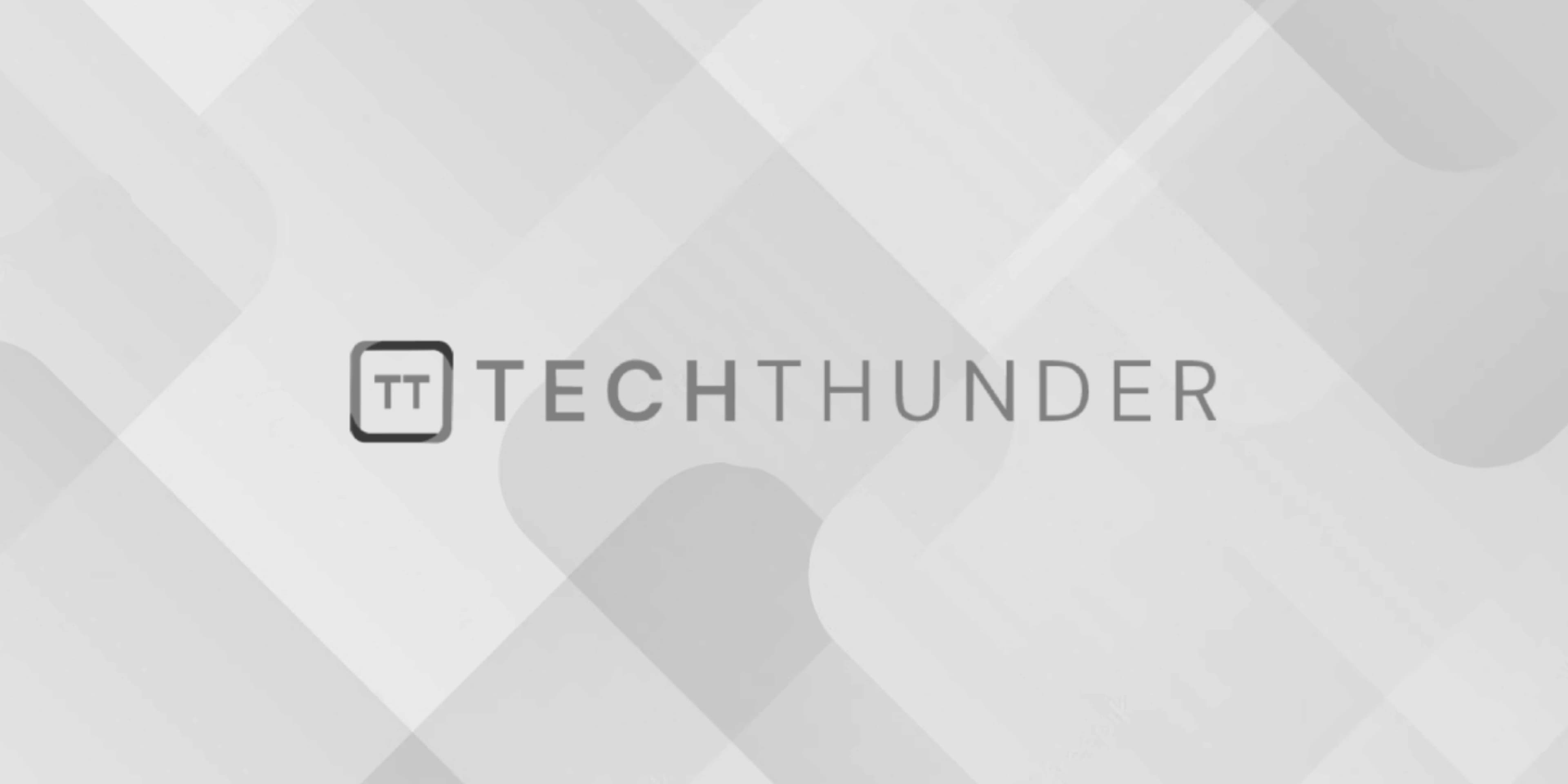
179 views
How to make a website in PHP
To create a website using PHP, you’ll need to follow these steps:
- Plan Your Website:
Before starting the development process, it’s essential to plan your website. Define the purpose, target audience, features, and layout of your site. Decide on the pages you want to include, the content, and any interactive elements. - Set Up the Development Environment:
To create a PHP website, you’ll need a local development environment. You can use XAMPP, WAMP, or MAMP, depending on your operating system. These packages include Apache, MySQL, and PHP, providing you with a complete development environment. - Create a Folder Structure:
Create a folder for your project and organize it into subdirectories. Common directories include “css” for stylesheets, “js” for JavaScript files, “images” for images, and “includes” for reusable PHP files. - Create the Home Page (index.php):
Start by creating the home page (index.php). This will be the main page of your website.
<!DOCTYPE html>
<html>
<head>
<title>Your Website Title</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<header>
<!-- Your website header content -->
</header>
<nav>
<!-- Your website navigation menu -->
</nav>
<main>
<!-- Your website main content -->
</main>
<footer>
<!-- Your website footer content -->
</footer>
<script src="js/script.js"></script>
</body>
</html>
- Create Other Pages:
Create additional PHP files for other pages on your website. For example, about.php, contact.php, services.php, etc. - Use PHP for Dynamic Content:
PHP allows you to create dynamic content by embedding PHP code within your HTML files. You can use PHP to fetch data from a database, display dynamic content, handle forms, and much more.
For example, to display dynamic content on the homepage:
<main>
<h1>Welcome to our website, <?php echo $username; ?></h1>
<p><?php echo $description; ?></p>
</main>
- Set Up a Database (Optional):
If your website requires a database for storing data, set up a database using tools like phpMyAdmin or MySQL Workbench. - Connect PHP to the Database (Optional):
In PHP, use themysqli
orPDO
extension to connect your website to the database and perform database operations. - Style Your Website:
Use CSS to style your website and make it visually appealing. - Test Your Website:
Test your website thoroughly to ensure everything works as expected. Check for bugs, broken links, and cross-browser compatibility. - Upload Your Website:
Once you are satisfied with your website, upload the files to your web server using FTP or cPanel file manager.
Remember to always keep security in mind, especially if your website handles user data or has any form of user interaction. Use secure coding practices and sanitize user input to prevent security vulnerabilities.
Creating a complete website is a significant project, and this overview provides a starting point. Depending on your specific requirements and the complexity of your website, you may need to dive deeper into specific aspects of PHP development, such as working with databases, using PHP frameworks (e.g., CodeIgniter, Laravel), and implementing advanced features.