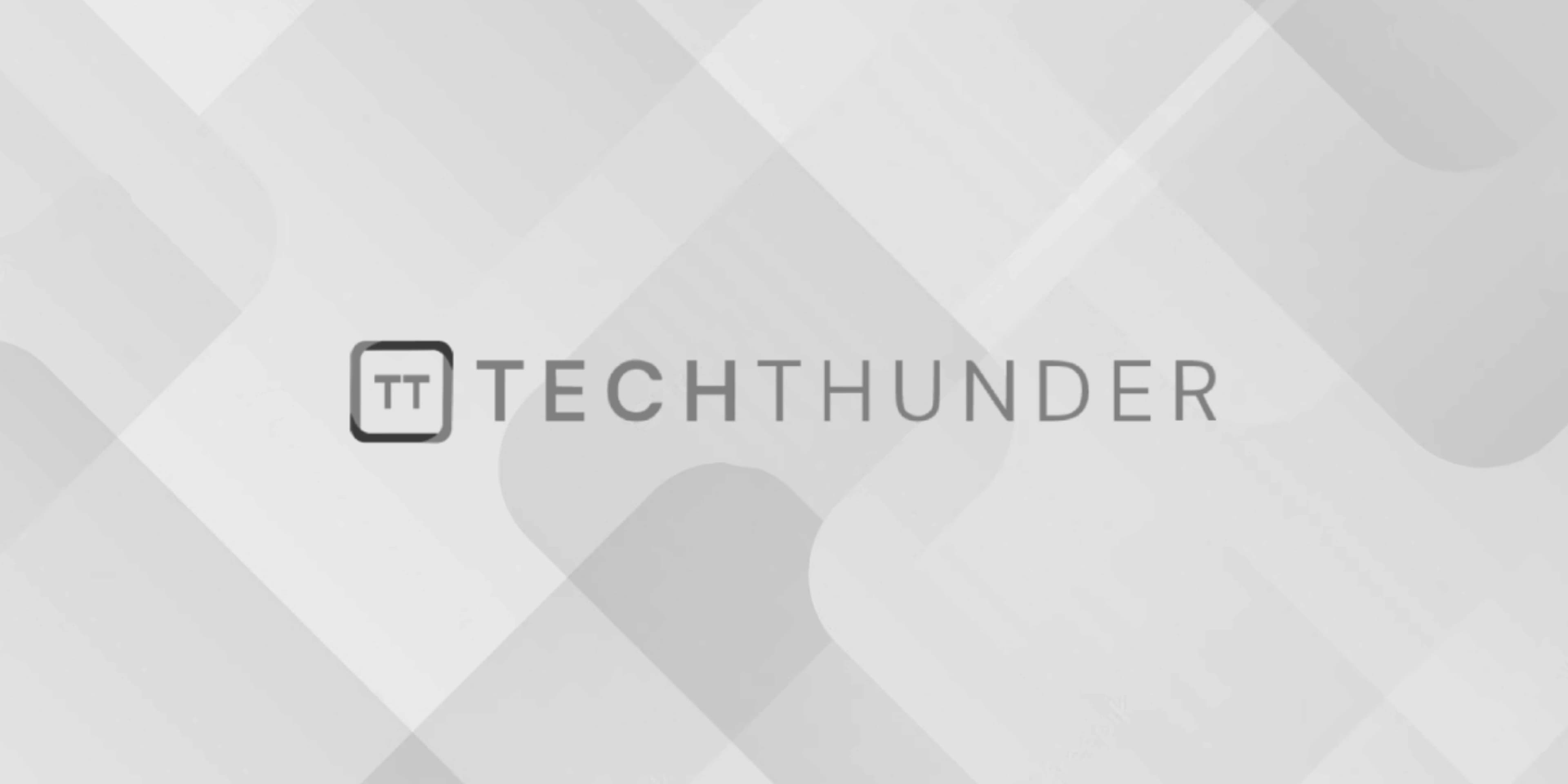
How to Use PHP Serialize() and Unserialize() Function
The serialize()
and unserialize()
functions in PHP are used to convert PHP data into a storable string representation (serialization) and to restore the original data from the serialized string (unserialization). This is particularly useful when you need to store data in files, databases, or pass data between different applications in a platform-independent format.
serialize()
Function:
The serialize()
function takes a PHP variable (e.g., array, object, or simple data type) and converts it into a string representation that can be stored or transmitted. The resulting string is a serialized form of the original data, which can later be converted back to its original form using the unserialize()
function.
Here’s the syntax of the serialize()
function:
string serialize(mixed $data)
Example:
$data = array('name' => 'John', 'age' => 30, 'email' => '[email protected]');
// Serialize the array into a string
$serializedData = serialize($data);
// Display the serialized data
echo $serializedData;
Output (Serialized String):
a:3:{s:4:"name";s:4:"John";s:3:"age";i:30;s:5:"email";s:17:"[email protected]";}
unserialize()
Function:
The unserialize()
function takes a serialized string and converts it back into its original PHP data format. It reverses the process performed by the serialize()
function.
Here’s the syntax of the unserialize()
function:
mixed unserialize(string $data)
Example:
$serializedData = 'a:3:{s:4:"name";s:4:"John";s:3:"age";i:30;s:5:"email";s:17:"[email protected]";}';
// Unserialize the string back into an array
$originalData = unserialize($serializedData);
// Display the original data
print_r($originalData);
Output (Original Array):
Array
(
[name] => John
[age] => 30
[email] => [email protected]
)
In this example, we first use the serialize()
function to convert an array into a serialized string. Then, we use the unserialize()
function to restore the original array from the serialized string.
It’s important to note that not all PHP data types can be successfully serialized and unserialized. For example, resources and objects that have non-serializable properties cannot be serialized. Additionally, when passing serialized data between different applications or environments, ensure that they have the same PHP configuration and versions to avoid compatibility issues.