orange");
unset($fruits[1]);
// The "banana" element is removed from the $fruits array
- Unsetting an Array Variable:
$fruits = array("apple", "banana", "orange");
unset($fruits);
// The entire $fruits array is unset
It’s important to note that unsetting an array element using unset()
leaves a gap in the array’s index. For example, if you unset the element at index 1 in the $fruits
array in the second example, the array will become:
Array
(
[0] => apple
[2] => orange
)
To reindex the array after unsetting an element, you can use the array_values()
function:
$fruits = array_values($fruits);
In summary, unset()
is a useful function for freeing up memory by removing variables or elements from arrays that are no longer needed in your PHP code. However, be cautious when using unset()
to avoid unintentionally removing data that is still needed elsewhere in your script.