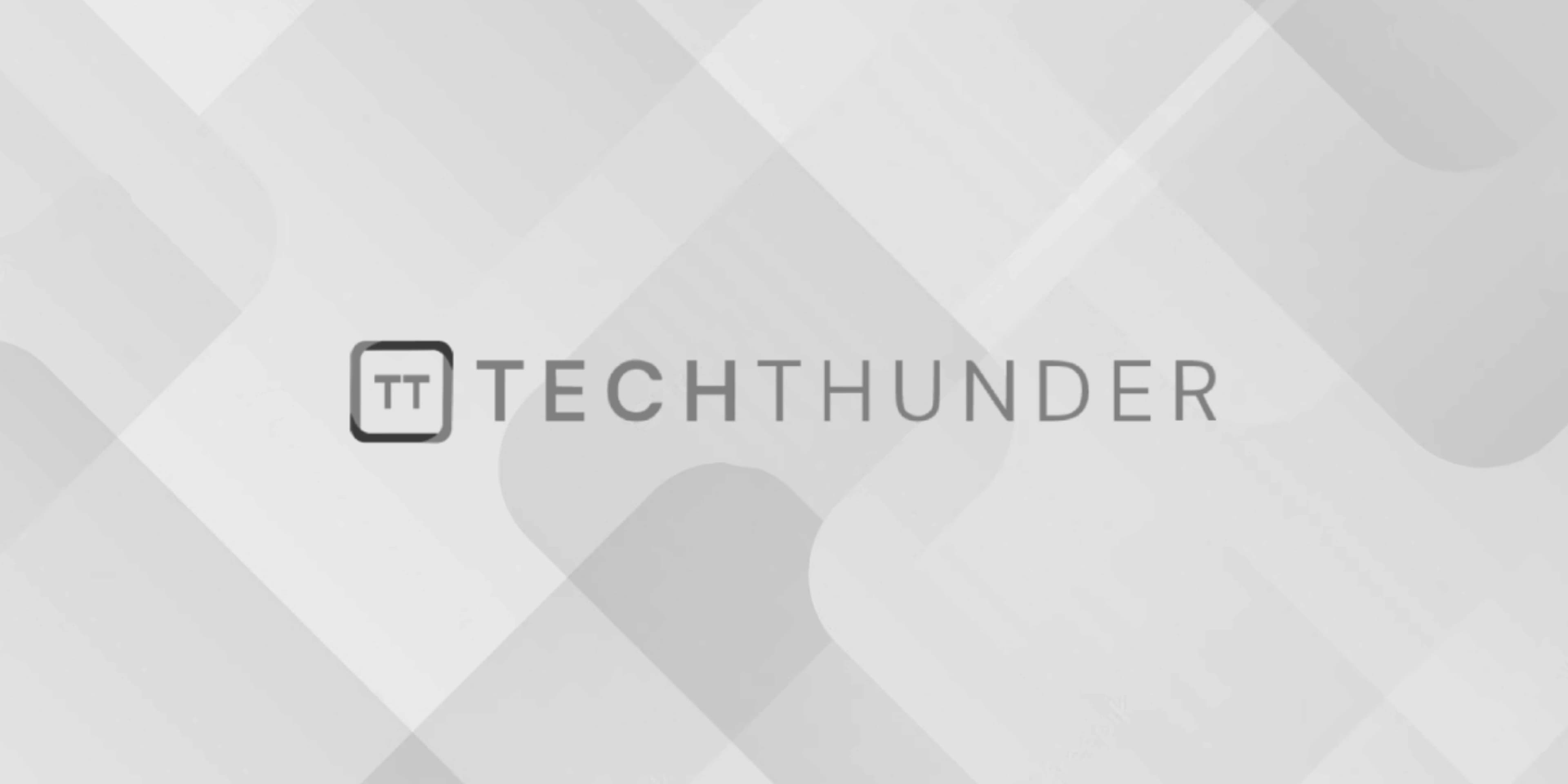
PHP empty() Function
In PHP, the empty()
function is a language construct used to determine if a variable is considered to be empty. It checks whether the variable has a non-empty value or evaluates to false. The function returns true
if the variable is empty and false
if it contains a value.
The empty()
function is particularly useful for checking whether a variable exists and has a non-empty value before using it in the code. It helps prevent errors caused by using uninitialized or undefined variables.
The syntax of the empty()
function is as follows:
empty($variable);
Parameters:
$variable
: The variable to be checked for emptiness.
Return value:
- Returns
true
if the variable is empty (i.e., it does not exist, is null, false, 0, an empty string, or an array with no elements). - Returns
false
if the variable contains a value that is considered non-empty.
Examples:
- Checking if a variable is empty:
$name = ""; // An empty string
$age = 0; // 0 (integer)
if (empty($name)) {
echo "Name is empty.\n";
}
if (empty($age)) {
echo "Age is empty.\n";
}
Output:
Name is empty.
Age is empty.
- Using
empty()
to check if an array is empty:
$fruits = array(); // An empty array
if (empty($fruits)) {
echo "The fruits array is empty.\n";
}
Output:
The fruits array is empty.
- Using
empty()
to validate user input:
// Assuming $_POST['username'] contains user input from a form submission
if (empty($_POST['username'])) {
echo "Please enter a username.\n";
} else {
echo "Welcome, " . $_POST['username'] . "!\n";
}
In this example, if the user does not provide a username in the form, the empty()
function is used to detect that and prompt the user to enter a username.
Keep in mind that the empty()
function treats variables with a value of 0
or "0"
as empty, which might not be the desired behavior in some cases. If you want to check if a variable is not only empty but also not equal to zero, use the isset()
function in conjunction with other appropriate checks.