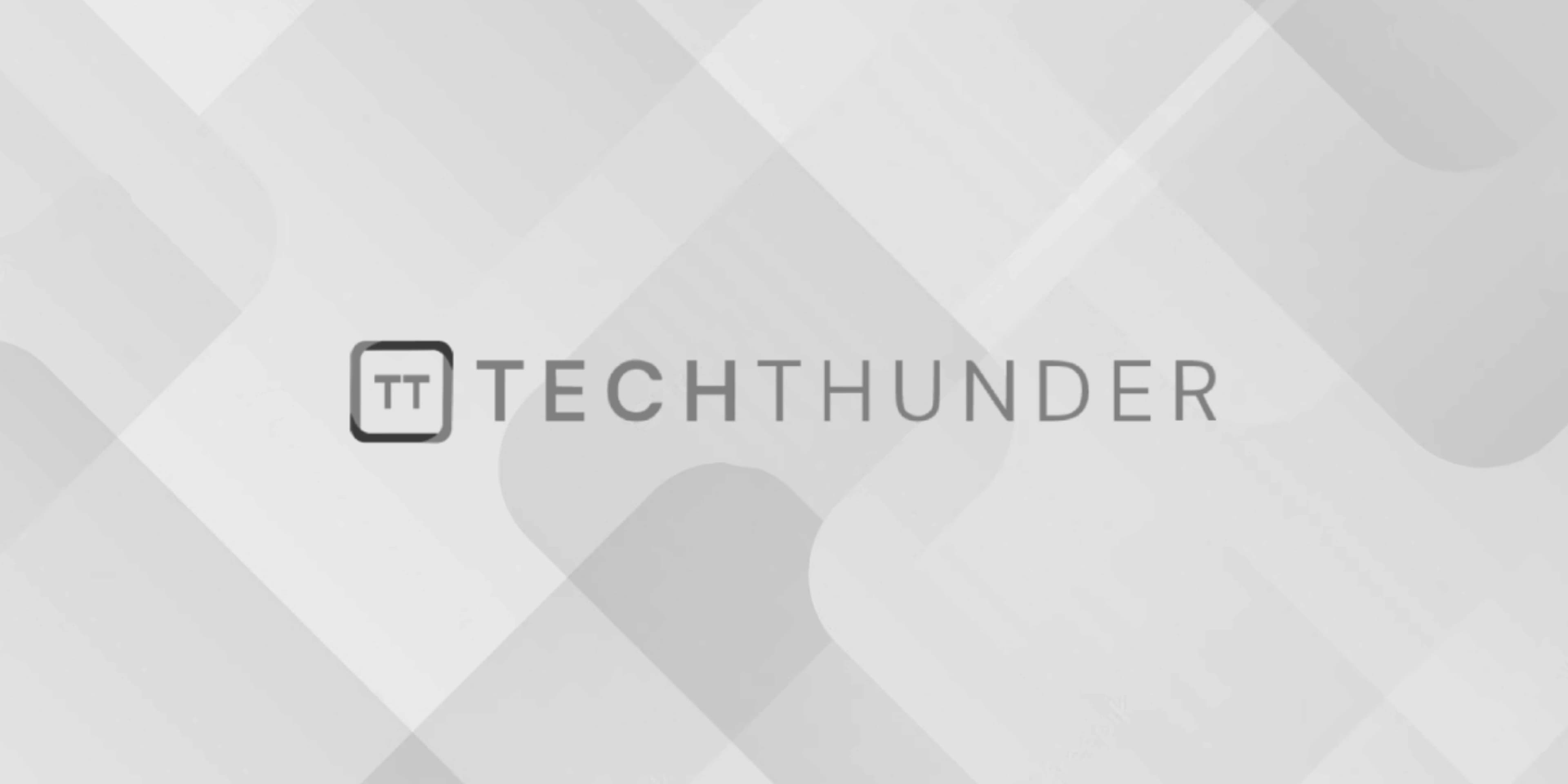
240 views
PHP Laravel 5.6 Rest API with Passport
To create a RESTful API with Laravel 5.6 and implement authentication using Laravel Passport, follow these steps:
- Install Laravel and Set Up Your Project:
Install Laravel 5.6 using Composer if you haven’t done so already:
composer create-project --prefer-dist laravel/laravel project-name "5.6.*"
- Set Up Database:
Configure your.env
file with the necessary database credentials to connect to your database. - Install Laravel Passport:
Run the following command to install Laravel Passport:
composer require laravel/passport
- Set Up Passport:
After installing Passport, run the following command to create the necessary database tables for Passport:
php artisan migrate
Next, run the passport:install
command to create the encryption keys required for token generation:
php artisan passport:install
- Configure Passport in AuthServiceProvider:
In theAuthServiceProvider
class (located atapp/Providers/AuthServiceProvider.php
), add the following line in theboot()
method to enable Passport routes and functionality:
use Laravel\Passport\Passport;
public function boot()
{
$this->registerPolicies();
Passport::routes();
}
- Create User Model:
Create aUser
model or use the existing one. Make sure the model uses theHasApiTokens
trait, which comes with Laravel Passport:
use Laravel\Passport\HasApiTokens;
use Illuminate\Foundation\Auth\User as Authenticatable;
class User extends Authenticatable
{
use HasApiTokens;
// Rest of your user model code
}
- Set Up API Routes:
In theroutes/api.php
file, define your API routes and their corresponding controllers. For example:
Route::group(['middleware' => 'auth:api'], function () {
// Your protected API routes here
});
- Create AuthController for Login and Register:
Create anAuthController
to handle user registration and login. You can use Laravel’s built-in authentication scaffolding or create custom controller methods. - Protect API Routes:
Use theauth:api
middleware on routes that require authentication. This middleware will validate the access tokens sent with the API requests. - Testing the API:
Use tools like Postman to test your API endpoints. To obtain an access token, make aPOST
request to/oauth/token
with the client credentials and user credentials. The response will include the access token, which you can use to access the protected routes.
That’s it! Now you have a RESTful API built with Laravel 5.6 and secured with Laravel Passport for authentication using OAuth2. You can now access your API endpoints by sending the appropriate access tokens with your requests.