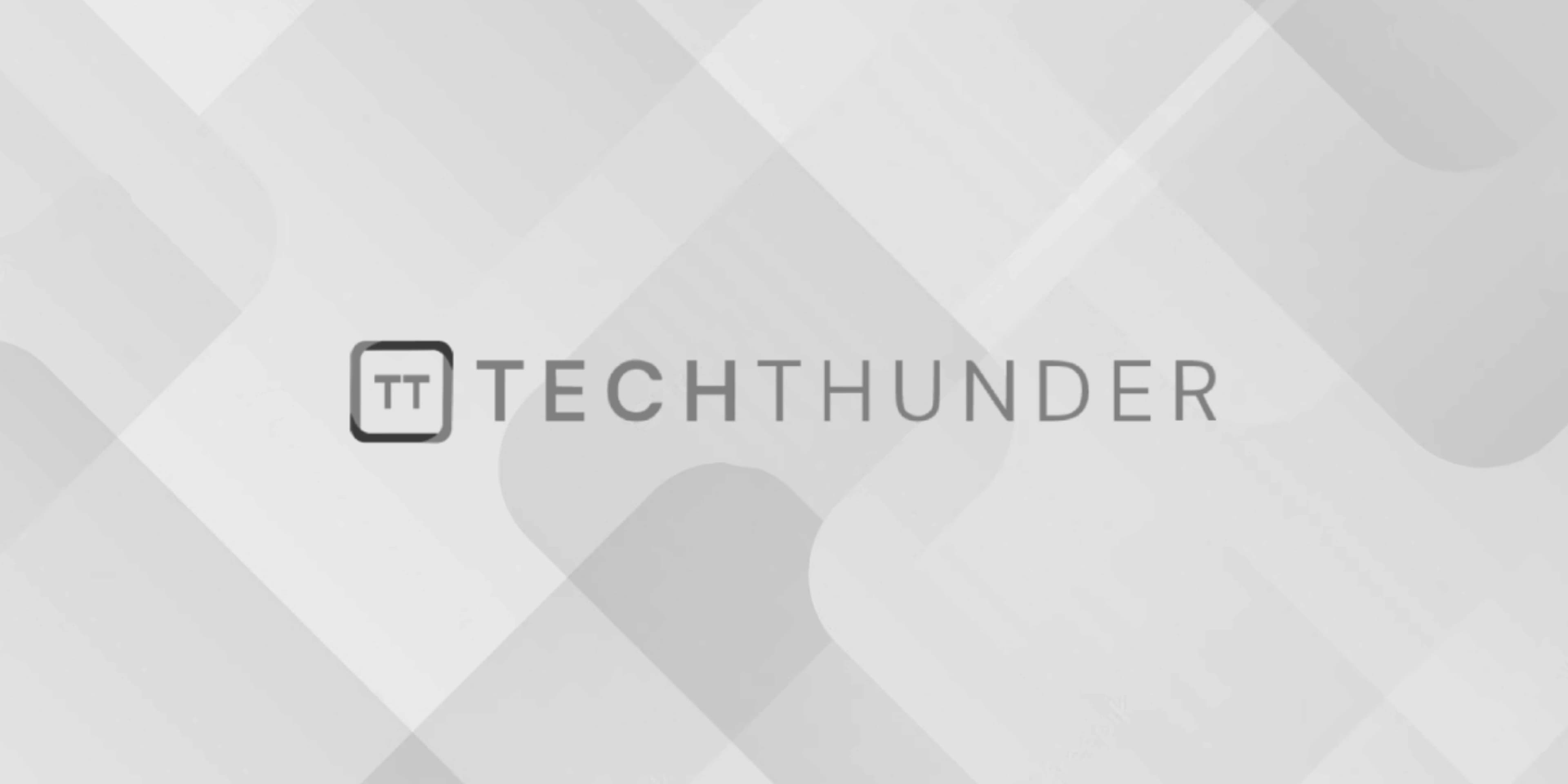
PHP alert
In PHP, there is no built-in alert()
function like in JavaScript. PHP is a server-side programming language, and it does not directly interact with the client-side (browser) like JavaScript does.
To display a message or alert to the user in PHP, you typically need to use HTML or JavaScript. Here are two common ways to achieve this:
- Using HTML and Echo/Print:
You can use PHP’secho
orprint
statement to output HTML content, including a message that will be displayed to the user when the PHP script is executed.
<?php
$message = "Hello, this is an alert in PHP!";
echo "<script>alert('$message');</script>";
?>
The echo
statement here outputs a JavaScript <script>
tag with an alert()
function that will display the message in an alert box when the PHP script is executed.
- Using JavaScript in an HTML Page:
Another approach is to use PHP to generate JavaScript code directly in an HTML page. You can place the PHP script in a<script>
block or an external JavaScript file.
<!DOCTYPE html>
<html>
<head>
<title>PHP Alert</title>
</head>
<body>
<!-- PHP script to generate JavaScript alert -->
<?php
$message = "Hello, this is an alert in PHP!";
?>
<script>
alert('<?php echo $message; ?>');
</script>
</body>
</html>
In this example, the PHP variable $message
contains the message you want to display, and PHP generates the JavaScript code within the <script>
block. The generated JavaScript code uses the alert()
function to show the message when the HTML page is loaded.
Remember that PHP runs on the server, and the output of PHP (such as HTML, JavaScript, or CSS) is sent to the client-side (browser) for rendering. So, any user interaction (e.g., showing alerts) typically involves JavaScript, which is a client-side scripting language.